Write a complete program that uses loops and methods to print the "99 Bottles of Beer" song. Your song will print the words of the numbers (e.g., Ninety-nine instead of 99). The Song "99 Bottles of Beer" is a traditional, repeating sing-along song that counts down from some number to zero. If you are unfamiliar with the song, you can read the lyrics hereLinks to an external site. or watch it being sung hereLinks to an external site.. Part One: Read in the number and validate with a method Your program will allow the user to specify a starting number of bottles. This number can be anywhere between 1 and 99 (inclusive). Use a loop to continue asking the user for a number until it is valid, meaning in the specified range. Write a method that determines if the number if valid. Invoke that method to determine whether you need to keep looping. For full credit, use constants instead of hard-coded values to make code more readable and easier to maintain. Part Two: Print the song with words Write a method that prints the song starting at the user's number. Use a loop to print each verse. Write one or more helper methods that convert a number into the words (e.g., 43 into "Forty-three"). For full credit, you should have no more than 28 hard-coded Strings. All of the numbers between 1 and 99 can be represented with some combination of these 28 Strings: Ninety, Eighty, ..., Thirty, Twenty (8 strings) Nineteen, Eighteen, ..., Eleven, Ten (10 strings) Nine, Eight, ..., One, Zero (10 strings) Hint: think about how you can use integer division / and modulus % to access a number's tens-place digit and ones-place digit (e.g., for the number 62, 6 is the tens-place digit and 2 is the ones-place digit). Recommendation: Incremental Development I strongly suggest you work on building up your program a little at a time: code and test, code and test, etc. Here is one possible approach you might use in development. First, sketch out in words/pseudocode your approach. As part of this, write out what you think the method headers will be: the inputs, outputs, and a descriptive name. Make note of which methods you plan to call from other methods. (See below for the extra credit related to this step!) Then begin coding. After each step of development, test and revise until that step is fully functional. Read in and validate a user number using a method Print out the song using numbers (not words) (e.g., 99 bottles not Ninety-nine bottles) Convert the numbers to words Start with the numbers 0-9 only Then add code for the numbers 10-19 Finally, add code for the numbers 20-99 Note that you do not have to follow this approach. It is just a suggestion. But you should follow some plan of incremental development. Code and test small pieces at a time as you build up your full solution. Java
Write a complete program that uses loops and methods to print the "99 Bottles of Beer" song. Your song will print the words of the numbers (e.g., Ninety-nine instead of 99).
The Song
"99 Bottles of Beer" is a traditional, repeating sing-along song that counts down from some number to zero. If you are unfamiliar with the song, you can read the lyrics hereLinks to an external site. or watch it being sung hereLinks to an external site..
Part One: Read in the number and validate with a method
Your program will allow the user to specify a starting number of bottles. This number can be anywhere between 1 and 99 (inclusive). Use a loop to continue asking the user for a number until it is valid, meaning in the specified range.
Write a method that determines if the number if valid. Invoke that method to determine whether you need to keep looping.
For full credit, use constants instead of hard-coded values to make code more readable and easier to maintain.
Part Two: Print the song with words
Write a method that prints the song starting at the user's number. Use a loop to print each verse.
Write one or more helper methods that convert a number into the words (e.g., 43 into "Forty-three").
For full credit, you should have no more than 28 hard-coded Strings. All of the numbers between 1 and 99 can be represented with some combination of these 28 Strings:
- Ninety, Eighty, ..., Thirty, Twenty (8 strings)
- Nineteen, Eighteen, ..., Eleven, Ten (10 strings)
- Nine, Eight, ..., One, Zero (10 strings)
Hint: think about how you can use integer division / and modulus % to access a number's tens-place digit and ones-place digit (e.g., for the number 62, 6 is the tens-place digit and 2 is the ones-place digit).
Recommendation: Incremental Development
I strongly suggest you work on building up your program a little at a time: code and test, code and test, etc. Here is one possible approach you might use in development.
First, sketch out in words/pseudocode your approach. As part of this, write out what you think the method headers will be: the inputs, outputs, and a descriptive name. Make note of which methods you plan to call from other methods. (See below for the extra credit related to this step!)
Then begin coding. After each step of development, test and revise until that step is fully functional.
- Read in and validate a user number using a method
- Print out the song using numbers (not words) (e.g., 99 bottles not Ninety-nine bottles)
- Convert the numbers to words
- Start with the numbers 0-9 only
- Then add code for the numbers 10-19
- Finally, add code for the numbers 20-99
Note that you do not have to follow this approach. It is just a suggestion. But you should follow some plan of incremental development. Code and test small pieces at a time as you build up your full solution.
Java

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

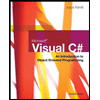
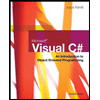