Python help please! Thank you! Add the following methods to your Boat Race class: Write a method called add_racer which takes in a Boat object and adds it to the end of the racers list. The function does not return anything. Write a method called print_racers which loops through racers and prints the Boat objects. This function takes in no parameters (other than self) and returns nothing. Write a method called count that returns the number of racers. Write a method called race. The race function calls the move function for all of the racers in the BoatRace. Once all the racers have moved, call the print_racers method to display information about the progress of each boat. Then, check if any of the racer’s current_progress is greater than or equal to the race’s distance. If so, then return a list of all of the racers whose current_progress is greater than or equal to distance. If no racer has finished the race then repeat the calls to move and check until at least one racer has finished the race. This is what I have so far: class BoatRace: def __init__(self, file_name): self.file_name = file_name with open(self.file_name, 'r') as file: lines = file.readlines() self.race_name = lines[0].split(",")[1].strip() self.race_id = int(lines[1].split(",")[1].strip()) self.distance = int(lines[2].split(",")[1].strip()) self.racers = [] for i in range(3, len(lines)): self.racers.append(Boat(lines[i].split(",")[0].strip(), int(lines[i].split(",")[1].strip()))) def get_race_name(self): return self.race_name def get_race_id(self): return self.race_id def get_distance(self): return self.distance def get_racers(self): return self.racers def add_racer(self): self.racers.append(boat)
Python help please! Thank you!
Add the following methods to your Boat Race class:
- Write a method called add_racer which takes in a Boat object and adds it to the end of the racers list. The function does not return anything.
- Write a method called print_racers which loops through racers and prints the Boat objects. This function takes in no parameters (other than self) and returns nothing.
- Write a method called count that returns the number of racers.
- Write a method called race.
- The race function calls the move function for all of the racers in the BoatRace.
- Once all the racers have moved, call the print_racers method to display information about the progress of each boat.
- Then, check if any of the racer’s current_progress is greater than or equal to the race’s distance.
- If so, then return a list of all of the racers whose current_progress is greater than or equal to distance.
- If no racer has finished the race then repeat the calls to move and check until at least one racer has finished the race.
This is what I have so far:
class BoatRace:
def __init__(self, file_name):
self.file_name = file_name
with open(self.file_name, 'r') as file:
lines = file.readlines()
self.race_name = lines[0].split(",")[1].strip()
self.race_id = int(lines[1].split(",")[1].strip())
self.distance = int(lines[2].split(",")[1].strip())
self.racers = []
for i in range(3, len(lines)):
self.racers.append(Boat(lines[i].split(",")[0].strip(), int(lines[i].split(",")[1].strip())))
def get_race_name(self):
return self.race_name
def get_race_id(self):
return self.race_id
def get_distance(self):
return self.distance
def get_racers(self):
return self.racers
def add_racer(self):
self.racers.append(boat)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

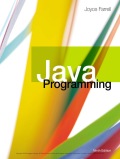
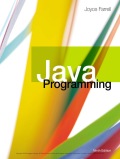