Your checkWinner function should determine whether or not the game is over. If the game is over, it should print who won (if anyone), and return true. If the game is not over, it should return false. The game is over if someone wins by getting three X's or three O's in a row, column or diagonal. So there are 8 different combinations of 3-in-a-row moves. You may assume that the board has a size of 3 (length and width). The TicTacBoard.java file I'm giving you uses a variable for that size, but you can assume it's always 3. The game is also over if the board is full but nobody won. I recommend you write some other functions to help in determining if the game is over. Remember you should never copy and paste code if you can avoid it. Write a function to perform a common, generalizable task, and call that function every time you need it. (Like I did with my dispRow method.) Please follow the standard conventions for indentation, meaningful variable names, etc. like the examples in class and in the textbook. Make sure to run your program to test it out (using replit.com or the compiler of your choice), and then submit the TicTacBoard.java file you modified, plus your output showing how the program worked for you. class Main { /////// main //////// // No changes needed in this function. // It declares the variables, initializes the game, // and plays until someone wins or the game becomes unwinnable. publicstaticvoid main(String[] args) { TicTacBoard game = new TicTacBoard(3); game.playGame(); } }
Your checkWinner function should determine whether or not the game is over. If the game is over, it should print who won (if anyone), and return true. If the game is not over, it should return false. The game is over if someone wins by getting three X's or three O's in a row, column or diagonal. So there are 8 different combinations of 3-in-a-row moves. You may assume that the board has a size of 3 (length and width). The TicTacBoard.java file I'm giving you uses a variable for that size, but you can assume it's always 3. The game is also over if the board is full but nobody won. I recommend you write some other functions to help in determining if the game is over. Remember you should never copy and paste code if you can avoid it. Write a function to perform a common, generalizable task, and call that function every time you need it. (Like I did with my dispRow method.) Please follow the standard conventions for indentation, meaningful variable names, etc. like the examples in class and in the textbook. Make sure to run your program to test it out (using replit.com or the compiler of your choice), and then submit the TicTacBoard.java file you modified, plus your output showing how the program worked for you. class Main { /////// main //////// // No changes needed in this function. // It declares the variables, initializes the game, // and plays until someone wins or the game becomes unwinnable. publicstaticvoid main(String[] args) { TicTacBoard game = new TicTacBoard(3); game.playGame(); } }
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter4: Selection Structures
Section: Chapter Questions
Problem 14PP
Related questions
Question
- Your checkWinner function should determine whether or not the game is over. If the game is over, it should print who won (if anyone), and return true. If the game is not over, it should return false.
- The game is over if someone wins by getting three X's or three O's in a row, column or diagonal. So there are 8 different combinations of 3-in-a-row moves.
- You may assume that the board has a size of 3 (length and width). The TicTacBoard.java file I'm giving you uses a variable for that size, but you can assume it's always 3.
- The game is also over if the board is full but nobody won.
- I recommend you write some other functions to help in determining if the game is over. Remember you should never copy and paste code if you can avoid it. Write a function to perform a common, generalizable task, and call that function every time you need it. (Like I did with my dispRow method.)
- Please follow the standard conventions for indentation, meaningful variable names, etc. like the examples in class and in the textbook.
- Make sure to run your program to test it out (using replit.com or the compiler of your choice), and then submit the TicTacBoard.java file you modified, plus your output showing how the program worked for you.
class Main
{
/////// main ////////
// No changes needed in this function.
// It declares the variables, initializes the game,
// and plays until someone wins or the game becomes unwinnable.
publicstaticvoid main(String[] args)
{
TicTacBoard game = new TicTacBoard(3);
game.playGame();
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
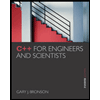
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
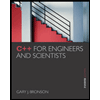
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr