Inside the FlightController class, you will define an object of type FlightSchedule which will contain an array of 3 objects of type Flight. In your Flight objects, the constructor of these objects will ask from the user for the inputs of: flight name, launch hour, launch minutes, flight duration. The value of state of the Flight object will be assigned to “Idle” as a default value in the constructor also and you will call the member function within the Flight class which will calculate the landing time for your flight object by using the duration and the launch time. Definition of Flight class will also contain the necessary getters and setters that are given in the diagram and needed throughout your program. In the constructor of your FlightController you will need to define a pointer that will point to the adress of the FlightSchedule object you have created. You will send that pointer into the functions launchSchedule and landingSchedule in your base classes and these functions will perform a sorting operation between these three flight objects. The launchController will check the launch times as in hours and minutes of the array of objects that you have passed as a reference and will sort the array in ascending order. After the sorting you will output the launch order of these flights in order. Similar approach will be taken in the landingController and the landing order will be displayed as output after the sorting is complete. Outputs will consist of flight name, landing hour, landing minute, flight’s state and they will be printed from top down in order. Same thing will hold for both of the landing and launch controllers. ________________________________________________________________________________ //this is my code for this hw but i confused for the arrays and inheritence classes please help #include using namespace std; class flight{ private: string flight_name; int launch_hour,launch_min; int duration; int land_hour; int land_min; int time; public: flight(){ cout<<"Enter the name of the flight:"; cin>>flight_name; cout<<"Enter the departure time of the plane:"; cin>>launch_hour>>launch_min; cout<<"Enter the duration of the flight in minutes:"; cin>>duration; cout<
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Inside the FlightController class, you will define an object of type FlightSchedule which will
contain an array of 3 objects of type Flight. In your Flight objects, the constructor of these
objects will ask from the user for the inputs of: flight name, launch hour, launch minutes,
flight duration. The value of state of the Flight object will be assigned to “Idle” as a default
value in the constructor also and you will call the member function within the Flight class
which will calculate the landing time for your flight object by using the duration and the
launch time. Definition of Flight class will also contain the necessary getters and setters that
are given in the diagram and needed throughout your
In the constructor of your FlightController you will need to define a pointer that will point to
the adress of the FlightSchedule object you have created. You will send that pointer into the
functions launchSchedule and landingSchedule in your base classes and these functions will
perform a sorting operation between these three flight objects.
The launchController will check the launch times as in hours and minutes of the array of
objects that you have passed as a reference and will sort the array in ascending order. After
the sorting you will output the launch order of these flights in order. Similar approach will be
taken in the landingController and the landing order will be displayed as output after the
sorting is complete. Outputs will consist of flight name, landing hour, landing minute, flight’s
state and they will be printed from top down in order. Same thing will hold for both of the
landing and launch controllers.
________________________________________________________________________________
//this is my code for this hw but i confused for the arrays and inheritence classes please help
#include<iostream>
using namespace std;
class flight{
private:
string flight_name;
int launch_hour,launch_min;
int duration;
int land_hour;
int land_min;
int time;
public:
flight(){
cout<<"Enter the name of the flight:";
cin>>flight_name;
cout<<"Enter the departure time of the plane:";
cin>>launch_hour>>launch_min;
cout<<"Enter the duration of the flight in minutes:";
cin>>duration;
cout<<endl<<endl;
}
int calculate1(){
launch_hour*=60;
time=launch_hour+launch_min;//time=610
land_hour=time/60;//land hour=10
land_min=time-land_hour*60;
launch_hour/=60;
return time;
}
int calculate(){
launch_hour*=60;
time=duration+launch_hour+launch_min;//time=610
land_hour=time/60;//land hour=10
land_min=time-land_hour*60;
launch_hour/=60;
return time;
}
void print_launch(){
cout<<flight_name<<" Launch at "<<launch_hour<<" : "<<launch_min<<" Flight State: IDLE"<<endl;
}
void print_land(){
cout<<flight_name<<" Landing at "<<land_hour<<" : "<<land_min<<" Flight State: IDLE"<<endl;
}
};
void check(flight f1,flight f2,flight f3){
cout<<"Launch Order"<<endl;
if(f1.calculate1()<=f3.calculate1()&&f1.calculate1()<=f2.calculate1()&&f2.calculate1()<f3.calculate1()){
f1.print_launch();
f2.print_launch();
f3.print_launch();
}
else if(f2.calculate1()<=f3.calculate1()&&f2.calculate1()<=f1.calculate1()&&f1.calculate1()<f3.calculate1()){
f2.print_launch();
f1.print_launch();
f3.print_launch();
}
else if(f3.calculate1()<=f2.calculate1()&&f3.calculate1()<=f1.calculate1()&&f1.calculate1()<f2.calculate1()){
f3.print_launch();
f1.print_launch();
f2.print_launch();
}
else if(f3.calculate1()<=f2.calculate1()&&f3.calculate1()<=f1.calculate1()&&f2.calculate1()<f1.calculate1()){
f3.print_launch();
f2.print_launch();
f1.print_launch();
}
else if(f2.calculate1()<=f3.calculate1()&&f2.calculate1()<=f1.calculate1()&&f3.calculate1()<f1.calculate1()){
f2.print_launch();
f3.print_launch();
f1.print_launch();
}
else if(f1.calculate1()<=f3.calculate1()&&f1.calculate1()<=f2.calculate1()&&f3.calculate1()<f2.calculate1()){
f1.print_launch();
f3.print_launch();
f2.print_launch();
}
cout<<"\n"<<"Landing Order"<<endl;
if(f1.calculate()<=f3.calculate()&&f1.calculate()<=f2.calculate()&&f2.calculate()<f3.calculate()){
f1.print_land();
f2.print_land();
f3.print_land();
}
else if(f1.calculate()<=f3.calculate()&&f1.calculate()<=f2.calculate()&&f3.calculate()<f2.calculate()){
f1.print_land();
f3.print_land();
f2.print_land();
}
else if(f2.calculate()<=f3.calculate()&&f2.calculate()<=f1.calculate()&&f3.calculate()<f1.calculate()){
f2.print_land();
f3.print_land();
f1.print_land();
}
else if(f2.calculate()<=f3.calculate()&&f2.calculate()<=f1.calculate()&&f1.calculate()<f3.calculate()){
f2.print_land();
f1.print_land();
f3.print_land();
}
else if(f3.calculate()<=f2.calculate()&&f3.calculate()<=f1.calculate()&&f1.calculate()<f2.calculate()){
f3.print_land();
f1.print_land();
f2.print_land();
}
else if(f3.calculate()<=f2.calculate()&&f3.calculate()<=f1.calculate()&&f2.calculate()<f1.calculate()){
f3.print_land();
f2.print_land();
f1.print_land();
}
}
int main(){
flight *f1[]={new flight,new flight,new flight};
f1.calculate();
flight f2;
f2.calculate();
flight f3;
f3.calculate();
check(f1,f2,f3);
system("PAUSE");
}

![cmpe225_hw1 (1).pdf
2 / 6
135%
+
Assignment
Write a program that will track certain information about the flights of aircrafts. You
will create your program by referencing the structure given in the diagram (1). Class
definitions, access specifiers, data members and member functions in your program must be
coherent with the diagram. Certain essential variables for operations such as loops, flag
values, counts etc. are not given in the diagram therefore you can define them as your own
extra variables in the program.
LaunchController
LandingController
-launchHour1: int
- launchHour2: int
- launchMin1: int
landHour1: int
- landHour2: int
- landMin1: int
- launchMin2: int
landMin2: int
+ launchSchedule(FlightSchedule" ): void
+ landingSchedule(FlightSchedule" ): void
Extends
Extends
FlightController
# flight_schedule: FlightSchedule
+ FlightController()
+ flights: FlightSchedule"
Flight
|- flightName: string
|- launchHour: int
- launchMin: int
-landHour: int
-landMin: int
FlightSchedule
duration: int
- flights[]: Flight
- state: string
+ method(type): type
+ getlaunchHour(): int
+ getLaunchMin(): int
+ getlandHour(): int
+ getLandMin(): int
+ getState(): string
+ setState(string): void
+ calculatelanding(): void](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F15a145e8-7f8f-437c-aa3d-ad03340b8212%2Ffa87e435-546a-4d76-bb46-f25021879877%2Fecyrv1q_processed.png&w=3840&q=75)

Step by step
Solved in 5 steps with 4 images

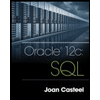
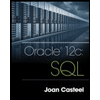