Write a program that asks the user to enter an item’s wholesale cost and its markup percentage. It should then display the item’s retail price. For example: • If an item’s wholesale cost is 5.00 and its markup percentage is 100 percent, then the item’s retail price is 10.00. • If an item’s wholesale cost is 5.00 and its markup percentage is 50 percent, then the item’s retail price is 7.50. The program should have a method named calculateRetail that receives the wholesale cost and the markup percentage as arguments, and returns the retail price of the item. Class name: RetailPriceCalculator
import java.util.Scanner;
public class RetailPriceCalculator {
public static double calculateRetail(double wholesale, double percentage) {
double retailPrice = wholesale + wholesale * (percentage / 100);
return retailPrice;
}
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
double wholesale = 0;
double percentage = 0;
// To check if it is the first or second input error
int n = 0;
boolean wholeSale = true;
boolean percent = true;
boolean condition = true;
while (condition) {
while (wholeSale) {
if (n == 0) {
System.out.println("Please enter the wholesale cost or -1 to exit: ");
} else {
System.out.println("Please enter the wholesale cost again or -1 to exit: ");
n = 0;
}
wholesale = sc.nextDouble();
if (wholesale == -1) {
condition = false; // Exit the loop
break;
} else if (wholesale < 0) {
System.out.println("Wholesale cost cannot be a negative value.");
n = 1; // Set the flag to indicate a second input error
continue; // Continue to prompt for input
}
wholeSale = false; // Valid input received, exit the inner loop
}
if (condition == false) {
break; // Exit the outer loop
}
while (percent) {
if (n == 0) {
System.out.println("Please enter the markup percentage or -1 to exit: ");
} else {
System.out.println("Please enter the markup again or -1 to exit: ");
n = 0;
}
percentage = sc.nextDouble();
if (percentage == -1) {
condition = false; // Exit the loop
break;
} else if (percentage < -100) {
System.out.println("Markup cannot be less than -100%.");
n = 1; // Set the flag to indicate a second input error
continue; // Continue to prompt for input
}
percent = false; // Valid input received, exit the inner loop
}
if (condition == false) {
break; // Exit the outer loop
}
wholeSale = true; // Reset the flag for the next input
percent = true; // Reset the flag for the next input
double retailPrice = calculateRetail(wholesale, percentage);
System.out.printf("The retail price is: %.2f\n", retailPrice);
}
}
}
Test Case 1
10ENTER
Please enter the markup percentage or -1 exit:\n
-1ENTER
Test Case 2
100ENTER
Please enter the markup percentage or -1 exit:\n
100ENTER
The retail price is: 200.00\n
Please enter the wholesale cost or -1 exit:\n
-1ENTER
Test Case 3
10ENTER
Please enter the markup percentage or -1 exit:\n
50ENTER
The retail price is: 15.00\n
Please enter the wholesale cost or -1 exit:\n
10ENTER
Please enter the markup percentage or -1 exit:\n
100ENTER
The retail price is: 20.00\n
Please enter the wholesale cost or -1 exit:\n
10ENTER
Please enter the markup percentage or -1 exit:\n
-100ENTER
The retail price is: 0.00\n
Please enter the wholesale cost or -1 exit:\n
-1ENTER
Test Case 4
-200ENTER
Wholesale cost cannot be a negative value.\n
Please enter the wholesale cost again or -1 exit:\n
10ENTER
Please enter the markup percentage or -1 exit:\n
-200ENTER
Markup cannot be less than -100%.\n
Please enter the markup again or -1 exit:\n
50ENTER
The retail price is: 15.00\n
Please enter the wholesale cost or -1 exit:\n
-1ENTER
Test Case 5
-1ENTER

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

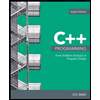
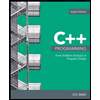