The Spring fork amateur golf club has a tournament every weekend. The club president has asked you to design a program. It contains the following menu: Select the task 1. Add new records 2. Read Records 3. End the program If the user enters 1, the program will read each players name and golf score as keyboard input, then save the records in a file names golf.dat If the user enters 2, the program reads the records from the golf.dat file and displays them. While the menu selection is not equal to 3, get the user selection. If the selection is 1, get the number of players in the tournament, open the output file. For each player, get the name and the score, and write them to the file. Close the file. If the selection is 2, open the input file, read the values from the file, display the values, close the file. If the selection is 3, exit the program. I already had a code written from before, but I'm having a hard time turning it into a menu driven code. It does need to have the function prototypes, definitions and function call. I need this in C++. Any advice would be greatly appreciated #include #include #include using namespace std; void golfPlayerDeatils(); int main() { golfPlayerDeatils(); return 0; } void golfPlayerDeatils(){ int players; int score; string name; ofstream outFile; outFile.open("golf.txt", ios::app); cout << "Enter the number of golfers competing: "; cin >> players; for(int i = 0; i < players; ++i) { cout << "Enter the name of the competitor " << (i+1) << ": "; cin >> name; cout << "Enter their score: " ; cin >> score; cout << "Golfer: " << name << " Score: " << score << endl; outFile <<"Golfer: " << name << " Score: " << score << endl; if (outFile.fail()) { cout << "Error creating file. " << endl; exit (1); } } outFile.close ();
The Spring fork amateur golf club has a tournament every weekend. The club president has asked you to design a program. It contains the following menu:
Select the task
1. Add new records
2. Read Records
3. End the program
If the user enters 1, the program will read each players name and golf score as keyboard input, then save the records in a file names golf.dat
If the user enters 2, the program reads the records from the golf.dat file and displays them.
While the menu selection is not equal to 3, get the user selection.
If the selection is 1, get the number of players in the tournament, open the output file. For each player, get the name and the score, and write them to the file. Close the file.
If the selection is 2, open the input file, read the values from the file, display the values, close the file.
If the selection is 3, exit the program.
I already had a code written from before, but I'm having a hard time turning it into a menu driven code. It does need to have the function prototypes, definitions and function call. I need this in C++. Any advice would be greatly appreciated
#include <iostream>
#include <string>
#include <fstream>
using namespace std;
void golfPlayerDeatils();
int main()
{
golfPlayerDeatils();
return 0;
}
void golfPlayerDeatils(){
int players;
int score;
string name;
ofstream outFile;
outFile.open("golf.txt", ios::app);
cout << "Enter the number of golfers competing: ";
cin >> players;
for(int i = 0; i < players; ++i)
{
cout << "Enter the name of the competitor " << (i+1) << ": ";
cin >> name;
cout << "Enter their score: " ;
cin >> score;
cout << "Golfer: " << name << " Score: " << score << endl;
outFile <<"Golfer: " << name << " Score: " << score << endl;
if (outFile.fail())
{
cout << "Error creating file. " << endl;
exit (1);
}
}
outFile.close ();
cout << "File is created";
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

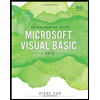
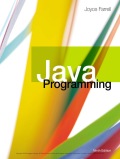
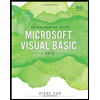
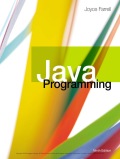