C language The program should allow users to add, subtract, multiply, and divide fractions. The program should display a menu and prompt the user for a mathematical operation, until they enter 5 to exit. The menu should give the user the option to add, subtract, multiply or divide fractions, or exit. After the user chooses their option, they should be prompted to input two fractions. The result of the arithmetic should then be displayed to the screen. Requirements main() Functionality: This main function should prompt the user for a menu option until they enter 5. If the user doesn't choose Option 5 to end the program, it should get two fractions from the user. It should decide, based on the menu option which actions to perform on the fractions. Option 1 adds the two fractions together. Option 2 subtracts the first fraction from the second. Option 3 multiplies the two fractions together. Option 4 divides the first fraction by the second one. In addition to the main functions, your program should have 6 more functions: getMenuChoice() Input Parameters: none Returned Output: int Functionality: This function should display to the screen the options available to the user and return the users inputted menu choice. getFraction() Input Parameters: int pointer, int pointer Returned Output: none Functionality: This function should prompt the user to enter a fraction, using the forward slash between the two values. The numerator should be stored into the variable that one of the pointer parameters points to, and the denominator should be stored into the variable that the other pointer parameter points to. addFraction() Input Parameters: int, int, int, int, int pointer, int pointer Returned Output: none Functionality: This function adds two fractions, each represented by a pair of integer input parameters. The resulting fraction should be stored in the variables that the last pair of pointer parameters points to. subtractFraction() Input Parameters: int, int, int, int, int pointer, int pointer Returned Output: none Functionality: This function subtracts two fractions, each represented by a pair of integer input parameters. The resulting fraction should be stored in the variables that the last pair of pointer parameters points to. multiplyFraction() Input Parameters: int, int, int, int, int pointer, int pointer Returned Output: none Functionality: This function multiplies two fractions, each represented by a pair of integer input parameters. The resulting fraction should be stored in the variables that the last pair of pointer parameters points to. dividesFraction() Input Parameters: int, int, int, int, int pointer, int pointer Returned Output: none Functionality: This function divides two fractions, each represented by a pair of integer input parameters. The resulting fraction should be stored in the variables that the last pair of pointer parameters points to.
Max Function
Statistical function is of many categories. One of them is a MAX function. The MAX function returns the largest value from the list of arguments passed to it. MAX function always ignores the empty cells when performing the calculation.
Power Function
A power function is a type of single-term function. Its definition states that it is a variable containing a base value raised to a constant value acting as an exponent. This variable may also have a coefficient. For instance, the area of a circle can be given as:
C language
The program should allow users to add, subtract, multiply, and divide fractions.
The program should display a menu and prompt the user for a mathematical operation, until they enter 5 to exit. The menu should give the user the option to add, subtract, multiply or divide fractions, or exit. After the user chooses their option, they should be prompted to input two fractions. The result of the arithmetic should then be displayed to the screen.
Requirements
main()
Functionality: This main function should prompt the user for a menu option until they enter 5. If the user doesn't choose Option 5 to end the program, it should get two fractions from the user. It should decide, based on the menu option which actions to perform on the fractions. Option 1 adds the two fractions together. Option 2 subtracts the first fraction from the second. Option 3 multiplies the two fractions together. Option 4 divides the first fraction by the second one.
In addition to the main functions, your program should have 6 more functions:
getMenuChoice()
Input Parameters: none
Returned Output: int
Functionality: This function should display to the screen the options available to the user and return the users inputted menu choice.
getFraction()
Input Parameters: int pointer, int pointer
Returned Output: none
Functionality: This function should prompt the user to enter a fraction, using the forward slash between the two values. The numerator should be stored into the variable that one of the pointer parameters points to, and the denominator should be stored into the variable that the other pointer parameter points to.
addFraction()
Input Parameters: int, int, int, int, int pointer, int pointer
Returned Output: none
Functionality: This function adds two fractions, each represented by a pair of integer input parameters. The resulting fraction should be stored in the variables that the last pair of pointer parameters points to.
subtractFraction()
Input Parameters: int, int, int, int, int pointer, int pointer
Returned Output: none
Functionality: This function subtracts two fractions, each represented by a pair of integer input parameters. The resulting fraction should be stored in the variables that the last pair of pointer parameters points to.
multiplyFraction()
Input Parameters: int, int, int, int, int pointer, int pointer
Returned Output: none
Functionality: This function multiplies two fractions, each represented by a pair of integer input parameters. The resulting fraction should be stored in the variables that the last pair of pointer parameters points to.
dividesFraction()
Input Parameters: int, int, int, int, int pointer, int pointer
Returned Output: none
Functionality: This function divides two fractions, each represented by a pair of integer input parameters. The resulting fraction should be stored in the variables that the last pair of pointer parameters points to.


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

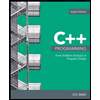
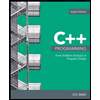