I'm seeking assistance in detecting all possible syntax and logical errors for these 4 pieces of python pseudocode. (1) // This pseudocode is intended to determine whether students have // passed or failed a course; student needs to average 60 or // more on two tests. start Declarations num firstTest num secondTest num average num PASSING = 60 while firstTest not equal to 0 output "Enter first score or 0 to quit " input firstTest output "Enter second score" input secondTest average = (firstTest + secondTest) / 2 ouput "Average is ", average if average >= PASSING then output "Pass" else output "Fail" endif endwhile stop (2) // This pseudocode is intended to display employee net pay values. // All employees have a standard $45 deduction from their checks. // If an employee does not earn enough to cover the deduction, // an error message is displayed. start Declarations string name num hours num rate string DEDUCTION = 45 string EOFNAME = "ZZZ" num gross num net output "Enter first name or ", EOFNAME, " to quit" input name if name not equal to EOFNAME output "Enter hours worked for ", name input hours output "Enter hourly rate for ", name input rate gross = hours * rate net = gross - DEDUCTION while net > 0 then output "Net pay for ", name, " is ", net else output "Deductions not covered. Net is 0." endwhile output "Enter next name or ", EOFNAME, " to quit" input name endif output "End of job" stop
Operations
In mathematics and computer science, an operation is an event that is carried out to satisfy a given task. Basic operations of a computer system are input, processing, output, storage, and control.
Basic Operators
An operator is a symbol that indicates an operation to be performed. We are familiar with operators in mathematics; operators used in computer programming are—in many ways—similar to mathematical operators.
Division Operator
We all learnt about division—and the division operator—in school. You probably know of both these symbols as representing division:
Modulus Operator
Modulus can be represented either as (mod or modulo) in computing operation. Modulus comes under arithmetic operations. Any number or variable which produces absolute value is modulus functionality. Magnitude of any function is totally changed by modulo operator as it changes even negative value to positive.
Operators
In the realm of programming, operators refer to the symbols that perform some function. They are tasked with instructing the compiler on the type of action that needs to be performed on the values passed as operands. Operators can be used in mathematical formulas and equations. In programming languages like Python, C, and Java, a variety of operators are defined.
I'm seeking assistance in detecting all possible syntax and logical errors for these 4 pieces of python pseudocode.
(1)
// This pseudocode is intended to determine whether students have
// passed or failed a course; student needs to average 60 or
// more on two tests.
start
Declarations
num firstTest
num secondTest
num average
num PASSING = 60
while firstTest not equal to 0
output "Enter first score or 0 to quit "
input firstTest
output "Enter second score"
input secondTest
average = (firstTest + secondTest) / 2
ouput "Average is ", average
if average >= PASSING then
output "Pass"
else
output "Fail"
endif
endwhile
stop
(2)
// This pseudocode is intended to display employee net pay values.
// All employees have a standard $45 deduction from their checks.
// If an employee does not earn enough to cover the deduction,
// an error message is displayed.
start
Declarations
string name
num hours
num rate
string DEDUCTION = 45
string EOFNAME = "ZZZ"
num gross
num net
output "Enter first name or ", EOFNAME, " to quit"
input name
if name not equal to EOFNAME
output "Enter hours worked for ", name
input hours
output "Enter hourly rate for ", name
input rate
gross = hours * rate
net = gross - DEDUCTION
while net > 0 then
output "Net pay for ", name, " is ", net
else
output "Deductions not covered. Net is 0."
endwhile
output "Enter next name or ", EOFNAME, " to quit"
input name
endif
output "End of job"
stop
(3)
// This pseudocode is intended to display
// employee net pay values. All employees have a standard
// $45 deduction from their checks.
// If an employee does not earn enough to cover the deduction,
// an error message is displayed.
// This example is modularized.
start
Declarations
string name
string EOFNAME = ZZZZ
while name not equal to EOFNAME
housekeeping()
endwhile
while name not equal to EOFNAME
mainLoop()
endwhile
while name not equal to EOFNAME
finish()
endwhile
stop
housekeeping()
output "Enter first name or ", EOFNAME, " to quit "
return
mainLoop()
Declarations
num hours
num rate
num DEDUCTION = 45
num net
output "Enter hours worked for ", name
input hours
output "Enter hourly rate for ", name
input rate
gross = hours * rate
net = gross - DEDUCTION
if net > 0 then
output "Net pay for ", name, " is ", net
else
output "Deductions not covered. Net is 0."
endif
output "Enter next name or ", EOFNAME, " to quit "
input name
return
finish()
output "End of job"
return
(4)
Image attached


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

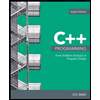
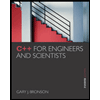
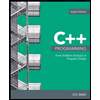
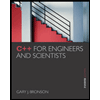
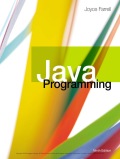