in C for STM32F446RE microcontroller Write a source library that contains the with the following public functions: void keypadInit(void); /Initiallized the GPIO to read the keypad. uint16_t readKeypad(void); //Returns the state of all of the keypad buttons in the return value at the moment the function is called. void decodeKeypad(uint16_t, char *); //Takes the state of the keypad and returns (by reference) an array of the key's pressed. The library should work with the following main: int main (void) { uint16_t key; char carray[17]; keypadInit(); while(1) { while(!(key = readKeypad())); /*Get which keys pressed*/ decodeKeypad(key, carray); /*What are those keys*/ printf("%s\n",carray); /*Print those keys to screen*/ while(readKeypad() == key); /*Wait for the keypad to change*/ } } Problem 1: Write a library that works with the following pin assignments R0 -> PC0 R1 -> PC2 R2-> PC4 R3 -> PC6 C0-> PC8 C1->PC10 C2->PC12
in C for STM32F446RE microcontroller
{
uint16_t key;
char carray[17];
keypadInit();
while(1)
{
while(!(key = readKeypad())); /*Get which keys pressed*/
decodeKeypad(key, carray); /*What are those keys*/
printf("%s\n",carray); /*Print those keys to screen*/
while(readKeypad() == key); /*Wait for the keypad to change*/
}
}

Here's a possible implementation of the requested library in C for the STM32F446RE microcontroller:
CODE in C:
#include "stm32f4xx.h"
#include "keypad.h"
#define KEYPAD_COLS 3
#define KEYPAD_ROWS 4
GPIO_TypeDef* KEYPAD_GPIO_PORT = GPIOC;
const uint16_t KEYPAD_GPIO_PINS[KEYPAD_ROWS + KEYPAD_COLS] = {
GPIO_PIN_0, GPIO_PIN_2, GPIO_PIN_4, GPIO_PIN_6, GPIO_PIN_8, GPIO_PIN_10, GPIO_PIN_12
};
const uint8_t KEYPAD_ROW_PINS[KEYPAD_ROWS] = {0, 1, 2, 3};
const uint8_t KEYPAD_COL_PINS[KEYPAD_COLS] = {4, 5, 6};
const uint8_t KEYPAD_KEYMAP[KEYPAD_ROWS][KEYPAD_COLS] = {
{'1', '2', '3'},
{'4', '5', '6'},
{'7', '8', '9'},
{'*', '0', '#'}
};
void keypadInit(void) {
RCC->AHB1ENR |= RCC_AHB1ENR_GPIOCEN; // enable GPIOC clock
// configure GPIO pins as inputs with pull-up resistors
GPIO_InitTypeDef GPIO_InitStruct;
GPIO_InitStruct.Mode = GPIO_MODE_INPUT;
GPIO_InitStruct.Pull = GPIO_PULLUP;
for (int i = 0; i < KEYPAD_ROWS + KEYPAD_COLS; i++) {
GPIO_InitStruct.Pin = KEYPAD_GPIO_PINS[i];
HAL_GPIO_Init(KEYPAD_GPIO_PORT, &GPIO_InitStruct);
}
}
uint16_t readKeypad(void) {
uint16_t state = 0;
for (int i = 0; i < KEYPAD_COLS; i++) {
// set column pin to low
HAL_GPIO_WritePin(KEYPAD_GPIO_PORT, KEYPAD_GPIO_PINS[KEYPAD_ROWS + i], GPIO_PIN_RESET);
// read row pins and update state
for (int j = 0; j < KEYPAD_ROWS; j++) {
uint8_t pin = KEYPAD_GPIO_PINS[j];
if (!HAL_GPIO_ReadPin(KEYPAD_GPIO_PORT, pin)) {
state |= 1 << (j * KEYPAD_COLS + i);
}
}
// set column pin back to high
HAL_GPIO_WritePin(KEYPAD_GPIO_PORT, KEYPAD_GPIO_PINS[KEYPAD_ROWS + i], GPIO_PIN_SET);
}
return state;
}
void decodeKeypad(uint16_t state, char *keys) {
for (int i = 0; i < KEYPAD_ROWS; i++) {
for (int j = 0; j < KEYPAD_COLS; j++) {
if (state & (1 << (i * KEYPAD_COLS + j))) {
*keys++ = KEYPAD_KEYMAP[i][j];
}
}
}
*keys = '\0';
}
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

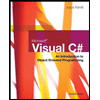
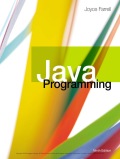
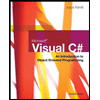
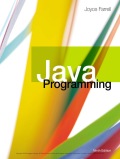