Write c++ code Implement a class (template) SortedSet which is actually a singly linked list with head and tail pointers. It will store data in ascending order while disallowing duplicate data. Implement the following member functions for this class. 1. Default Constructor. SortedSet(); 2. An insert function that will insert the data such that resultant set is in ascending order. Duplicate data will not be allowed. void insert(T const data); 3. A delete function that will delete the element at the given index. void delete(int const index); 4. A print function that will print the contents of the sorted set. void print() const; 5. A union function that will be passed another sorted set. This function will take union of two sets and store the result in the first set. void union(SortedSetconst &otherSet); For example: SortedSet a; (suppose it has 1, 2, 3, 4, 10, 50) SortedSet b; (suppose it has 6, 10, 11) a.union(b); (a will now contain 1, 2, 3, 4, 6, 10, 11, 50) 6. An intersection function that will be passed another sorted set. This function will take intersection of two sets and store the result in the first set. void intersect(SortedSetconst & otherSet); You can create any other helper member functions for SortedSet if you want.
Write c++ code
Implement a class (template) SortedSet which is actually a singly linked list with head and tail pointers. It
will store data in ascending order while disallowing duplicate data. Implement the following member
functions for this class.
1. Default Constructor. SortedSet();
2. An insert function that will insert the data such that resultant set is in ascending order. Duplicate
data will not be allowed. void insert(T const data);
3. A delete function that will delete the element at the given index. void delete(int
const index);
4. A print function that will print the contents of the sorted set. void print() const;
5. A union function that will be passed another sorted set. This function will take union of two sets
and store the result in the first set. void union(SortedSet<T>const &otherSet);
For example:
SortedSet a; (suppose it has 1, 2, 3, 4, 10, 50)
SortedSet b; (suppose it has 6, 10, 11)
a.union(b); (a will now contain 1, 2, 3, 4, 6, 10, 11, 50)
6. An intersection function that will be passed another sorted set. This function will take
intersection of two sets and store the result in the first set. void
intersect(SortedSet<T>const & otherSet);
You can create any other helper member functions for SortedSet if you want.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 5 images

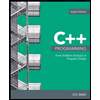
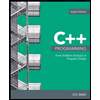