Problem C. Front Tooth Concealment You have recently been appointed vice principal of a high school, and as such, you have the honor of helping out with their graduation ceremony. You will alternate reading off the names of the graduates with the principal. Unfortunately, you got your front teeth knocked out last night while fighting crime as a masked vigilante, so you are unable to pronounce any name containing the letters ‘s’ or ‘z’. Since you don’t want your enemies to learn of your secret identity, and you suspect that some of them may be present at graduation, you need to avoid saying any names containing the letters ‘s’ or ‘z’. You will read the first name from the list of graduates, meaning that you will also read the third, the fifth, the seventh, and so on. Therefore, you must ensure that all names at odd positions in the list of names (or at even indexes, since we start indexing at 0), do not contain the letters ‘s’ or ‘z’. Write a function avoid_sz(names_list) which takes in a list of strings representing the names of the students in the order they will be read. avoid_sz should return a new list containing the same set of names, except rearranged such that the strings at all even indexes do not contain the characters 's', 'z', or their upper case variants, 'S' or 'Z'. If this is not possible because there are too many names that contain ‘s’ or ‘z’, the function should instead print the string "Impossible: Too many s/z names", and return an empty list. Hints: ● You can use ('s' in word) to check whether the character 's' appears in the string word. Note that this only finds the lowercase version of the letter. ● Separate the names into two lists: names that contain ‘s’ or ‘z’, and names that don’t. Then recombine them in an order that avoids any names containing ‘s’ or ‘z’ in even indexed spots. This will likely require keeping track of indexes as you loop. Examples (text in bold is returned, text in italics is printed): >>> avoid_sz(['Sam Fountain', 'Andrew LaFortune']) ['Andrew LaFortune', 'Sam Fountain'] >>> avoid_sz(['Lucas Flom', 'Emily Welp', 'Akshay Peddi']) Impossible: Too many s/z names [] >>> avoid_sz(['Jessica Thorne', 'Manan Mrig', 'Elijha Gordon', 'Daniel Binsfeld', 'Abdullahi Abdullahi', 'Chris Park', 'Nathan Stearley', 'Aishwarya Belhe']) Impossible: Too many s/z names []
Problem C. Front Tooth Concealment
You have recently been appointed vice principal of a high school, and as such, you have the
honor of helping out with their graduation ceremony. You will alternate reading off the names of the graduates with the principal. Unfortunately, you got your front teeth knocked out last night while fighting crime as a masked vigilante, so you are unable to pronounce any name containing the letters ‘s’ or ‘z’. Since you don’t want your enemies to learn of your secret identity, and you suspect that some of them may be present at graduation, you need to avoid saying any names containing the letters ‘s’ or ‘z’. You will read the first name from the list of graduates, meaning that you will also read the third, the fifth, the seventh, and so on. Therefore, you must ensure that all names at odd positions in the list of names (or at even indexes, since we start indexing at 0), do not contain the letters ‘s’ or ‘z’.
Write a function avoid_sz(names_list) which takes in a list of strings representing the names of the students in the order they will be read. avoid_sz should return a new list containing the same set of names, except rearranged such that the strings at all even indexes do not contain the characters 's', 'z', or their upper case variants, 'S' or 'Z'. If this is not possible because there are too many names that contain ‘s’ or ‘z’, the function should instead print the string "Impossible: Too many s/z names", and return an empty list.
Hints:
-
● You can use ('s' in word) to check whether the character 's' appears in the string
word. Note that this only finds the lowercase version of the letter.
-
● Separate the names into two lists: names that contain ‘s’ or ‘z’, and names that don’t.
Then recombine them in an order that avoids any names containing ‘s’ or ‘z’ in even indexed spots. This will likely require keeping track of indexes as you loop.
Examples (text in bold is returned, text in italics is printed):
>>> avoid_sz(['Lucas Flom', 'Emily Welp', 'Akshay Peddi']) Impossible: Too many s/z names
>>> avoid_sz(['Sam Fountain', 'Andrew LaFortune']) ['Andrew LaFortune', 'Sam Fountain'][]
>>> avoid_sz(['Jessica Thorne', 'Manan Mrig', 'Elijha Gordon', 'Daniel Binsfeld', 'Abdullahi Abdullahi', 'Chris Park', 'Nathan Stearley', 'Aishwarya Belhe'])
Impossible: Too many s/z names[]

Answer:
Code:
def no_front_teeth(ma,es_list):
odd = []
even = []
newNames = []
threshold = len(names_list)//2
# Threshold is the number of even indices in the given names list
# It is the minimum number of names without s or z in them , that we must have
# If there are less than this number , then we have to fill an even index with s or z name
if len(names_list)%2 == 1:
threshols+=1
for name in names_list:
#separate names
if 's' in name.lower() or 'z' in name.lower():
odd.append(name)
else:
even.append(name)
if len(even)<threshold: #check if we have enough names to fill even index without having to use s or z name
print("Mission impossible:too many unpronounceable names")
return []
else:
for i in range(len(odd)):
#first fill all the s or z names at odd indices
newNames.append(even[i])
newNames.append(odd[i])
if len(even)>len(odd):
# if we have some names without s and z still left
for i in range(len(odd) , len(even)):
newNames.append(even[i])
#Append them at the end
return newNames
Step by step
Solved in 2 steps with 1 images

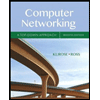
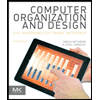
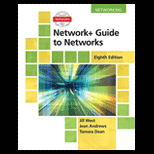
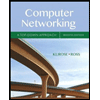
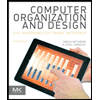
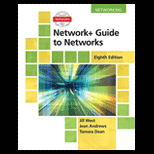
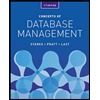
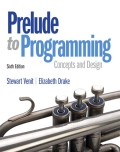
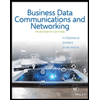