Also, workers in skill level 3 can elect to participate in the retirement plan at 3% of their gross pay. Write an interactive Java payroll application that calculates the net pay for a factory worker. The program prompts the user for skill level and hours worked, as well as appropriate insurance and retirement options for the employee's skill level category. The application displays: (1) the hours worked, (2) the hourly pay rate, (3) the regular pay for 40 hours, (4) the overtime pay, (5) the total of regular and overtime pay, and (6) the total itemized deductions. If the deductions exceed the gross pay, display an error message; otherwise, calculate and display (7) the net pay after all the deductions have been subtracted from the gross. Save the file as Pay.java. Command Prompt - java Pay Input JavabatchFile>javac Pay.java 1JavabatchFile>java Pay Do you want the long term disability insurance? ? Enter 1 for Yes and 2 for No. Input OK Cancel Please enter skill levet: 1, 2, or 3 ? 2 OK Cancel Message Input ****** Gross Pay * Please enter hours worked: ? 45 Hours Worked: 45.0 Hourty Pay Rate: 20.0 Regular Pay: 800.0 Overtime Pay: 150.0 Total Pay: 950.0 OK Cancel Input ***** Deductions *** Medicat: 32.5 ? Do you want the medical insurance? Dental: 20.0 Enter 1 for Yes and 2 for No. Disability: 10.0 Retirement: 0.0 Total Deductions: 62.5 OK Cancel Net Pay: $887.5 OK Input Do you want the dental insurance? I did not print screens for every scenario (no overtime, no insurance, and no disability, etc., but I will check several different scenarios when I grade your program, so please check the program for logic/calculation errors to receive full credit. Enter 1 for Yes and 2 for No. OK Cancel
Also, workers in skill level 3 can elect to participate in the retirement plan at 3% of their gross pay. Write an interactive Java payroll application that calculates the net pay for a factory worker. The program prompts the user for skill level and hours worked, as well as appropriate insurance and retirement options for the employee's skill level category. The application displays: (1) the hours worked, (2) the hourly pay rate, (3) the regular pay for 40 hours, (4) the overtime pay, (5) the total of regular and overtime pay, and (6) the total itemized deductions. If the deductions exceed the gross pay, display an error message; otherwise, calculate and display (7) the net pay after all the deductions have been subtracted from the gross. Save the file as Pay.java. Command Prompt - java Pay Input JavabatchFile>javac Pay.java 1JavabatchFile>java Pay Do you want the long term disability insurance? ? Enter 1 for Yes and 2 for No. Input OK Cancel Please enter skill levet: 1, 2, or 3 ? 2 OK Cancel Message Input ****** Gross Pay * Please enter hours worked: ? 45 Hours Worked: 45.0 Hourty Pay Rate: 20.0 Regular Pay: 800.0 Overtime Pay: 150.0 Total Pay: 950.0 OK Cancel Input ***** Deductions *** Medicat: 32.5 ? Do you want the medical insurance? Dental: 20.0 Enter 1 for Yes and 2 for No. Disability: 10.0 Retirement: 0.0 Total Deductions: 62.5 OK Cancel Net Pay: $887.5 OK Input Do you want the dental insurance? I did not print screens for every scenario (no overtime, no insurance, and no disability, etc., but I will check several different scenarios when I grade your program, so please check the program for logic/calculation errors to receive full credit. Enter 1 for Yes and 2 for No. OK Cancel
Chapter9: Advanced Array Concepts
Section: Chapter Questions
Problem 10PE
Related questions
Question
Im stuck

Transcribed Image Text:| Also, workers in skill level 3 can elect to participate in the retirement plan at 3% of
their gross pay.
Write an interactive Java payroll application that calculates the net pay for a factory
worker. The program prompts the user for skill level and hours worked, as well as
appropriate insurance and retirement options for the employee's skill level category.
The application displays: (1) the hours worked, (2) the hourly pay rate, (3) the regular
pay for 40 hours, (4) the overtime pay, (5) the total of regular and overtime pay, and
(6) the total itemized deductions. If the deductions exceed the gross pay, display an
error message; otherwise, calculate and display (7) the net pay after all the deductions
have been subtracted from the gross. Save the file as Pay.java.
A Command Prompt - java Pay
Input
:\JavaBatchfile>javac Pay.java
:\JavabatchFile>java Pay
Do you want the long term disability insurance?
?
Enter 1 for Yes and 2 for No.
Input
OK
Cancel
Please enter skill level: 1, 2, or 3
?
2
OK
Cancel
Message
Input
******* Gross Pay ******
Hours Worked: 45.0
Please enter hours worked:
?
45
Hourly Pay Rate: 20.0
Regular Pay: 800.0
Overtime Pay: 150.0
OK
Cancel
Total Pay: 950.0
Input
******* Deductions *******
Medical: 32.5
?
Do you want the medical insurance?
Dental: 20.0
Enter 1 for Yes and 2 for No.
Disability: 10.0
1
Retirement: 0.0
Total Deductions: 62.5
OK
Cancel
Net Pay: $887.5
ок
Input
?
Do you want the dental insurance?
I did not print screens for every scenario (no overtime,
no insurance, and no disability, etc., but I will check
several different scenarios when I grade your program,
so please check the program for logic/calculation
errors to receive full credit.
Enter 1 for Yes and 2 for No.
OK
Cancel
Expert Solution

Step 1
Note:
- Comments mentioned in the code for understandability.
- In the implementation:
- If skill level =1 then hourlypay rate considered as 17.
- If skill level =2 then hourlypay rate considered as 20.
- If skill level =3 then hourlypay rate considered as 22.
Code:
import javax.swing.JOptionPane;
public class Pay
{
public static void main(String argds[])
{
//variable declaration
int REGULAR_HOURS =40;
int skillLevel;
int hoursWorked;
int medicalOption;
int dentalOption;
int disabilityOption;
double medicalCost=0.0;
double dentalCost=0.0;
double disabilityCost=0.0;
int retirementPlan;
double hourlyPayRate;
double regularPay;
int overtimeHoursWorked;
double overtimePay;
double totalPay;
double deductions;
double insuranceCost;
double retirementCost;
double netPay;
//Getting skill Level from user
do{
skillLevel =Integer.parseInt(JOptionPane.showInputDialog("Please Enter sill level: 1,2 or 3 "));
} while(skillLevel<1 || skillLevel>3);
//Getting hours worked from user
hoursWorked =Integer.parseInt(JOptionPane.showInputDialog("Please Enter Hours worked: "));
//getting Medical insurance option from user
do{
medicalOption =Integer.parseInt(JOptionPane.showInputDialog("Do you want the medical insurance?\nEnter 1 for Yes and 2 for No. "));
}while(medicalOption<1 || medicalOption>2);
// Getting dental insurance option from user
do{
dentalOption =Integer.parseInt(JOptionPane.showInputDialog("Do you want the dental insurance?\nEnter 1 for Yes and 2 for No. "));
}while(dentalOption<1 || dentalOption>2);
// Getting diability insurance option from user
do{
disabilityOption =Integer.parseInt(JOptionPane.showInputDialog("Do you want the long term disability insurance?\nEnter 1 for Yes and 2 for No. "));
}while(disabilityOption<1 || disabilityOption>2);
//Calculating hourly pay rate based on skill level
switch(skillLevel)
{
case 1:
hourlyPayRate = 17.00;
break;
case 2:
hourlyPayRate = 20.00;
break;
case 3:
hourlyPayRate = 22.00;
break;
default:
hourlyPayRate = 0.0;
}
//Calculating regular pay and overtime pay vased on hours worked
if(hoursWorked > 40)
{
regularPay = REGULAR_HOURS * hourlyPayRate;
overtimeHoursWorked = hoursWorked - REGULAR_HOURS;
overtimePay=overtimeHoursWorked*(hourlyPayRate*1.5);
}
else
{
regularPay = hoursWorked * hourlyPayRate;
overtimePay= 0.0;
}
//Claculate totalpay
totalPay = regularPay +overtimePay;
//Calculating Insurance cost based on medical,dental and disability insurance options.
insuranceCost= 0.0;
if(medicalOption == 1)
{
insuranceCost += 32.50;
medicalCost =32.50;
}
if(dentalOption == 1)
{
insuranceCost += 20.00;
dentalCost =20.00;
}
if(disabilityOption == 1)
{
insuranceCost += 10.00;
disabilityCost =10.00;
}
//calculating retiremnet cost based on skill level.
retirementCost=0.0;
if(skillLevel == 3)
{
retirementCost = (totalPay * 3)/100;
}
//Calculating deductions
deductions = insuranceCost + retirementCost;
//if deductions is greater than total pay then error message is displayed.
if(deductions > totalPay)
{
JOptionPane.showMessageDialog(null,"Error: the deductions exceed the gross pay, so deductions set to 0.");
deductions=0.0;
}
//calculating total pay
netPay = totalPay - deductions;
//Desgining a string to display output
String s1= "******* Gross Pay *******"
+"\n Hours Worked: "+hoursWorked
+"\n Hourly Pay rate: "+hourlyPayRate
+"\n Regular Pay: "+regularPay
+"\n Overtime Pay: "+overtimePay
+"\n Total Pay: "+totalPay
+ "\n\n******* Deductions *******"
+"\n Medical: "+medicalCost
+"\n Dental: "+dentalCost
+"\n Disability: "+disabilityCost
+"\n Retirement: "+retirementCost
+"\n Total deductions: "+deductions
+"\n\nNet Pay: "+netPay;
// Display string
JOptionPane.showMessageDialog(null,s1);
}
}
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 10 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
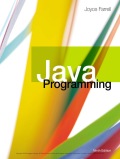
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
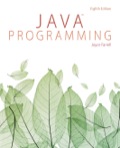
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
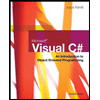
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
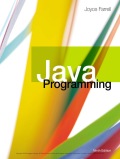
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
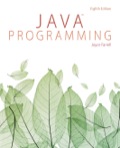
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
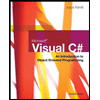
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,