PART 1 - Complete the function below to deocompose # a compound formula written as a string # in a dictionary ###################################################### import chemparse def mol_form(compound_formula): """(str) -> dictionary When passed a string of the compound formula, returns a dictionary with the elements as keys and the number of atoms of that element as values. >>> mol_form("C2H6O") {'C': 2, 'H': 6, 'O': 1} >>> mol_form("CH4") {'C': 1, 'H': 4} """ print((chemparse.parse_formula(compound_formula))) mol_form("C2H6O") mol_form("CH4") ###################################################### # PART 2 - Complete the function below that takes two # tuples representing one side of a # chemical equation and returns a dictionary # with the elements as keys and the total # number of atoms in the entire expression # as values. ###################################################### def expr_form(expr_coeffs:tuple, expr_molecs: tuple) -> dict: """ (tuple (of ints), tuple (of dictionaries)) -> dictionary For example, consider the expression 2NaCl + H2 + 5NaF >>> expr_form((2,1,5), ({"Na":1, "Cl":1}, {"H":2}, {"Na":1, "F":1})) {'Na': 7, 'Cl': 2, 'H': 2, 'F': 5} """ # TODO your code here expr_Length = len(expr_molecs) expr_result= {} for i in range (expr_Length): coefficient = expr_coeffs[i] molecules = expr_molecs[i] for item, num in molecules.items(): if item not in expr_result: expr_result[item]= coefficient * num else: expr_result[item]+= coefficient * num return expr_result ######################################################## # PART 3 - Check if two dictionaries representing # the type and number of atoms on two sides of # a chemical equation contain different # key-value pairs ######################################################## def find_unbalanced_atoms(reactant_atoms, product_atoms): """ (Dict,Dict) -> Set >>> find_unbalanced_atoms({"H" : 2, "Cl" : 2, "Na" : 2}, {"H" : 2, "Na" : 1, "Cl" : 2}) {'Na'} >>> find_unbalanced_atoms({"H" : 2, "Cl" : 2, "Na" : 2}, {"H" : 2, "Na" : 2, "Cl" : 2}) set() >>> find_unbalanced_atoms({"H" : 2, "Cl" : 2, "Na" : 2}, {"H" : 2, "F" : 2, "Cl" : 2}) {'F', 'Na'} """ # TODO your code here unbalancedAtomsSetObjct = set() keysObjctOfreactant_atomsDict = reactant_atoms.keys() for itemOftheReactntAtomsDictnry in keysObjctOfreactant_atomsDict: if (itemOftheReactntAtomsDictnry not in product_atoms) or (reactant_atoms[itemOftheReactntAtomsDictnry] != product_atoms[itemOftheReactntAtomsDictnry]): unbalancedAtomsSetObjct.add(itemOftheReactntAtomsDictnry) keysObjctOfproduct_atomsDict = product_atoms.keys() for itemOftheProductAtomsDictnry in keysObjctOfproduct_atomsDict: if (itemOftheProductAtomsDictnry not in reactant_atoms) or (product_atoms[itemOftheProductAtomsDictnry] != reactant_atoms[itemOftheProductAtomsDictnry]): unbalancedAtomsSetObjct.add(itemOftheProductAtomsDictnry) return unbalancedAtomsSetObjct ######################################################## # PART 4 - Check if a chemical equation represented by # two nested tuples is balanced ######################################################## def check_eqn_balance(reactants,products): """ (tuple,tuple) -> Set Check if a chemical equation is balanced. Return any unbalanced elements in a set. Both inputs are nested tuples. The first element of each tuple is a tuple containing the coefficients for molecules in the reactant or product expression. The second element is a tuple containing strings of the molecules within the reactant or product expression. The order of the coefficients corresponds to the order of the molecules. The function returns a set containing any elements that are unbalanced in the equation. For example, the following balanced equation C3H8 + 5O2 <-> 4H2O + 3CO2 would be input as the following two tuples: reactants: ((1,5), ("C3H8","O2")) products: ((4,3), ("H2O","CO2")) >>> check_eqn_balance(((1,5), ("C3H8","O2")),((4,3), ("H2O","CO2"))) set() Similarly for the unbalanced equation C3H8 + 2O2 <-> 4H2O + 3CO2 would be input as the following two tuples: reactants: ((1,2), ("C3H8","O2")) products: ((4,3), ("H2O","CO2")) >>> check_eqn_balance(((1,2), ("C3H8","O2")),((4,3), ("H2O","CO2"))) {'O'} """ #TODO your code here (NEED PART 4 ONLY)
# PART 1 - Complete the function below to deocompose
# a compound formula written as a string
# in a dictionary
######################################################
import chemparse
def mol_form(compound_formula):
"""(str) -> dictionary
When passed a string of the compound formula, returns a dictionary
with the elements as keys and the number of atoms of that element as values.
>>> mol_form("C2H6O")
{'C': 2, 'H': 6, 'O': 1}
>>> mol_form("CH4")
{'C': 1, 'H': 4}
"""
print((chemparse.parse_formula(compound_formula)))
mol_form("C2H6O")
mol_form("CH4")
######################################################
# PART 2 - Complete the function below that takes two
# tuples representing one side of a
# chemical equation and returns a dictionary
# with the elements as keys and the total
# number of atoms in the entire expression
# as values.
######################################################
def expr_form(expr_coeffs:tuple, expr_molecs: tuple) -> dict:
"""
(tuple (of ints), tuple (of dictionaries)) -> dictionary
For example, consider the expression 2NaCl + H2 + 5NaF
>>> expr_form((2,1,5), ({"Na":1, "Cl":1}, {"H":2}, {"Na":1, "F":1}))
{'Na': 7, 'Cl': 2, 'H': 2, 'F': 5}
"""
# TODO your code here
expr_Length = len(expr_molecs)
expr_result= {}
for i in range (expr_Length):
coefficient = expr_coeffs[i]
molecules = expr_molecs[i]
for item, num in molecules.items():
if item not in expr_result:
expr_result[item]= coefficient * num
else:
expr_result[item]+= coefficient * num
return expr_result
########################################################
# PART 3 - Check if two dictionaries representing
# the type and number of atoms on two sides of
# a chemical equation contain different
# key-value pairs
########################################################
def find_unbalanced_atoms(reactant_atoms, product_atoms):
"""
(Dict,Dict) -> Set
>>> find_unbalanced_atoms({"H" : 2, "Cl" : 2, "Na" : 2}, {"H" : 2, "Na" : 1, "Cl" : 2})
{'Na'}
>>> find_unbalanced_atoms({"H" : 2, "Cl" : 2, "Na" : 2}, {"H" : 2, "Na" : 2, "Cl" : 2})
set()
>>> find_unbalanced_atoms({"H" : 2, "Cl" : 2, "Na" : 2}, {"H" : 2, "F" : 2, "Cl" : 2})
{'F', 'Na'}
"""
# TODO your code here
unbalancedAtomsSetObjct = set()
keysObjctOfreactant_atomsDict = reactant_atoms.keys()
for itemOftheReactntAtomsDictnry in keysObjctOfreactant_atomsDict:
if (itemOftheReactntAtomsDictnry not in product_atoms) or (reactant_atoms[itemOftheReactntAtomsDictnry] != product_atoms[itemOftheReactntAtomsDictnry]):
unbalancedAtomsSetObjct.add(itemOftheReactntAtomsDictnry)
keysObjctOfproduct_atomsDict = product_atoms.keys()
for itemOftheProductAtomsDictnry in keysObjctOfproduct_atomsDict:
if (itemOftheProductAtomsDictnry not in reactant_atoms) or (product_atoms[itemOftheProductAtomsDictnry] != reactant_atoms[itemOftheProductAtomsDictnry]):
unbalancedAtomsSetObjct.add(itemOftheProductAtomsDictnry)
return unbalancedAtomsSetObjct
########################################################
# PART 4 - Check if a chemical equation represented by
# two nested tuples is balanced
########################################################
def check_eqn_balance(reactants,products):
"""
(tuple,tuple) -> Set
Check if a chemical equation is balanced. Return any unbalanced
elements in a set.
Both inputs are nested tuples. The first element of each tuple is a tuple
containing the coefficients for molecules in the reactant or product expression.
The second element is a tuple containing strings of the molecules within
the reactant or product expression. The order of the coefficients corresponds
to the order of the molecules. The function returns a set containing any
elements that are unbalanced in the equation.
For example, the following balanced equation
C3H8 + 5O2 <-> 4H2O + 3CO2
would be input as the following two tuples:
reactants: ((1,5), ("C3H8","O2"))
products: ((4,3), ("H2O","CO2"))
>>> check_eqn_balance(((1,5), ("C3H8","O2")),((4,3), ("H2O","CO2")))
set()
Similarly for the unbalanced equation
C3H8 + 2O2 <-> 4H2O + 3CO2
would be input as the following two tuples:
reactants: ((1,2), ("C3H8","O2"))
products: ((4,3), ("H2O","CO2"))
>>> check_eqn_balance(((1,2), ("C3H8","O2")),((4,3), ("H2O","CO2")))
{'O'}
"""
#TODO your code here
(NEED PART 4 ONLY)

Step by step
Solved in 4 steps with 3 images

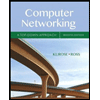
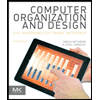
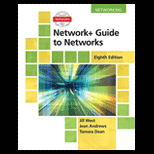
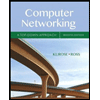
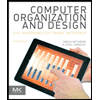
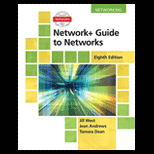
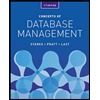
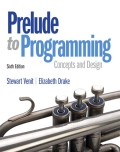
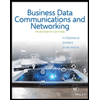