Java Program Write the FullName class to be a subclass of Name, so that it also stores a middle name. It needs a constructor and a toString() method. Make sure: FullName class definition as described above Instantiating a FullName object from the new mayor's name entered by the user, and adding that object to the mayorList Inputting the names from mayors.txt Instantiating a FullName object for each of those names from the file and adding them to the mayorList Using exception handling to catch errors that might occur in file i/o: your program should catch all exceptions. Your main method should not have a "throws" clause. Other methods may throw exceptions, as long as the main method catches them. Code: import java.io.*; import java.util.*; class Main { public static void main(String[] args) { Scanner inFile = null, keyboard; String first, middle, last; ArrayList mayorList = new ArrayList(); keyboard = new Scanner(System.in); System.out.println("We have a new mayor:"); System.out.println("Please enter first name"); first = keyboard.nextLine(); System.out.println("Please enter middle name"); middle = keyboard.nextLine(); System.out.println("Please enter last name"); last = keyboard.nextLine(); // DO NOT CHANGE ANY OF THE ABOVE CODE. // ADD YOUR CODE HERE. // DO NOT CHANGE ANY OF THE BELOW CODE. Collections.sort(mayorList); System.out.println("Here is the list of mayors, sorted by last name:"); for(FullName mayorName : mayorList) System.out.println(mayorName); } } class Name implements Comparable { protected String fName, lName; public Name(String f, String l) { fName = f; lName = l; } // Compare two names: last names take precedence, so sorting is by last name. // The FullName class doesn't need to override this method. // (Middle names don't have to affect sorting.) @Override public int compareTo(Name other) { int lastNameCompareToResult = lName.compareTo(other.lName); // The String class implements Comparable, so it has .compareTo defined // already, so use that. if(lastNameCompareToResult == 0) // if last names are same return fName.compareTo(other.fName); // sort on first name else // last names are different, so sort on them: return lastNameCompareToResult; } } Output: We have a new mayor: Please enter first name Mark Please enter middle name E Please enter last name Farrell Here is the list of mayors, sorted by last name: Arthur Christ Agnos London Nicole Breed Willie Lewis Brown Mark E Farrell Dianne Goldman Feinstein Francis Michael Jordan Edwin Mah Lee George Richard Moscone Gavin Christopher Newsom **** If I change the filename of mayors.txt to something else before I run the program: *** We have a new mayor: Please enter first name David Please enter middle name Sen-Fu Please enter last name Chiu Error reading or writing file: mayors.txt (No such file or directory) Here is the list of mayors, sorted by last name: David Sen-Fu Chiu
Java Program
Write the FullName class to be a subclass of Name, so that it also stores a middle name. It needs a constructor and a toString() method.
Make sure:
FullName class definition as described above
Instantiating a FullName object from the new mayor's name entered by the user, and adding that object to the mayorList
Inputting the names from mayors.txt
Instantiating a FullName object for each of those names from the file and adding them to the mayorList
Using exception handling to catch errors that might occur in file i/o: your program should catch all exceptions. Your main method should not have a "throws" clause. Other methods may throw exceptions, as long as the main method catches them.
Code:
import java.io.*;
import java.util.*;
class Main
{
public static void main(String[] args)
{
Scanner inFile = null, keyboard;
String first, middle, last;
ArrayList<FullName> mayorList = new ArrayList<FullName>();
keyboard = new Scanner(System.in);
System.out.println("We have a new mayor:");
System.out.println("Please enter first name");
first = keyboard.nextLine();
System.out.println("Please enter middle name");
middle = keyboard.nextLine();
System.out.println("Please enter last name");
last = keyboard.nextLine();
// DO NOT CHANGE ANY OF THE ABOVE CODE.
// ADD YOUR CODE HERE.
// DO NOT CHANGE ANY OF THE BELOW CODE.
Collections.sort(mayorList);
System.out.println("Here is the list of mayors, sorted by last name:");
for(FullName mayorName : mayorList)
System.out.println(mayorName);
}
}
class Name implements Comparable<Name>
{
protected String fName, lName;
public Name(String f, String l)
{
fName = f;
lName = l;
}
// Compare two names: last names take precedence, so sorting is by last name.
// The FullName class doesn't need to override this method.
// (Middle names don't have to affect sorting.)
@Override
public int compareTo(Name other)
{
int lastNameCompareToResult = lName.compareTo(other.lName);
// The String class implements Comparable, so it has .compareTo defined
// already, so use that.
if(lastNameCompareToResult == 0) // if last names are same
return fName.compareTo(other.fName); // sort on first name
else // last names are different, so sort on them:
return lastNameCompareToResult;
}
}
Output:
We have a new mayor:
Please enter first name
Mark
Please enter middle name
E
Please enter last name
Farrell
Here is the list of mayors, sorted by last name:
Arthur Christ Agnos
London Nicole Breed
Willie Lewis Brown
Mark E Farrell
Dianne Goldman Feinstein
Francis Michael Jordan
Edwin Mah Lee
George Richard Moscone
Gavin Christopher Newsom
**** If I change the filename of mayors.txt to something else before I run the program: ***
We have a new mayor:
Please enter first name
David
Please enter middle name
Sen-Fu
Please enter last name
Chiu
Error reading or writing file: mayors.txt (No such file or directory)
Here is the list of mayors, sorted by last name:
David Sen-Fu Chiu

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

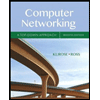
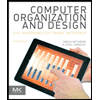
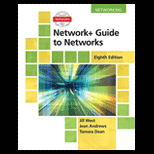
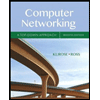
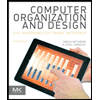
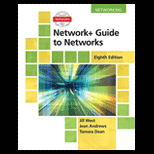
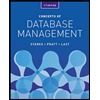
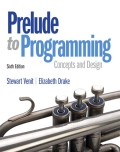
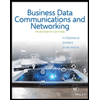