Modify the following program so it displays a menu allowing the user to select an addition, subtraction, multiplication, or division problem. The final selection on the menu should let the user quit the program. After the user has finished the math problem, the program should display the menu again. This process is repeated until the user chooses to quit the program. Input Validation: If the user selects an item not on the menu, display an error message and display the menu again.
Operations
In mathematics and computer science, an operation is an event that is carried out to satisfy a given task. Basic operations of a computer system are input, processing, output, storage, and control.
Basic Operators
An operator is a symbol that indicates an operation to be performed. We are familiar with operators in mathematics; operators used in computer programming are—in many ways—similar to mathematical operators.
Division Operator
We all learnt about division—and the division operator—in school. You probably know of both these symbols as representing division:
Modulus Operator
Modulus can be represented either as (mod or modulo) in computing operation. Modulus comes under arithmetic operations. Any number or variable which produces absolute value is modulus functionality. Magnitude of any function is totally changed by modulo operator as it changes even negative value to positive.
Operators
In the realm of programming, operators refer to the symbols that perform some function. They are tasked with instructing the compiler on the type of action that needs to be performed on the values passed as operands. Operators can be used in mathematical formulas and equations. In programming languages like Python, C, and Java, a variety of operators are defined.
C++ Visual Studio
Modify the following
Input Validation: If the user selects an item not on the menu, display an error message and display the menu again.
Code:
#include <iostream>
#include <cstdlib>
#include <ctime>
#include <conio.h>
using namespace std;
int main() {
srand(static_cast<unsigned int>(time(nullptr)));
while (true) {
// Generate two random numbers.
int num1 = rand() % 1000;
int num2 = rand() % 1000;
// Display the problem.
cout << "Solve the following math problem:" << endl;
cout << num1 << " + " << num2 << endl;
// Wait for user input to reveal the answer.
cout << "Press any key to reveal the answer...";
_getch(); // Wait for a key press
cout << endl;
// Display the correct solution.
int sum = num1 + num2;
cout << num1 << " + " << num2 << " = " << sum << endl;
// Ask if user wants to continue.
cout << "Do you want to try another problem? (y/n): ";
char choice = _getch();
cout << endl;
if (choice != 'y' && choice != 'Y') {
break; // Exit the loop if the user doesn't want to continue
}
// Clear the screen for the next problem
system("cls");
}
cout << "You may exit the program." << endl;
return 0;
}
Thank you!

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

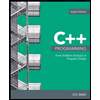
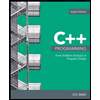