Given two integers that represent the miles to drive forward and the miles to drive in reverse as user inputs, create a SimpleCar variable that performs the following operations:
Given two integers that represent the miles to drive forward and the miles to drive in reverse as user inputs, create a SimpleCar variable that performs the following operations:
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter6: Modularity Using Functions
Section6.2: Returning A Single Value
Problem 12E
Related questions
Question
Given two integers that represent the miles to drive forward and the miles to drive in reverse as user inputs, create a SimpleCar variable that performs the following operations:

Transcribed Image Text:File is marked as read only
1 #ifndef SIMPLE_CAR_H
2 #define SIMPLE_CAR_H
3
4 typedef struct SimpleCar_struct {
5
int miles;
6} SimpleCar;
7
8 SimpleCar InitCar();
9 SimpleCar Drive(int dist, SimpleCar car);
10 SimpleCar Reverse(int dist, SimpleCar car);
11 int GetOdometer (SimpleCar car);
12 void HonkHorn (SimpleCar car);
13 void Report(SimpleCar car);
14
15 #endif
File is marked as read only
1 #include <stdio.h>
2 #include <string.h>
3
4 #include "SimpleCar.h"
5
6 SimpleCar InitCar() {
7
SimpleCar newCar;
8
9
newCar.miles = 0;
10
11
12 }
13
22
23
return newCar;
24 }
25
14 SimpleCar Drive(int dist, SimpleCar car) {
15
car.miles = car.miles + dist;
16
17
18 }
19
20 SimpleCar Reverse(int dist, SimpleCar car) {
car.miles = car.miles - dist;
21
return car;
return car;
26 int GetOdometer (SimpleCar car) {
27
return car.miles;
Current file: SimpleCar.h
28 }
29
30 void HonkHorn (SimpleCar car) {
31
printf("beep beep\n");
32 }
33
34 void Report(SimpleCar car){
35
36 }
Current file: SimpleCar.c
printf("Car has driven: %d miles\n", car.miles);

Transcribed Image Text:Given two integers that represent the miles to drive forward and the miles to drive in reverse as user inputs, create a SimpleCar variable that
performs the following operations:
Drives input number of miles forward
• Drives input number of miles in reverse
• Honks the horn
• Reports car status
.
SimpleCar.h contains the struct definition and related function declarations. SimpleCar.c contains related function definitions.
Ex: If the input is:
100 4
the output is:
beep beep
Car has driven: 96 miles
1 #include <stdio.h>
2
3 #include "SimpleCar.h"
4
5 int main() {
6
7
8
9
10 }
/* Type your code here. */
return 0;
Current file: main.c
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
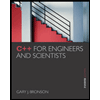
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
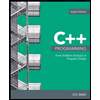
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
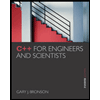
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
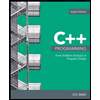
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning