INSTRUCTIONS: • You are to answer this activity individually. • You are to create a Python application that makes use of inputs, outputs, and sequence structures in Python. • It must meet all of the following requirements: • The application must create a list with exactly 10 items. The contents and type of list will be up to the student (e.g. Phone Brands, Types of Shoes, Shopping Apps). The application must first display the list after which the application must remove 5 random items from the list. The application must then show the updated list with 5 of the items removed. After this is done, the application must add 7 new items to the list. These items must be completely new and not the ones that were on the list initially. The application must then output the updated list with the added items. The application must then reverse the list and then display the updated list after it has been reversed.
INSTRUCTIONS: • You are to answer this activity individually. • You are to create a Python application that makes use of inputs, outputs, and sequence structures in Python. • It must meet all of the following requirements: • The application must create a list with exactly 10 items. The contents and type of list will be up to the student (e.g. Phone Brands, Types of Shoes, Shopping Apps). The application must first display the list after which the application must remove 5 random items from the list. The application must then show the updated list with 5 of the items removed. After this is done, the application must add 7 new items to the list. These items must be completely new and not the ones that were on the list initially. The application must then output the updated list with the added items. The application must then reverse the list and then display the updated list after it has been reversed.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
![INSTRUCTIONS:
• You are to answer this activity individually.
• You are to create a Python application that makes use of inputs, outputs, and sequence structures in Python.
• It must meet all of the following requirements:
• The application must create a list with exactly 10 items. The contents and type of list will be up to the student (e.g. Phone Brands, Types of Shoes, Shopping Apps). The application must first display the list
after which the application must remove 5 random items from the list. The application must then show the updated list with 5 of the items removed. After this is done, the application must add 7 new items to
the list. These items must be completely new and not the ones that were on the list initially. The application must then output the updated list with the added items. The application must then reverse the list
and then display the updated list after it has been reversed.
• The application must create a tuple with exactly 10 items. The contents and type of tuple will be up to the student (e.g. Laptop Brands, Types of Pants, Game Apps). The application must then display the
tuple that was created.
• The application must create a dictionary with exactly 5 items. The contents and type of dictionary will be up to the student (e.g. Lightbulb Brands and Uses, Types of Kitchen Utensil and Uses, Social Media
Apps and Social Media Types). The application must first display the dictionary after which the application must remove 2 random items from the dictionary. The application must then show the updated list
with 2 of the items removed. After this is done, the application must add 3 new items to the dictionary. These items must be completely new and not the ones that were on the dictionary initially. The
application must then output the updated dictionary with the added items.
• UPLOAD YOUR SOURCE CODE IN ".py" or ".ipynb" FORMATS ONLY.
Sample Output:
LIST: TYPES OF BREAD
['Baguettes', 'Bagels', 'Pita Bread', 'Rye Bread', 'Whole Wheat Bread', 'Sourdough Bread', 'Chapati Bread', 'Ciabatta Bread', 'Focaccia Bread', 'Multigrain Bread']
Removing 5 Items from the List...
['Baguettes', 'Bagels', 'Pita Bread', 'Rye Bread', 'Sourdough Bread']
Adding 7 New Items to the List...
['Baguettes', 'Bagels', 'Pita Bread', 'Rye Bread', 'Sourdough Bread', 'Arepa Bread', 'Grissini Bread', 'English Muffins Bread', 'Naan Bread', 'Paratha Bread', 'Roti Bread', 'Soda Bread']
Reversing the Contents of the List...
['Soda Bread', 'Roti Bread', 'Paratha Bread', 'Naan Bread', 'English Muffins Bread', 'Grissini Bread', 'Arepa Bread', 'Sourdough Bread', 'Rye Bread', 'Pita Bread', 'Bagels', 'Baguettes']
TUPLE: TYPES OF CARS
('Sedan', 'Coupe', 'Station Wagon', 'Hatchback', 'Convertible', 'Sport-Utility Vehicle(SUV)', 'Minivan', 'Pickup Truck', 'Sports Car', 'Race Car')
DICTIONARY: Social Media Companies and thier CEOS
{'Facebook": 'Mark Zuckerberg', 'Twitter': 'Jack Dorsey', 'Instagram': 'Kevin Systrom', 'Snapchat': 'Evan Spiegel', 'Reddit': 'Steve Huffman'}
Removing 2 Items from the Dictionary...
{'Facebook': 'Mark Zuckerberg', 'Twitter': 'Jack Dorsey', 'Instagram': 'Kevin Systrom'}
Adding 3 Items to the Dictionary...
{'Facebook': 'Mark Zuckerberg', 'Twitter': 'Jack Dorsey', 'Instagram': 'Kevin Systrom', 'Tumblr': "Jeff D'Onofrio", 'Myspace': 'Tim Vanderhook', 'TikTok': 'Shou Zi Chew'}](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F70cf2dee-41a1-45ed-ab83-91338f1d7d3a%2Fe41b611e-700d-4c85-af1c-aaeb8b29ddeb%2Fofi6ion_processed.png&w=3840&q=75)
Transcribed Image Text:INSTRUCTIONS:
• You are to answer this activity individually.
• You are to create a Python application that makes use of inputs, outputs, and sequence structures in Python.
• It must meet all of the following requirements:
• The application must create a list with exactly 10 items. The contents and type of list will be up to the student (e.g. Phone Brands, Types of Shoes, Shopping Apps). The application must first display the list
after which the application must remove 5 random items from the list. The application must then show the updated list with 5 of the items removed. After this is done, the application must add 7 new items to
the list. These items must be completely new and not the ones that were on the list initially. The application must then output the updated list with the added items. The application must then reverse the list
and then display the updated list after it has been reversed.
• The application must create a tuple with exactly 10 items. The contents and type of tuple will be up to the student (e.g. Laptop Brands, Types of Pants, Game Apps). The application must then display the
tuple that was created.
• The application must create a dictionary with exactly 5 items. The contents and type of dictionary will be up to the student (e.g. Lightbulb Brands and Uses, Types of Kitchen Utensil and Uses, Social Media
Apps and Social Media Types). The application must first display the dictionary after which the application must remove 2 random items from the dictionary. The application must then show the updated list
with 2 of the items removed. After this is done, the application must add 3 new items to the dictionary. These items must be completely new and not the ones that were on the dictionary initially. The
application must then output the updated dictionary with the added items.
• UPLOAD YOUR SOURCE CODE IN ".py" or ".ipynb" FORMATS ONLY.
Sample Output:
LIST: TYPES OF BREAD
['Baguettes', 'Bagels', 'Pita Bread', 'Rye Bread', 'Whole Wheat Bread', 'Sourdough Bread', 'Chapati Bread', 'Ciabatta Bread', 'Focaccia Bread', 'Multigrain Bread']
Removing 5 Items from the List...
['Baguettes', 'Bagels', 'Pita Bread', 'Rye Bread', 'Sourdough Bread']
Adding 7 New Items to the List...
['Baguettes', 'Bagels', 'Pita Bread', 'Rye Bread', 'Sourdough Bread', 'Arepa Bread', 'Grissini Bread', 'English Muffins Bread', 'Naan Bread', 'Paratha Bread', 'Roti Bread', 'Soda Bread']
Reversing the Contents of the List...
['Soda Bread', 'Roti Bread', 'Paratha Bread', 'Naan Bread', 'English Muffins Bread', 'Grissini Bread', 'Arepa Bread', 'Sourdough Bread', 'Rye Bread', 'Pita Bread', 'Bagels', 'Baguettes']
TUPLE: TYPES OF CARS
('Sedan', 'Coupe', 'Station Wagon', 'Hatchback', 'Convertible', 'Sport-Utility Vehicle(SUV)', 'Minivan', 'Pickup Truck', 'Sports Car', 'Race Car')
DICTIONARY: Social Media Companies and thier CEOS
{'Facebook": 'Mark Zuckerberg', 'Twitter': 'Jack Dorsey', 'Instagram': 'Kevin Systrom', 'Snapchat': 'Evan Spiegel', 'Reddit': 'Steve Huffman'}
Removing 2 Items from the Dictionary...
{'Facebook': 'Mark Zuckerberg', 'Twitter': 'Jack Dorsey', 'Instagram': 'Kevin Systrom'}
Adding 3 Items to the Dictionary...
{'Facebook': 'Mark Zuckerberg', 'Twitter': 'Jack Dorsey', 'Instagram': 'Kevin Systrom', 'Tumblr': "Jeff D'Onofrio", 'Myspace': 'Tim Vanderhook', 'TikTok': 'Shou Zi Chew'}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 2 images

Recommended textbooks for you
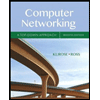
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
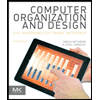
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
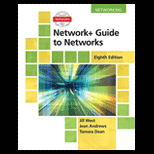
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
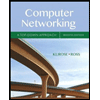
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
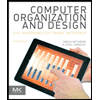
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
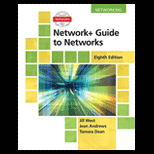
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
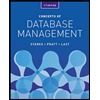
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
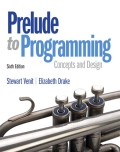
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
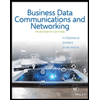
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY