OBJECT ORIENTED IN JAVA Example for VERSION 1: Welcome to Nick's Neon Tubing Calculator Enter the shape type (R, S, C, T, L, Q): R Enter Height and Width of Rectangle: 6.5 12 The perimeter of a 6.5 x 12 rectangle is 37.0 Enter the shape type (R, S, C, T, L, Q): C Enter Diameter: 12 The circumference of circle with diameter 12 is 37.7 Enter the shape type (R, S, C, T, L, Q): Q Shapes Needed 37.0 - rectangle, 6.5 x 12 37.7 - circle, diameter 12 --------------------------- 74.7 Total Length Thank You there is a version 2 as well, if you look at the pictures. Every person I have asked to help has either not made it object oriented or that code did not work. PLEASE help.
OBJECT ORIENTED IN JAVA
Example for VERSION 1:
Welcome to Nick's Neon Tubing Calculator
Enter the shape type (R, S, C, T, L, Q):
R
Enter Height and Width of Rectangle:
6.5 12
The perimeter of a 6.5 x 12 rectangle is 37.0
Enter the shape type (R, S, C, T, L, Q):
C
Enter Diameter:
12
The circumference of circle with diameter 12 is 37.7
Enter the shape type (R, S, C, T, L, Q):
Q
Shapes Needed
37.0 - rectangle, 6.5 x 12
37.7 - circle, diameter 12
---------------------------
74.7 Total Length
Thank You
there is a version 2 as well, if you look at the pictures. Every person I have asked to help has either not made it object oriented or that code did not work. PLEASE help.
![Nick has just opened a sign shop, specializing in "Old School" Neon signs (bent glass tubes, inert gasses, the
real deal). Nick is trying to reduce how much glass tubing he uses (i.e. wastes through "trial and error") and has
asked you to write a Java program to figure out how much tubing to use for various sizes and shapes.
Design and code a Java program that prompts the user for the shapes and sizes, and calculates and prints the
"outside" dimension:
(R)ectangle given height and width
(S)quare given height
(C)ircumference of a circle, given the diameter.
(T)riangle perimeter
(L)ine given length [used for connecting shapes]
The program should continue to ask the user for values until the user enters 'Q' as the type of calculation.
After data entry, print the list of shapes and the Total Length.
The program should echo print the input data and prompt appropriately. Label the output and format to one
decimal place (omit units for now).](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fd9e43a20-7eff-4cd6-a8db-cbd57531164e%2F1f94755b-14b4-48a0-b52d-cd408eeed464%2Fp55jzci_processed.png&w=3840&q=75)


Algorithm:
- Start the program.
- Declare and initialize a Scanner object named "input" to read input from the console.
- Declare and initialize a double variable named "totalLength" to keep track of the total length of neon tubing required.
- Print a welcome message to the user.
- Enter a while loop that will continue until the user enters "Q" to quit the program.
- Inside the loop, prompt the user to enter the shape type they want to calculate the perimeter of (R, S, C, T, L, Q).
- Convert the input to upper case using the toUpperCase() method to avoid case sensitivity issues.
- Use a switch statement to execute different code based on the shape type entered by the user.
- For each case in the switch statement, prompt the user to enter the required parameters for the shape type and perform the appropriate calculation to find the perimeter of the shape. Also, add the perimeter to the total length variable.
- If the user enters an invalid shape type, print an error message.
- After the switch statement, use input.nextLine() to clear the input buffer and prepare for the next iteration of the loop.
- Once the user enters "Q" to quit the program, print out the total length of neon tubing required and a thank you message.
- End the program.
Step by step
Solved in 4 steps with 4 images

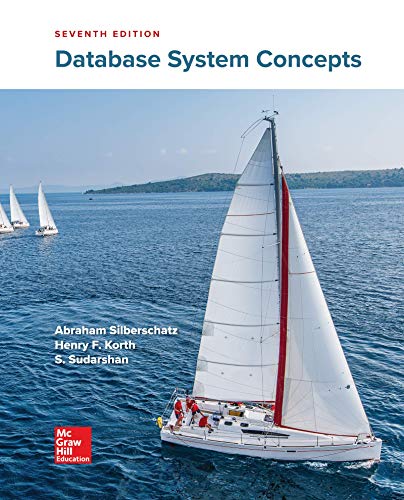
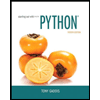
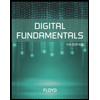
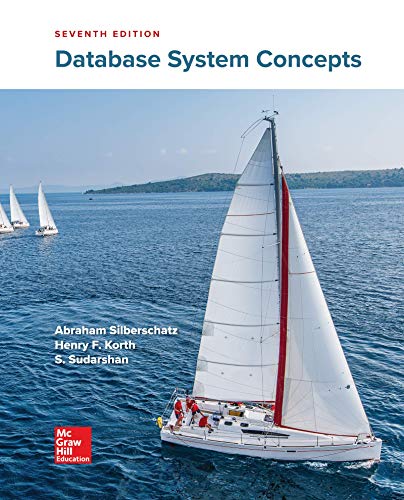
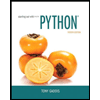
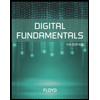
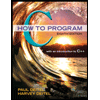
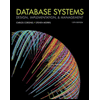
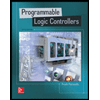