# Lab Assignment 14
.py
keyboard_arrow_up
School
Weber State University *
*We aren’t endorsed by this school
Course
1400
Subject
Computer Science
Date
Apr 30, 2024
Type
py
Pages
2
Uploaded by CountOkapiPerson1132 on coursehero.com
# Lab Assignment 14: Card Clash
import random
# Card classes' info for every card
class Card:
def __init__(self, faceValue, suit):
self.faceValue = faceValue
self.suit = suit
# Display face value/type of cards in print
def display(self):
print(f"{self.faceValue} of {self.suit}")
# Deck class contains info for card deck
class Deck:
def __init__(self, acesLow, addJokers):
self.acesLow = acesLow
self.addJokers = addJokers
self.stack = list()
self.reset()
# Reset returns unshuffles deck def reset(self):
self.stack.clear()
suits = ["Spades", "Clubs", "Diamonds", "Hearts"]
face_values = ["A", "2", "3", "4", "5", "6", "7", "8", "9", "10", "J", "Q",
"K"]
for suit in suits:
for face_value in face_values:
self.stack.append(Card(face_value, suit))
if self.addJokers:
self.stack.append(Card("Joker", ""))
# Shuffle deck/draw cards
def shuffle(self):
random.shuffle(self.stack)
def draw(self):
return self.stack.pop(0)
# Game classes for card game
class CardGame:
def __init__(self):
self.deck = Deck(acesLow=False, addJokers=False)
self.round_number = 0
self.player_wins = 0
self.computer_wins = 0
# Deal cards for new round
def deal_cards(self):
self.round_number += 1
print(f"\nRound {self.round_number}:")
self.deck.reset()
self.deck.shuffle()
self.player_hand = [self.deck.draw() for _ in range(3)]
self.computer_hand = [self.deck.draw() for _ in range(3)]
print("\nYour hand:")
for i, card in enumerate(self.player_hand):
print(f"{i + 1}: {card.faceValue} of {card.suit}")
# Determine round winners
def determine_round_winner(self, player_card, computer_card):
if player_card.faceValue == computer_card.faceValue:
return "Tie"
elif player_card.faceValue > computer_card.faceValue:
return "Player"
else:
return "Computer"
# Play a round
def play_round(self):
print("Choose a card to play (1-3): ")
chosen_card_index = int(input()) - 1
player_card = self.player_hand.pop(chosen_card_index)
print(f"\nYou played: {player_card.faceValue} of {player_card.suit}")
# Computer's turn: Choose a random card to play
computer_card_index = random.randint(0, 2)
computer_card = self.computer_hand.pop(computer_card_index)
print(f"Computer played: {computer_card.faceValue} of {computer_card.suit}")
# Determine round winners
winner = self.determine_round_winner(player_card, computer_card)
print(f"\nThe winner of this round is: {winner}")
# Update score based on round winner
if winner == "Player":
self.player_wins += 1
elif winner == "Computer":
self.computer_wins += 1
# Start game
def play(self):
print("Welcome to Card Clash!")
print("You'll be dealt 3 cards, and so will the computer.")
print("Choose the card in your hand with the highest value to pit against the computer's to win.")
print("The player who wins 2 out of 3 rounds wins the game.")
print("Let's play!")
while True:
# Deal cards to play a round
self.deal_cards()
self.play_round()
# Check if either player won 2 rounds
if self.player_wins == 2:
print("\nCongratulations! You won!")
break
elif self.computer_wins == 2:
print("\nComputer wins. Better luck next time!")
break
else:
print("\nNo one has won 2 rounds yet. Starting the next round...")
game = CardGame()
game.play()
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
class City(object):
county = "Lake"
population = 3000000
def -_init__(self, name, state):
self.name = name
self.state = state
def region(self):
return "Midwest"
a) create an instance of this object, called X, for Chicago, Illinois
b) return the population of the instance X
c) change the county of the instance X from Lake to Cook
d) return the name of the state of the instance X
d) call the method region() on the instance X
arrow_forward
Focus on classes, objects, methods and good programming style
Your task is to create a BankAccount class.
Class name
BankAccount
Attributes
_balance
float
_pin
integer
Methods
init ()
get_pin()
check pin ()
deposit ()
withdraw ()
get_balance ()
The bank account will be protected by a 4-digit pin number (i.e. between 1000 and 9999). The
pin should be generated randomly when the account object is created. The initial balance should
be 0.
get_pin () should return the pin.
check_pin (pin) should check the argument against the saved pin and return True if it
matches, False if it does not.
deposit (amount) should receive the amount as the argument, add the amount to the account
and return the new balance.
withraw (amount) should check if the amount can be withdrawn (not more than is in
the account), If so, remove the argument amount from the account and return the new balance if
the transaction was successful. Return False if it was not.
get_balance () should return the current balance.…
arrow_forward
class Student: def __init__(self, id, fn, ln, dob, m='undefined'): self.id = id self.firstName = fn self.lastName = ln self.dateOfBirth = dob self.Major = m def set_id(self, newid): #This is known as setter self.id = newid def get_id(self): #This is known as a getter return self.id def set_fn(self, newfirstName): self.fn = newfirstName def get_fn(self): return self.fn def set_ln(self, newlastName): self.ln = newlastName def get_ln(self): return self.ln def set_dob(self, newdob): self.dob = newdob def get_dob(self): return self.dob def set_m(self, newMajor): self.m = newMajor def get_m(self): return self.m def print_student_info(self): print(f'{self.id} {self.firstName} {self.lastName} {self.dateOfBirth} {self.Major}')all_students = []id=100user_input = int(input("How many students: "))for x in range(user_input): firstName = input('Enter…
arrow_forward
Pet ClassDesign a class named Pet, which should have the following fields:
name: The name field holds the name of a pet.
type: The type field holds the type of animal that a pet is. Example values are "Dog", "Cat", and "Bird".
age: The age field holds the pet’s age.
The Pet class should also have the following methods:
setName: The setName method stores a value in the name
setType: The setType method stores a value in the type
setAge: The setAge method stores a value in the age
getName: The getName method returns the value of the name
getType: The getType method returns the value of the type
getAge: The getAge method returns the value of the age
Once you have designed the class, design a program that creates an object of the class and prompts the user to enter the name, type, and age of his or her pet. This data should be stored in the object. Use the object’s accessor methods to retrieve the pet’s name, type, and age and display this data on the screen. The output Should be…
arrow_forward
add override display methodusing System;
//base classclass Book{//data membersprivate string title;private string author;protected double price;//constructorpublic Book(string t, string a){title = t;author = a;price = 500;}//method to display detailspublic void display(){Console.WriteLine("Title:" + title + " author:" + author + " price:" + price);}}
//child classclass PopularBooks : Book {//constructorpublic PopularBooks(string t, string a) :base(t,a) {price = 50000;}}
class Program {static void Main() {//an array of 5 objectsBook[] B = new Book[5];//input title and authorfor(int i=0; i<5;i++){Console.Write("Input title: ");string name = Console.ReadLine();Console.Write("Input author name: ");string author = Console.ReadLine();//if author is popular,//call constructor of sub classif (author.Equals("Khaled Hosseini") || author.Equals("Oscar Wilde") || author.Equals("Rembrandt")){B[i] = new PopularBooks(name, author);}//call base class constructorelse B[i] = new Book(name,…
arrow_forward
Assignment:The BankAccount class models an account of a customer. A BankAccount has the followinginstance variables: A unique account id sequentially assigned when the Bank Account is created. A balance which represents the amount of money in the account A date created which is the date on which the account is created.The following methods are defined in the BankAccount class: Withdraw – subtract money from the balance Deposit – add money to the balance Inquiry on:o Balanceo Account ido Date created
arrow_forward
C# languageCreate a class for “Plane” having functionalities (methods) startengine(), fly() and land(). When ever engine starts it should reset the attribute TTK (Total travel kilometer) to zero and attribute Fuel to 100. On fly() it should add 10 kilometers if the Fuel attribute is greater than zero and decrement Fuel by 20.On land() it should print total distance covered. Write the code providing all necessary details. And then show working object of Plane in main().
arrow_forward
C++ Language
Activity 5: DERIVED CLASS
• Create a SAVING ACCOUNT class that inherits the class BANK ACCOUNT.
• Create a constructor for the derived class that accepts INTEREST RATE.
• Create a method that displays the INTEREST RATE.
• Create a method that computes the INTEREST (BALANCE * INTEREST RATE) and adds to the current BALANCE.
arrow_forward
Parking Ticket SimulatorFor this assignment you will design a set of classes that work together to simulate apolice officer issuing a parking ticket. The classes you should design are:• The Parkedcar Class: This class should simulate a parked car. The class's respon-sibilities are:To know the car's make, model, color, license number, and the number of min-utes that the car has been parkedThe BarkingMeter Class: This class should simulate a parking meter. The class'sonly responsibility is:- To know the number of minutes of parking time that has been purchased• The ParkingTicket Class: This class should simulate a parking ticket. The class'sresponsibilities areTo report the make, model, color, and license number of the illegally parked carTo report the amount of the fine, which is $2S for the first hour or part of anhour that the car is illegally parked, plus $10 for every additional hour or part ofan hour that the car is illegally parkedTo report the name and badge number of the police…
arrow_forward
Dynamic Games
Instructions:
Write a class called Game that contains a video game’s name, genre, and difficultyLevel. Include a default constructor and destructor for the class. The constructor should print out the following message: “Creating a new game”. The destructor should print out the following message: “In the Game destructor.” Include appropriate get/set functions for the class.
In main(), prompt the user to enter the number of games he or she has played in the past year. Dynamically create a built-in array based on this number (not a vector or object of the array class) to hold pointers to Game objects.
Construct a loop in main() that executes once for each of the number of games that the user indicated. Within this loop, ask the user to enter the name and genre of each game. Using a random number generator, generate a difficultyLevel between 1-10 (inclusive). Seed this random number generator with 100. Next, dynamically create a Game object (remember that this…
arrow_forward
class TicTacToePlayer:
# Player for a game of Tic Tac Toe
def __init__(self, symbol, name):
self.symbol = symbol
self.name = name
def move(self, board):
move = int(input(self.name + " make your move 1-9:"))
move -= 1
y = move % 3
x = move // 3
board[x][y] = self.symbol
def is_symbol(self, symbol):
if symbol == self.symbol:
return True
class TicTacToe:
# Game of TicTacToe in a class!
def __init__(
self, p1_symbol="X", p2_symbol="O", p1_name="Player1", p2_name="Player2"
):
self.p1 = TicTacToePlayer(p1_symbol, p1_name)
self.p2 = TicTacToePlayer(p2_symbol, p2_name)
self.board = [[" " for x in range(3)] for x in range(3)]
def play_game(self):
turn = 0
winner_symbol = False
while not winner_symbol:
self._print_board()
if turn % 2:
self.p2.move(self.board) # replace this line with a call…
arrow_forward
Student Name:
8) Consider the following ciass definitions.
public class LibraryID{
private String name; // Person's name
private int ID;
// Person's library ID #
private ArrayList Books; // Loaned books
public LibraryID (String n, int num) {
name = n;
ID = num;
Books = new ArrayList(); }
public int getID () {
return ID; }
public String getName() {
return name; }
/* CheckOut () and ReturnBook () methods not shown */
Complete the following override method, contained in the LibraryID class. Two LibraryID's are
considered the same if their ID numbers are the same.
public boolean equals (Object other) {
if (other == null){
return false;}
LibraryID o = (LibraryID) other;
9) Assuming the code written in 8 is correct, write the call to compare LibraryID one and
two inside the if statement.
LibraryID one = new LibraryID("Mankind", 106);
LibraryID two = new LibraryID ("Mick Foley", 106);
if
System.out.println("ID's belong to the same person");
else
System.out.println("ID's belong to the…
arrow_forward
Exercises abstract class
• Design a new Triangle class that extends the abstract
GeometricObject class:
• Draw the UML diagram for the classes Triangle and
GeometricObject
• Implement the Triangle class
• Write a test program that prompts the user to enter three sides of the
triangle, a color, and a Boolean value to indicate whether the triangle is
filled:
• The program should create a Triangle object with these sides and
set the color and filled properties using the input
• The program should display the area, perimeter, color, and true or
false to indicate whether it is filled or not
arrow_forward
StockList Class
• This class will contain the methods necessary to display various information about the stock data.
• This class shall be a subclass of ArrayList.
• This class shall not contain any data fields. (You don't need them for this class if done correctly).
• This class shall have a default constructor with no parameters.
o No other constructors are allowed.
• This class shall have the following public, non-static, methods:
• printAllStocks:
This method shall take no parameters and return nothing.
▪ This method should print each stock in the StockList to an output file.
▪ NOTE: This method shall NOT print the data to the console.
The output file shall be called all_stock_data.txt.
The data should be printed in a nice and easy to read format.
o displayFirstTenStocks:
This method shall take no parameters and return nothing.
▪ This method should display only the first 10 stocks in the StockList in a nice and easy to read format.
▪ This method should display the data in the…
arrow_forward
[] class Person:
def
_init_(self, first_name, last_nam
self.first_name = first_name
self.last_name
self.age
self.gender = gender
self.hobbies = hobbies
%3D
last_name
= age
%3D
%3D
def year_passed(self):
self.age += 1
print(f'Happy birthday {self.first_nai
def add_hobby(self, hobby):
# write code to add a hobby to this p
# start of code
# end of code
# write a function that removes a hobby
# end of code
arrow_forward
Book Donation App
Create a book-app directory. The app can be used to manage book donations and track donors and books. The catalog is implemented using the following classes:
1. The app should have donors-repo.js to maintain the list of donors and allow adding, updating, and deleting donors. The donor object has donorID, firstName, lastName, and email properties. This module should implement the following functions:
• getDonor(donorId): returns a donor by id.
• addDonor(donor): adds a donor to the list of donors; donorID should be autoassigned a random number.
• updateDonor(donor): updates the donor having the matching donorID.
• deleteDonor(donorID): delete the donor with donorID from the list of donors, only if they are not associated with any books.
2. The app should have books-repo.js to maintain the list of donated books and allow adding, updating, and deleting books. The book object has bookID, title, authors, and donorID properties.
• donorID references the book’s donor. This…
arrow_forward
Hands-on Activity
Blood Bank (Part 1)
Objective:
At the end of the activity, the student should be able to:
Create and overload constructors.
Procedure:
Develop a simple program that stores a patient's blood Create two (2) classes named BloodData (no class modifier) and RunBloodData (public).
For the BloodData class: declare two (2) static String fields named bloodType for accepting (O, A, B, and AB) and rhFactor (stands for Rhesus factor, an inherited protein found on the surface of red blood cells) for accepting + and -.
For the default constructor (public) of the BloodData class, set bloodType to "O" and rhFactor to '+'.
Create an overloaded constructor (public) with two (2) String parameters: bt and rh. In this constructor, bloodType should store bt while rhFactor should store rh.
Create a public method named display. This method will be used to display the values of bloodType and rhFactor.
In the RunBloodData class, import the Scanner class for the user input.
In the main…
arrow_forward
Parent Class: Food
Write a parent class called Food. A food is described by a name, the number of grams of sugar (as a whole number), and the number of grams of sodium (as a whole number).
Core Class Components
For the Food class, write:
the complete class header
the instance data variables
a constructor that sets the instance data variables based on parameters
getters and setters; use validity checking on the parameters where appropriate
a toString method that returns a text representation of a Food object that includes all three characteristics of the food
Class-Specific Method
Write a method that calculates what percent of the daily recommended amount of sugar is contained in a food. The daily recommended amount might change, so the method takes in the daily allowance and then calculates the percentage.
For example, let's say a food had 6 grams of sugar. If the daily allowance was 24 grams, the percent would be 0.25. For that same food, if the daily allowance was 36 grams, the…
arrow_forward
Library Information System Design and Testing
Library Item Class Design and TestingDesign a class that holds the Library Item Information, with item name, author, publisher. Write appropriate accessor and mutator methods. Also, write a tester program(Console and GUI Program) that creates three instances/objects of the Library Items class.
Extending Library Item Class Library and Book Classes: Extend the Library Item class in (1) with a Book class with data attributes for a book’s title, author, publisher and an additional attributes as number of pages, and a Boolean data attribute indicating whether there is both hard copy as well as eBook version of the book. Demonstrate Book Class in a Tester Program (Console and GUI Program) with an object of Book class.
arrow_forward
Make Album in c++
You are going to develop a new class named Album. This class will has (at a minimum) teh following attributes and features:
Attributes:
Album Title
Artist Name
Array of song names (Track Listing)
Array of track lengths (decide on a unit)
At most there can be 20 songs on an album.
Behaviors:
Default and at least one argumented constructor
Appropriate getters for attributes
bool addTrack(string name, type duration) - adds track to album. You decide type on duration. Make sure you document what dis is.
getAlbumLength() - method dat returns total length of album matching watever units / type you decide.
getAlbumLength_string() - method dat returns total length of album in mm:ss format string. I would suggest using you're other getLength function here.
string shuffleAlbum(int n) - method dat returns a formatted string made up of n random tracks from teh album.
The string will be in the format [1] track_name (mm:ss), [2] track_name (mm:ss)...
Well formatted print()…
arrow_forward
Make Album in c++
You are going to develop a new class named Album. This class will has (at a minimum) teh following attributes and features:
Attributes:
Album Title
Artist Name
Array of song names (Track Listing)
Array of track lengths (decide on a unit)
At most there can be 20 songs on an album.
Behaviors:
Default and at least one argumented constructor
Appropriate getters for attributes
bool addTrack(string name, type duration) - adds track to album. You decide type on duration. Make sure you document what dis is.
getAlbumLength() - method dat returns total length of album matching watever units / type you decide.
getAlbumLength_string() - method dat returns total length of album in mm:ss format string. I would suggest using you're other getLength function here.
string shuffleAlbum(int n) - method dat returns a formatted string made up of n random tracks from teh album.
The string will be in the format [1] track_name (mm:ss), [2] track_name (mm:ss)...
Well formatted print()…
arrow_forward
Employee class
Write a Python program employee.py that computes the cumulative salary of employees based on the length of their contract and their annual merit rate.
Your program must include a class Employee with the following requirements:
Class attributes:
name: the name of the employee
position: the position of the employee
start_salary: the starting salary of the employee
annual_rate: the annual merit rate on the salary
contract_years: the number of years of salary
Class method:
get_cumulative_salary(): calculates and returns the cumulative salary of an employee based on the number of contract years. Round the cumulative salary to two digits after the decimal point.
Example: If start_salary = 100000, annual_rate = 5% and contract_years = 3:
Then the cumulative salary should be : 100000 + 105000 + 110250 = 315250
Outside of the class Employee, the program should have two functions:
Function name
Function description
Function input(s)
Function output(s) / return…
arrow_forward
T/F
A method defined in a class can access the class' instance data without needing to pass them as parameters or declare them as local variables.
arrow_forward
Focus on classes, objects, methods and good programming style
Your task is to create a BankAccount class(See the pic attached)
The bank account will be protected by a 4-digit pin number (i.e. between 1000 and 9999). The pin should be generated randomly when the account object is created. The initial balance should be 0.
get_pin()should return the pin.
check_pin(pin) should check the argument against the saved pin and return True if it matches, False if it does not.
deposit(amount) should receive the amount as the argument, add the amount to the account and return the new balance.
withraw(amount) should check if the amount can be withdrawn (not more than is in the account), If so, remove the argument amount from the account and return the new balance if the transaction was successful. Return False if it was not.
get_balance() should return the current balance.
Finally, write a main() to demo your bank account class. Present a menu offering a few actions and perform the action the user…
arrow_forward
Question 31
class Person:
def __init__(mysillyobject, name, age):
= name
mysillyobject.name
mysillyobject.age = age
def myfunc(abc):
print("Hello my age is", abc.age)
p1 = Person("John", 36)
p1.age = 40
p1.myfunc()
O 40
Hello my age is 40
36
Hello my age is 36
arrow_forward
14.23 LAB: Dean's list
Students make the Dean's list if their GPA is 3.5 or higher. Complete the Course class by implementing the get_deans_list() instance
method, which returns a list of students with a GPA of 3.5 or higher.
The file main.py contains:
. The main function for testing the program.
Class Course represents a course, which contains a list of student objects as a course roster. (Type your code in here.)
• Class Student represents a classroom student, which has three attributes: first name, last name, and GPA. (Hint: get_gpa() returns a
student's GPA.)
Note: For testing purposes, different student values will be used.
Ex. For the following students:
Henry Nguyen 3.5
Brenda Stern 2.0
Lynda Robison 3.2
Sonya King 3.9
the output is:
Dean's list:
Henry Nguyen (GPA: 3.5)
Sonya King (GPA: 3.9)
arrow_forward
Child Class: Vegetable
Write a child class called Vegetable. A vegetable is described by a name, the number of grams of sugar (as a whole number), the number of grams of sodium (as a whole number), and whether or not the vegetable is a starch.
Core Class Components
For the Vegetable class, write:
the complete class header
the instance data variables
a constructor that sets the instance data variables based on parameters
getters and setters; use instance data variables where appropriate
a toString method that returns a text representation of a Vegetable object that includes all four characteristics of the vegetableJava
arrow_forward
True or False Objects that are instances of a class can be stored in an array.
arrow_forward
11:02
all 10%
+
Create 1 1000.01
Create 2 2000.02
Create 3 3000.03
Deposit 111.11
Deposit 2 22.22
Withdraw 4 5000.00
Create 4 4000.04
Withdraw 1 0.10
Balance 2
Withdraw 2 0.20
Deposit 3 33.33
Withdraw 4 0.40
Bad Command 65
Balance 1
Balance 2
Balance 3
Balance 4
arrow_forward
// volunteer.h
#include
class Volunteer {
public:
Volunteer () { }
std::string Name ()
void SetName (const
private:
std::string name_;
};
const { return name_; }
std::string& name) { name_
= name; }
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
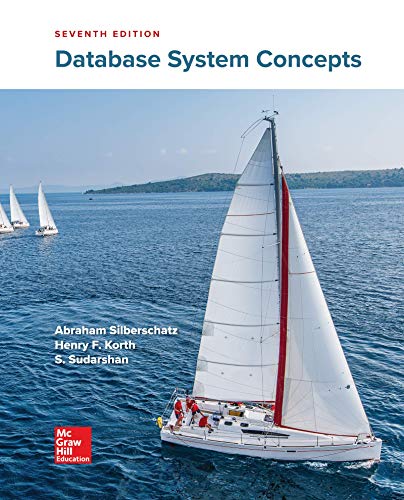
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
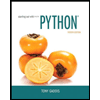
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
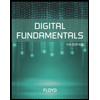
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
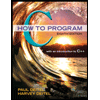
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
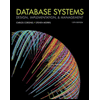
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
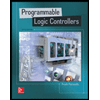
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education
Related Questions
- class City(object): county = "Lake" population = 3000000 def -_init__(self, name, state): self.name = name self.state = state def region(self): return "Midwest" a) create an instance of this object, called X, for Chicago, Illinois b) return the population of the instance X c) change the county of the instance X from Lake to Cook d) return the name of the state of the instance X d) call the method region() on the instance Xarrow_forwardFocus on classes, objects, methods and good programming style Your task is to create a BankAccount class. Class name BankAccount Attributes _balance float _pin integer Methods init () get_pin() check pin () deposit () withdraw () get_balance () The bank account will be protected by a 4-digit pin number (i.e. between 1000 and 9999). The pin should be generated randomly when the account object is created. The initial balance should be 0. get_pin () should return the pin. check_pin (pin) should check the argument against the saved pin and return True if it matches, False if it does not. deposit (amount) should receive the amount as the argument, add the amount to the account and return the new balance. withraw (amount) should check if the amount can be withdrawn (not more than is in the account), If so, remove the argument amount from the account and return the new balance if the transaction was successful. Return False if it was not. get_balance () should return the current balance.…arrow_forwardclass Student: def __init__(self, id, fn, ln, dob, m='undefined'): self.id = id self.firstName = fn self.lastName = ln self.dateOfBirth = dob self.Major = m def set_id(self, newid): #This is known as setter self.id = newid def get_id(self): #This is known as a getter return self.id def set_fn(self, newfirstName): self.fn = newfirstName def get_fn(self): return self.fn def set_ln(self, newlastName): self.ln = newlastName def get_ln(self): return self.ln def set_dob(self, newdob): self.dob = newdob def get_dob(self): return self.dob def set_m(self, newMajor): self.m = newMajor def get_m(self): return self.m def print_student_info(self): print(f'{self.id} {self.firstName} {self.lastName} {self.dateOfBirth} {self.Major}')all_students = []id=100user_input = int(input("How many students: "))for x in range(user_input): firstName = input('Enter…arrow_forward
- Pet ClassDesign a class named Pet, which should have the following fields: name: The name field holds the name of a pet. type: The type field holds the type of animal that a pet is. Example values are "Dog", "Cat", and "Bird". age: The age field holds the pet’s age. The Pet class should also have the following methods: setName: The setName method stores a value in the name setType: The setType method stores a value in the type setAge: The setAge method stores a value in the age getName: The getName method returns the value of the name getType: The getType method returns the value of the type getAge: The getAge method returns the value of the age Once you have designed the class, design a program that creates an object of the class and prompts the user to enter the name, type, and age of his or her pet. This data should be stored in the object. Use the object’s accessor methods to retrieve the pet’s name, type, and age and display this data on the screen. The output Should be…arrow_forwardadd override display methodusing System; //base classclass Book{//data membersprivate string title;private string author;protected double price;//constructorpublic Book(string t, string a){title = t;author = a;price = 500;}//method to display detailspublic void display(){Console.WriteLine("Title:" + title + " author:" + author + " price:" + price);}} //child classclass PopularBooks : Book {//constructorpublic PopularBooks(string t, string a) :base(t,a) {price = 50000;}} class Program {static void Main() {//an array of 5 objectsBook[] B = new Book[5];//input title and authorfor(int i=0; i<5;i++){Console.Write("Input title: ");string name = Console.ReadLine();Console.Write("Input author name: ");string author = Console.ReadLine();//if author is popular,//call constructor of sub classif (author.Equals("Khaled Hosseini") || author.Equals("Oscar Wilde") || author.Equals("Rembrandt")){B[i] = new PopularBooks(name, author);}//call base class constructorelse B[i] = new Book(name,…arrow_forwardAssignment:The BankAccount class models an account of a customer. A BankAccount has the followinginstance variables: A unique account id sequentially assigned when the Bank Account is created. A balance which represents the amount of money in the account A date created which is the date on which the account is created.The following methods are defined in the BankAccount class: Withdraw – subtract money from the balance Deposit – add money to the balance Inquiry on:o Balanceo Account ido Date createdarrow_forward
- C# languageCreate a class for “Plane” having functionalities (methods) startengine(), fly() and land(). When ever engine starts it should reset the attribute TTK (Total travel kilometer) to zero and attribute Fuel to 100. On fly() it should add 10 kilometers if the Fuel attribute is greater than zero and decrement Fuel by 20.On land() it should print total distance covered. Write the code providing all necessary details. And then show working object of Plane in main().arrow_forwardC++ Language Activity 5: DERIVED CLASS • Create a SAVING ACCOUNT class that inherits the class BANK ACCOUNT. • Create a constructor for the derived class that accepts INTEREST RATE. • Create a method that displays the INTEREST RATE. • Create a method that computes the INTEREST (BALANCE * INTEREST RATE) and adds to the current BALANCE.arrow_forwardParking Ticket SimulatorFor this assignment you will design a set of classes that work together to simulate apolice officer issuing a parking ticket. The classes you should design are:• The Parkedcar Class: This class should simulate a parked car. The class's respon-sibilities are:To know the car's make, model, color, license number, and the number of min-utes that the car has been parkedThe BarkingMeter Class: This class should simulate a parking meter. The class'sonly responsibility is:- To know the number of minutes of parking time that has been purchased• The ParkingTicket Class: This class should simulate a parking ticket. The class'sresponsibilities areTo report the make, model, color, and license number of the illegally parked carTo report the amount of the fine, which is $2S for the first hour or part of anhour that the car is illegally parked, plus $10 for every additional hour or part ofan hour that the car is illegally parkedTo report the name and badge number of the police…arrow_forward
- Dynamic Games Instructions: Write a class called Game that contains a video game’s name, genre, and difficultyLevel. Include a default constructor and destructor for the class. The constructor should print out the following message: “Creating a new game”. The destructor should print out the following message: “In the Game destructor.” Include appropriate get/set functions for the class. In main(), prompt the user to enter the number of games he or she has played in the past year. Dynamically create a built-in array based on this number (not a vector or object of the array class) to hold pointers to Game objects. Construct a loop in main() that executes once for each of the number of games that the user indicated. Within this loop, ask the user to enter the name and genre of each game. Using a random number generator, generate a difficultyLevel between 1-10 (inclusive). Seed this random number generator with 100. Next, dynamically create a Game object (remember that this…arrow_forwardclass TicTacToePlayer: # Player for a game of Tic Tac Toe def __init__(self, symbol, name): self.symbol = symbol self.name = name def move(self, board): move = int(input(self.name + " make your move 1-9:")) move -= 1 y = move % 3 x = move // 3 board[x][y] = self.symbol def is_symbol(self, symbol): if symbol == self.symbol: return True class TicTacToe: # Game of TicTacToe in a class! def __init__( self, p1_symbol="X", p2_symbol="O", p1_name="Player1", p2_name="Player2" ): self.p1 = TicTacToePlayer(p1_symbol, p1_name) self.p2 = TicTacToePlayer(p2_symbol, p2_name) self.board = [[" " for x in range(3)] for x in range(3)] def play_game(self): turn = 0 winner_symbol = False while not winner_symbol: self._print_board() if turn % 2: self.p2.move(self.board) # replace this line with a call…arrow_forwardStudent Name: 8) Consider the following ciass definitions. public class LibraryID{ private String name; // Person's name private int ID; // Person's library ID # private ArrayList Books; // Loaned books public LibraryID (String n, int num) { name = n; ID = num; Books = new ArrayList(); } public int getID () { return ID; } public String getName() { return name; } /* CheckOut () and ReturnBook () methods not shown */ Complete the following override method, contained in the LibraryID class. Two LibraryID's are considered the same if their ID numbers are the same. public boolean equals (Object other) { if (other == null){ return false;} LibraryID o = (LibraryID) other; 9) Assuming the code written in 8 is correct, write the call to compare LibraryID one and two inside the if statement. LibraryID one = new LibraryID("Mankind", 106); LibraryID two = new LibraryID ("Mick Foley", 106); if System.out.println("ID's belong to the same person"); else System.out.println("ID's belong to the…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
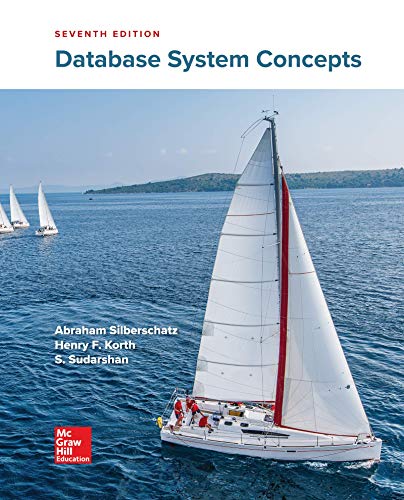
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
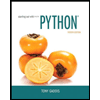
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
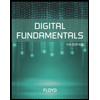
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
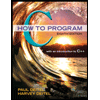
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
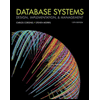
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
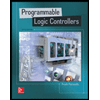
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education