My code : # TODO: Write your header comment here. questions = [ "I am the life of the party E:1", "I warm up quickly to others A:1 E:1", "I never at a loss for words O:1 E:1", "I seldom get lost in thought C:1 O:-1", "I am easily distracted N:-1 C:-1" ] # Store the printed statement and answer options in string variables welcome_message = "Welcome to the Personality Quiz!" question_prompt = "Choose question number (1-5): " answer_prompt = "Enter your answer here (1-5): " # Function to extract the statement from a question string def extract_statement(question): return question.split(' ', 1)[1] # Print the welcome message print(welcome_message) print() # Get the question number from the user question_number = int(input(question_prompt)) # Validate the user input while question_number < 1 or question_number > 5: print("Invalid input. Please choose a question number between 1 and 5.") question_number = int(input(question_prompt)) # Get the selected question selected_question = questions[question_number - 1] # Extract the statement from the selected question question_statement = extract_statement(selected_question) # Print the statement with quotation marks and formatting print(f'Choose question number (1-5):\n') print(f'How much do you agree with this statement?\n"{question_statement}"\n') # Print answer options print("1. Strongly disagree") print("2. Disagree") print("3. Neutral") print("4. Agree") print("5. Strongly agree") # Get the user's answer user_answer = int(input(answer_prompt)) # Validate the user input while user_answer < 1 or user_answer > 5: print("Invalid input. Please enter a number between 1 and 5.") user_answer = int(input(answer_prompt)) # Print the user's answer print(f'\nYour answer is {user_answer}') ------------------------------------------------- Fix it and match it with expected output to pass the tests
My code : # TODO: Write your header comment here. questions = [ "I am the life of the party E:1", "I warm up quickly to others A:1 E:1", "I never at a loss for words O:1 E:1", "I seldom get lost in thought C:1 O:-1", "I am easily distracted N:-1 C:-1" ] # Store the printed statement and answer options in string variables welcome_message = "Welcome to the Personality Quiz!" question_prompt = "Choose question number (1-5): " answer_prompt = "Enter your answer here (1-5): " # Function to extract the statement from a question string def extract_statement(question): return question.split(' ', 1)[1] # Print the welcome message print(welcome_message) print() # Get the question number from the user question_number = int(input(question_prompt)) # Validate the user input while question_number < 1 or question_number > 5: print("Invalid input. Please choose a question number between 1 and 5.") question_number = int(input(question_prompt)) # Get the selected question selected_question = questions[question_number - 1] # Extract the statement from the selected question question_statement = extract_statement(selected_question) # Print the statement with quotation marks and formatting print(f'Choose question number (1-5):\n') print(f'How much do you agree with this statement?\n"{question_statement}"\n') # Print answer options print("1. Strongly disagree") print("2. Disagree") print("3. Neutral") print("4. Agree") print("5. Strongly agree") # Get the user's answer user_answer = int(input(answer_prompt)) # Validate the user input while user_answer < 1 or user_answer > 5: print("Invalid input. Please enter a number between 1 and 5.") user_answer = int(input(answer_prompt)) # Print the user's answer print(f'\nYour answer is {user_answer}') ------------------------------------------------- Fix it and match it with expected output to pass the tests
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
My code :
# TODO: Write your header comment here.
questions = [
"I am the life of the party E:1",
"I warm up quickly to others A:1 E:1",
"I never at a loss for words O:1 E:1",
"I seldom get lost in thought C:1 O:-1",
"I am easily distracted N:-1 C:-1"
]
# Store the printed statement and answer options in string variables
welcome_message = "Welcome to the Personality Quiz!"
question_prompt = "Choose question number (1-5): "
answer_prompt = "Enter your answer here (1-5): "
# Function to extract the statement from a question string
def extract_statement(question):
return question.split(' ', 1)[1]
# Print the welcome message
print(welcome_message)
print()
# Get the question number from the user
question_number = int(input(question_prompt))
# Validate the user input
while question_number < 1 or question_number > 5:
print("Invalid input. Please choose a question number between 1 and 5.")
question_number = int(input(question_prompt))
# Get the selected question
selected_question = questions[question_number - 1]
# Extract the statement from the selected question
question_statement = extract_statement(selected_question)
# Print the statement with quotation marks and formatting
print(f'Choose question number (1-5):\n')
print(f'How much do you agree with this statement?\n"{question_statement}"\n')
# Print answer options
print("1. Strongly disagree")
print("2. Disagree")
print("3. Neutral")
print("4. Agree")
print("5. Strongly agree")
# Get the user's answer
user_answer = int(input(answer_prompt))
# Validate the user input
while user_answer < 1 or user_answer > 5:
print("Invalid input. Please enter a number between 1 and 5.")
user_answer = int(input(answer_prompt))
# Print the user's answer
print(f'\nYour answer is {user_answer}')
-------------------------------------------------
Fix it and match it with expected output to pass the tests

Transcribed Image Text:Milestone #2 - Ask One Question
In a file called main.py, implement a program that asks the user one question and prints the answer to this question. The program should
have two input prompts: the integer value of the question number and the integer value of the user's answer on that question.
Your program should print the welcome message, ask for a question number, ask the question from the questions list, record the user's
answer into the list, and then print the answer. You are required to use the provided list with the questions. See example below.
Welcome to the Personality Quiz!
Choose question number (1-5): 5
How much do you agree with this statement?
"I am easily distracted"
1. Strongly disagree
2. Disagree
3. Neutral
4. Agree
5. Strongly agree
Enter your answer here (1-5): 1
Your answer is 1
Store the printed statement and the answers options in string variables for the next milestones.
Each question from the questions list consists of the statement and the OCEAN code. Use string parsing to get only the statement from the
question. You may assume that all statements end with a period. There are several ways to do this and you may find split and find
functions useful.
Run and Test Milestone #2
Develop your code incrementally and run often until it looks right. You should test on all files to make sure your function is written generally
and will work for all. When you feel confident your code is right, submit your code and test against the autograder. You have unlimited
attempts. See the test case below for the format of the output (e.g. hit "Submit for Grading" to see how the output is formatted).

Transcribed Image Text:Your Output
2.
3. Choose question number (1-5): Choose question numbe
4.
5. How much do you agree with this statement?
6.
"am easily distracted N:-1 C:-1"
7.
8.
1. Strongly disagree
9.
2. Disagree
10. 3. Neutral
11. 4. Agree
12. 5. Strongly agree
13. Enter your answer here (1-5):
14. Your answer is 1
2: Test 2
Input
4
2
Your Output
come to the Personality Quiz!
ose question number (1-5): Choose question number (1-5):
much do you agree with this statement?
Idom get lost in thought C:1 0:-1"
strongly disagree
Disagree
Neutral
Agree
Strongly agree
Output is nearly correct, but whitespace differs. See highlights above. Special character legend
er your answer here (1-5):
1. Welcome to the Personality Quiz!
2.
3.
4
Choose question number (1-5):
4.
5.
6.
7.
8.
9.
10. 4. Agree
11. 5. Strongly agree
12.
How much do you agree with this statement?
"I am easily distracted"
1. Strongly disagree
2. Disagree
3. Neutral
13. Enter your answer here (1-5):
14. Your answer is 1
1. Welcome to the Personality Quiz!
2.
3.
Choose question number (1-5):
4.
5.
6.
7.
1. Strongly disagree
8.
2. Disagree
9. 3. Neutral
How much do you agree with this statement?
"I seldom get lost in thought"
10. 4. Agree
11. 5. Strongly agree
12.
Expected output
13. Enter your answer here (1-5):
14. Your answer is 2
Output is nearly correct, but whitespace differs. See highlights above. Special character legend
0/3
Expected output
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
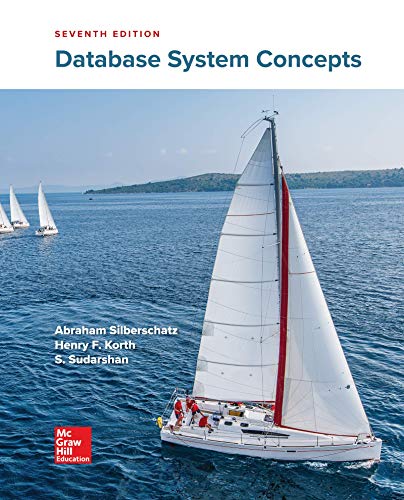
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
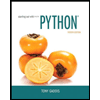
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
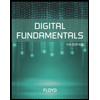
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
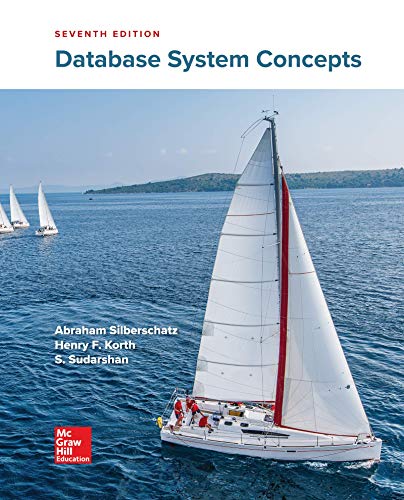
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
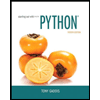
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
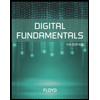
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
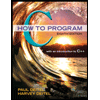
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
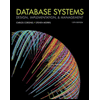
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
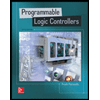
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education