art A Write a menu-driven program that will give the user the three choices: 1) Wage calculator, 2) Coupon Calculator, and 3) Exit. Class Name: PartA Wage Calculator: For the wage calculator, prompt for the name and hourly pay rate of an employee. Here the hourly pay rate is a floating-point number, such as $9.25. Then ask how many hours the employee worked in the past week. Be sure to accept fractional hours. Compute the pay. Any overtime work (over 40 hours per week) is paid at 150 percent of the regular wage (1.5 the hourly pay rate). Print the employee’s name, regular hours worked, regular hours pay, overtime hours worked (do not show overtime hours, if there are none), overtime hours pay (do not show overtime pay if there is none), and total pay. [Do not prompt for overtime hours] Coupon Calculator: For the coupon calculator, the total coup
In Java
Menu-Driven System Part A
Write a menu-driven
Coupon Calculator, and 3) Exit. Class Name: PartA
Wage Calculator: For the wage calculator, prompt for the name and hourly pay rate of an
employee. Here the hourly pay rate is a floating-point number, such as $9.25. Then ask how
many hours the employee worked in the past week. Be sure to accept fractional hours. Compute
the pay. Any overtime work (over 40 hours per week) is paid at 150 percent of the regular wage
(1.5 the hourly pay rate). Print the employee’s name, regular hours worked, regular hours pay,
overtime hours worked (do not show overtime hours, if there are none), overtime hours pay (do
not show overtime pay if there is none), and total pay. [Do not prompt for overtime hours]
Coupon Calculator: For the coupon calculator, the total coupon amount is calculated based on
the type of items purchased. Ask for the shopper’s total purchase amount. Then show the
following options to determine coupon amount: 1=Auto Parts, 2=Fragrances, 3=Accessories. If
the choice is 1, calculate a 10 percent coupon. If the choice is 2, calculate a 15 percent coupon. If
the choice is 3, calculate a 20 percent coupon. Print the choice and coupon amount in dollars and
cents. [Format: “Your coupon is $10.00 (Auto Parts)”]
Exit: The exit choice will display a statement that the program will end and thank the user for
using the program. [They must choose to exit the menu, not default out – it is a menu option]
Your program should be complete and with correct convention (ie. variable naming and
declaration/NO on-the-fly declarations) and commenting (correct header with well-formed
pseudocode). To obtain 50 of 100 points, your program must compile and begin execution. You
will obtain 30 points if your program runs correctly. You will obtain 20 points for all other
convention and commenting (this includes file and class naming convention). All output should
be properly formatted and user friendly. Your program should NOT loop on the menu (we will
execute the program for each option).
Once complete, you will submit your .java file using the ProjectPartA link in Moodle. See the
next page for an example program execution.


import java.util.*;
class partA
{
public static void main(String a[])
{
String name;
float hourlywage;
double hours, payAmount = 0.0;
int ex;
do
{
Scanner sc = new Scanner(System.in);
System.out.println("Calculator Menu:");
System.out.println("1) Wage calculator");
System.out.println("2) Coupon Calculator");
System.out.println("3) Exit");
System.out.println("Please enter your choice: ");
int option = sc.nextInt();
switch(option)
{
case 1:System.out.println("Please enter your name: ");
name=sc.next();
System.out.println("Please enter your hourly wage: ");
hourlywage=sc.nextFloat();
System.out.println("Please enter your hours worked: ");
hours=sc.nextInt();
if(hours>40.0)
{
payAmount=(hours * (hourlywage*1.5));
}
else
{
payAmount=hours*hourlywage;
}
System.out.println("Hello"+name);
System.out.println("your regular hours worked are "+hours+".");
System.out.println("your regular pay "+);
System.out.println("your overtime pay");
System.out.println("your total pay");
System.out.println("Thank you for using wage calculator "+name);
System.out.println("Have a grate day!");
break;
case 2: System.out.println("Please enter your purchase amount ");
System.out.println("plase enter purchase type (1=Auto parts, 2=Fragrences, 3=Accessories): ");
break;
case 3:System.out.println("This program will now end.");
System.out.println("Thank you for using our program!");
break;
case 4: break;
default: System.out.println("Invalid choice");
}
System.out.println("Do you want to continue?1.Yes 2.No");
ex=sc.nextInt();
}while(ex==1);
}
}
Step by step
Solved in 2 steps

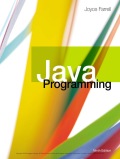
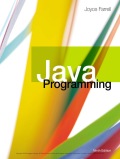