Write Java classes to solve the following problem: A company has two types of employees. These are called Exempt and NonExempt. Exempt means the employee is not eligible for overtime pay and is paid a fixed amount each pay period. NonExempt means the employee is paid by the hour and receives 1.5 times the hourly rate for hours worked over 40. Each Employee has an id number which is a string. They also have a name. Employee should be an abstract class with the member variables described in number 2. Employee should have an abstract method called findPay, a toString method, and it should implement the Comparable interface. Use alphabetical order of id number to determine less than, greater than, and equal to. The toString method should produce a string like the following: Emp: ID: 4590 Emp Name: Fred Jones Employee should have an equals method. Define two employees to be equal if they have the same name and the same id number. Provide accessor and mutators for Employee. NonExempt should be derived from the abstract class Employee. NonExempt should have the additional member variables hoursWorked and hourlyRate. NonExempt should override the findPay method in Employee. If an employee works less than or equal to 40 hours, the pay = hourlyRate x hoursWorked. If the employee works more than 40 hours in one week, the pay is 40 x hourslyRate + 1.5 x hourlyRate x (hoursWorked -40). NonExempt should use the toString from Employee and add information as shown in the following example: Emp: ID : 4590; Emp Name: Fred Jones; Hr. Rate: 10.00; Hrs. Worked: 45 Reg. ; Pay: 400.00; OTime pay: 75.00; Total Pay: 475.00 Exempt should extend Employee. Exempt should have a variable called weeklySalary that holds an exempt employee’s weekly pay Exempt should implement the findPay method in Employee. This method simply returns the value stored in weeklySalary. The toString method for Exempt should use the super class toString plus code to return a string like the following: Emp: ID : 4590; Emp Name: Fred Jones; Pay: 1000 Both Exempt and NonExempt should provide accesors and mutators for their member variables. Remember that Employee should have accessors for id and name and is the parent for exempt and non-exempt classes.
Write Java classes to solve the following problem: A company has two types of employees. These are called Exempt and NonExempt. Exempt means the employee is not eligible for overtime pay and is paid a fixed amount each pay period. NonExempt means the employee is paid by the hour and receives 1.5 times the hourly rate for hours worked over 40. Each Employee has an id number which is a string. They also have a name. Employee should be an abstract class with the member variables described in number 2. Employee should have an abstract method called findPay, a toString method, and it should implement the Comparable interface. Use alphabetical order of id number to determine less than, greater than, and equal to. The toString method should produce a string like the following: Emp: ID: 4590 Emp Name: Fred Jones Employee should have an equals method. Define two employees to be equal if they have the same name and the same id number. Provide accessor and mutators for Employee. NonExempt should be derived from the abstract class Employee. NonExempt should have the additional member variables hoursWorked and hourlyRate. NonExempt should override the findPay method in Employee. If an employee works less than or equal to 40 hours, the pay = hourlyRate x hoursWorked. If the employee works more than 40 hours in one week, the pay is 40 x hourslyRate + 1.5 x hourlyRate x (hoursWorked -40). NonExempt should use the toString from Employee and add information as shown in the following example: Emp: ID : 4590; Emp Name: Fred Jones; Hr. Rate: 10.00; Hrs. Worked: 45 Reg. ; Pay: 400.00; OTime pay: 75.00; Total Pay: 475.00 Exempt should extend Employee. Exempt should have a variable called weeklySalary that holds an exempt employee’s weekly pay Exempt should implement the findPay method in Employee. This method simply returns the value stored in weeklySalary. The toString method for Exempt should use the super class toString plus code to return a string like the following: Emp: ID : 4590; Emp Name: Fred Jones; Pay: 1000 Both Exempt and NonExempt should provide accesors and mutators for their member variables. Remember that Employee should have accessors for id and name and is the parent for exempt and non-exempt classes.
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter11: Inheritance And Composition
Section: Chapter Questions
Problem 9PE
Related questions
Question
100%
Write Java classes to solve the following problem:
- A company has two types of employees. These are called Exempt and NonExempt. Exempt means the employee is not eligible for overtime pay and is paid a fixed amount each pay period. NonExempt means the employee is paid by the hour and receives 1.5 times the hourly rate for hours worked over 40.
- Each Employee has an id number which is a string. They also have a name.
- Employee should be an abstract class with the member variables described in number 2.
- Employee should have an abstract method called findPay, a toString method, and it should implement the Comparable interface. Use alphabetical order of id number to determine less than, greater than, and equal to. The toString method should produce a string like the following: Emp: ID: 4590 Emp Name: Fred Jones
- Employee should have an equals method. Define two employees to be equal if they have the same name and the same id number.
- Provide accessor and mutators for Employee.
- NonExempt should be derived from the abstract class Employee.
- NonExempt should have the additional member variables hoursWorked and hourlyRate.
- NonExempt should override the findPay method in Employee. If an employee works less than or equal to 40 hours, the pay = hourlyRate x hoursWorked. If the employee works more than 40 hours in one week, the pay is 40 x hourslyRate + 1.5 x hourlyRate x (hoursWorked -40).
- NonExempt should use the toString from Employee and add information as shown in the following example: Emp: ID : 4590; Emp Name: Fred Jones; Hr. Rate: 10.00; Hrs. Worked: 45 Reg. ; Pay: 400.00; OTime pay: 75.00; Total Pay: 475.00
- Exempt should extend Employee.
- Exempt should have a variable called weeklySalary that holds an exempt employee’s weekly pay
- Exempt should implement the findPay method in Employee. This method simply returns the value stored in weeklySalary.
- The toString method for Exempt should use the super class toString plus code to return a string like the following: Emp: ID : 4590; Emp Name: Fred Jones; Pay: 1000
- Both Exempt and NonExempt should provide accesors and mutators for their member variables. Remember that Employee should have accessors for id and name and is the parent for exempt and non-exempt classes.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
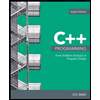
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
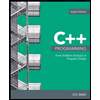
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning