I have the following code: public class Length { private int feet; private int inches; // Your code goes here Length() { feet = inches = 0; } Length(int newFeet, int newInches) { feet = newFeet; inches = newInches; } public int getFeet() { return feet; } public void setFeet(int newFeet) { feet = newFeet; } public int getInches() { return inches; } public void setInches(int newInches) { inches = newInches; } public Length add(Length otherLength) { int newFeet = feet + otherLength.feet; int newInches = inches + otherLength.inches; if(newInches >= 12) { newFeet++; newInches -= 12; } return (new Length(newFeet,newInches)); } public Length subtract(Length otherLength) { if(this.feet > otherLength.feet) { int newFeet = feet - otherLength.feet; int newInches = inches - otherLength.inches; if(newInches < 0) { newFeet--; newInches += 12; } return (new Length(newFeet,newInches)); } else { int newFeet = otherLength.feet - feet; int newInches = otherLength.inches - inches; if(newInches < 0) { newFeet--; newInches += 12; } return (new Length(newFeet,newInches)); } } public boolean equals(Length otherLength) { if(feet == otherLength.feet && inches == otherLength.inches) return true; else return false; } public String toString() { return ("["+feet + "' " + inches + "\""+"]"); } } _____________________________________________________ import java.util.Random; public class TestLength { public static void main(String[] args) { Random rand = new Random(10); Length length[] = new Length[10]; // fill length array and print for (int i = 0; i < length.length; i++) { length[i] = new Length(rand.nextInt(11) + 10, rand.nextInt(12)); System.out.print(length[i].toString() + " "); } System.out.println(); System.out.println(); // Print sum of the Length objects Length sum = sum(length); System.out.println("Sum is: "+sum); System.out.println(); // use sort method and print System.out.println("Sorted List"); selectionSort(length); for (int i = 0; i < length.length; i++) { System.out.print(length[i].toString() + " "); } System.out.println(); } public static void selectionSort(Length[] arr) { // Your code goes here int n = arr.length; for (int i = 0; i < n-1; i++) { int min = i; for (int j = i+1; j < n; j++) { if (arr[j].getFeet() < arr[min].getFeet()) min = j; } Length temp = new Length(); temp = arr[min]; arr[min] = arr[i]; arr[i] = temp; } } public static Length sum(Length[] list) { // Your code goes here int f = 0, inch = 0; for(int i = 0 ; i < list.length ; i++) { f = f + list[i].getFeet(); inch = inch + list[i].getInches(); } return (new Length(f,inch)); } } ________________________________________________ But my output is [15' 0"] [20' 6"] [17' 4"] [16' 4"] [17' 10"] [16' 11"] [13' 0"] [10' 0"] [13' 1"] [20' 10"] Sum is: [157' 46"] Sorted List [10' 0"] [13' 0"] [13' 1"] [15' 0"] [16' 11"] [16' 4"] [17' 10"] [17' 4"] [20' 6"] [20' 10"] And what it's supposed to be is: [15' 0"] [20' 6"] [17' 4"] [16' 4"] [17' 10"] [16' 11"] [13' 0"] [10' 0"] [13' 1"] [20' 10"] Sum is: [160' 10"] Sorted List [10' 0"] [13' 0"] [13' 1"] [15' 0"] [16' 4"] [16' 11"] [17' 4"] [17' 10"] [20' 6"] [20' 10"]
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
I have the following code:
public class Length
{
private int feet;
private int inches;
// Your code goes here
Length()
{
feet = inches = 0;
}
Length(int newFeet, int newInches)
{
feet = newFeet;
inches = newInches;
}
public int getFeet() { return feet; }
public void setFeet(int newFeet) { feet = newFeet; }
public int getInches() { return inches; }
public void setInches(int newInches) { inches = newInches; }
public Length add(Length otherLength)
{
int newFeet = feet + otherLength.feet;
int newInches = inches + otherLength.inches;
if(newInches >= 12)
{
newFeet++;
newInches -= 12;
}
return (new Length(newFeet,newInches));
}
public Length subtract(Length otherLength)
{
if(this.feet > otherLength.feet)
{
int newFeet = feet - otherLength.feet;
int newInches = inches - otherLength.inches;
if(newInches < 0)
{
newFeet--;
newInches += 12;
}
return (new Length(newFeet,newInches));
}
else
{
int newFeet = otherLength.feet - feet;
int newInches = otherLength.inches - inches;
if(newInches < 0)
{
newFeet--;
newInches += 12;
}
return (new Length(newFeet,newInches));
}
}
public boolean equals(Length otherLength)
{
if(feet == otherLength.feet && inches == otherLength.inches)
return true;
else
return false;
}
public String toString()
{
return ("["+feet + "' " + inches + "\""+"]");
}
}
_____________________________________________________
import java.util.Random;
public class TestLength
{
public static void main(String[] args)
{
Random rand = new Random(10);
Length length[] = new Length[10];
// fill length array and print
for (int i = 0; i < length.length; i++)
{
length[i] = new Length(rand.nextInt(11) + 10, rand.nextInt(12));
System.out.print(length[i].toString() + " ");
}
System.out.println();
System.out.println();
// Print sum of the Length objects
Length sum = sum(length);
System.out.println("Sum is: "+sum);
System.out.println();
// use sort method and print
System.out.println("Sorted List");
selectionSort(length);
for (int i = 0; i < length.length; i++)
{
System.out.print(length[i].toString() + " ");
}
System.out.println();
}
public static void selectionSort(Length[] arr)
{
// Your code goes here
int n = arr.length;
for (int i = 0; i < n-1; i++)
{
int min = i;
for (int j = i+1; j < n; j++)
{
if (arr[j].getFeet() < arr[min].getFeet())
min = j;
}
Length temp = new Length();
temp = arr[min];
arr[min] = arr[i];
arr[i] = temp;
}
}
public static Length sum(Length[] list)
{
// Your code goes here
int f = 0, inch = 0;
for(int i = 0 ; i < list.length ; i++)
{
f = f + list[i].getFeet();
inch = inch + list[i].getInches();
}
return (new Length(f,inch));
}
}
________________________________________________
But my output is
[15' 0"] [20' 6"] [17' 4"] [16' 4"] [17' 10"] [16' 11"] [13' 0"] [10' 0"] [13' 1"] [20' 10"] Sum is: [157' 46"] Sorted List [10' 0"] [13' 0"] [13' 1"] [15' 0"] [16' 11"] [16' 4"] [17' 10"] [17' 4"] [20' 6"] [20' 10"]
And what it's supposed to be is:
[15' 0"] [20' 6"] [17' 4"] [16' 4"] [17' 10"] [16' 11"] [13' 0"] [10' 0"] [13' 1"] [20' 10"] Sum is: [160' 10"] Sorted List [10' 0"] [13' 0"] [13' 1"] [15' 0"] [16' 4"] [16' 11"] [17' 4"] [17' 10"] [20' 6"] [20' 10"]

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 5 images

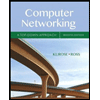
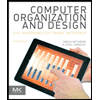
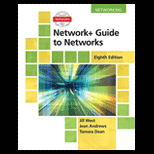
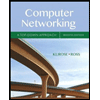
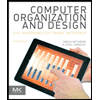
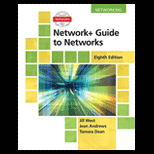
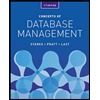
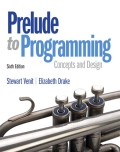
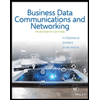