Programing C Just with #include Matrix Addition and Subtraction (Associatively) Write a program that performs matrices addition and subtraction. As shown in the example below, you have to ask the user for the number of rows and number of columns that both matrices should have (they both have to be the same size, that is why you only ask once). You will then ask the user for the values of each matrix. Then, you will print the values that the user inputted, the added values of both matrices, and the subtracted values (matrix 2 should be subtracted from matrix 1). In this challenge Guidelines/steps: • The number of rows and the number of columns should be global variables. • Once you get these two from the user, you should declare your two matrices in main. • Then, from main, you will call function aaa twice; first, you will send matrix 1 and populate it with values inputted by the user. You will call the function a second time, send matrix 2 and populate it. In other words, function aaa is the function that populates matrices. • Next, also from main (after calling function aaa), you will call function bbb twice. First, to send matrix 1 and print its values; then, to send matrix 2 and print its values. In other words, bbb is the function that prints the values of a matrix. • Afterwards, also in main, you will call function ccc. This function will receive both matrices. In here, your task will be to create a third (temporary) matrix with the same dimensions as the other two. You will use this matrix to hold the added values of the other two. Once the associative addition of all values is stored, you will call function bbb, and send the third (temporary) matrix to print the resulting added-values. Think of ccc as the function that adds matrices. Lastly, for the subtraction, you should do the same. Call function ddd in main. Send both matrices. Create a temporary matrix in ddd. Perform the subtraction (first matrix minus the second one). Send the resulting matrix to bbb. Function ddd will be the function that subtracts matrices. • None of the functions should return anything. How many rows will your matrices have? 3 How many columns will your matrices have? 2 Please input values of matrix 1: 1.1 -1.5 2.8 3.9 2.7 5.4 Please input values of matrix 2: -8.2 4.9 9.8 10.1 7.5 6.4 Values of matrix 1 are: 1.10 2.80 2.70 -1.50 3.90 5.40 Values of matrix 2 are: -8.20 9.80 4.90 10.10 7.50 6.40 Addition of both matrices results in: -7.10 12.60 10.20 3.40 14.00 11.80 If we subtract your second matrix from your first matrix, we get: 9.30 -6.40 -7.00 -4.80 -6.20 -1.00
Programing C Just with #include Matrix Addition and Subtraction (Associatively) Write a program that performs matrices addition and subtraction. As shown in the example below, you have to ask the user for the number of rows and number of columns that both matrices should have (they both have to be the same size, that is why you only ask once). You will then ask the user for the values of each matrix. Then, you will print the values that the user inputted, the added values of both matrices, and the subtracted values (matrix 2 should be subtracted from matrix 1). In this challenge Guidelines/steps: • The number of rows and the number of columns should be global variables. • Once you get these two from the user, you should declare your two matrices in main. • Then, from main, you will call function aaa twice; first, you will send matrix 1 and populate it with values inputted by the user. You will call the function a second time, send matrix 2 and populate it. In other words, function aaa is the function that populates matrices. • Next, also from main (after calling function aaa), you will call function bbb twice. First, to send matrix 1 and print its values; then, to send matrix 2 and print its values. In other words, bbb is the function that prints the values of a matrix. • Afterwards, also in main, you will call function ccc. This function will receive both matrices. In here, your task will be to create a third (temporary) matrix with the same dimensions as the other two. You will use this matrix to hold the added values of the other two. Once the associative addition of all values is stored, you will call function bbb, and send the third (temporary) matrix to print the resulting added-values. Think of ccc as the function that adds matrices. Lastly, for the subtraction, you should do the same. Call function ddd in main. Send both matrices. Create a temporary matrix in ddd. Perform the subtraction (first matrix minus the second one). Send the resulting matrix to bbb. Function ddd will be the function that subtracts matrices. • None of the functions should return anything. How many rows will your matrices have? 3 How many columns will your matrices have? 2 Please input values of matrix 1: 1.1 -1.5 2.8 3.9 2.7 5.4 Please input values of matrix 2: -8.2 4.9 9.8 10.1 7.5 6.4 Values of matrix 1 are: 1.10 2.80 2.70 -1.50 3.90 5.40 Values of matrix 2 are: -8.20 9.80 4.90 10.10 7.50 6.40 Addition of both matrices results in: -7.10 12.60 10.20 3.40 14.00 11.80 If we subtract your second matrix from your first matrix, we get: 9.30 -6.40 -7.00 -4.80 -6.20 -1.00
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter4: Selection Structures
Section: Chapter Questions
Problem 14PP
Related questions
Question
100%

Transcribed Image Text:Programing C
Just with #include<stdio.h>
Matrix Addition and Subtraction (Associatively)
Write a program that performs matrices addition and subtraction. As shown in the example below, you have to
ask the user for the number of rows and number of columns that both matrices should have (they both have to
be the same size, that is why you only ask once). You will then ask the user for the values of each matrix. Then,
you will print the values that the user inputted, the added values of both matrices, and the subtracted values
(matrix 2 should be subtracted from matrix 1). In this challenge
Guidelines/steps:
• The number of rows and the number of columns should be global variables.
• Once you get these two from the user, you should declare your two matrices in main.
• Then, from main, you will call function aaa twice; first, you will send matrix 1 and populate it with values
inputted by the user. You will call the function a second time, send matrix 2 and populate it. In other words,
function aaa is the function that populates matrices.
• Next, also from main (after calling function aaa), you will call function bbb twice. First, to send matrix 1 and
print its values; then, to send matrix 2 and print its values. In other words, bbb is the function that prints the
values of a matrix.
• Afterwards, also in main, you will call function ccc. This function will receive both matrices. In here, your
task will be to create a third (temporary) matrix with the same dimensions as the other two. You will use this
matrix to hold the added values of the other two. Once the associative addition of all values is stored, you will
call function bbb, and send the third (temporary) matrix to print the resulting added-values. Think of ccc as the
function that adds matrices.
Lastly, for the subtraction, you should do the same. Call function ddd in main. Send both matrices. Create a
temporary matrix in ddd. Perform the subtraction (first matrix minus the second one). Send the resulting matrix
to bbb. Function ddd will be the function that subtracts matrices.
• None of the functions should return anything.

Transcribed Image Text:How many rows will your matrices have? 3
How many columns will your matrices have? 2
Please input values of matrix 1:
1.1
-1.5
2.8
3.9
2.7
5.4
Please input values of matrix 2:
-8.2
4.9
9.8
10.1
7.5
6.4
Values of matrix 1 are:
1.10
2.80
2.70
-1.50
3.90
5.40
Values of matrix 2 are:
-8.20
9.80
4.90
10.10
7.50
6.40
Addition of both matrices results in:
-7.10
12.60
10.20
3.40
14.00
11.80
If we subtract your second matrix from your first matrix, we get:
9.30
-6.40
-7.00
-4.80
-6.20
-1.00
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 6 steps with 6 images

Recommended textbooks for you
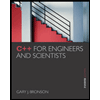
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
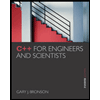
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr