I have provided the code I have written. All I need you to do is to rewrite the code. I need you to rewrite the comments, rename all the variables, and shift the code. It should look different once you have edited it. I would like to see another format for this code. It is in C-Programming. I think it shouldn’t take so long. Take your time, please! I really appreciate any help you can provide! CODE STARTS HERE: #include #include struct node { int key; struct node *left, *right; }; // A utility function to create a new BST node struct node *newNode(int item) { struct node *temp = (struct node *)malloc(sizeof(struct node)); temp->key = item; temp->left = temp->right = NULL; return temp; } // A utility function to do inorder traversal of BST void inorder(struct node *root) { if (root != NULL) { printf("("); inorder(root->left); printf("%d", root->key); inorder(root->right); printf(")"); } } struct node* search(struct node* root, int key) { // Base Cases: root is null or key is present at root if (root == NULL || root->key == key) return root; // Key is greater than root's key if (root->key < key) return search(root->right, key); // Key is smaller than root's key return search(root->left, key); } /* A utility function to insert a new node with given key in BST */ struct node* insert(struct node* node, int key) { /* If the tree is empty, return a new node */ if (node == NULL) { printf("inserted\n"); return newNode(key); } /* Otherwise, recur down the tree */ if (key < node->key) node->left = insert(node->left, key); else if (key > node->key) node->right = insert(node->right, key); else { printf("duplicate\n"); } /* return the (unchanged) node pointer */ return node; } struct node * minValueNode(struct node* node) { struct node* current = node; /* loop down to find the leftmost leaf */ while (current->left != NULL) current = current->left; return current; } /* Given a binary search tree and a key, this function deletes the key and returns the new root */ struct node* deleteNode(struct node* root, int key) { // base case if (root == NULL) return root; // If the key to be deleted is smaller than the root's key, // then it lies in left subtree if (key < root->key) root->left = deleteNode(root->left, key); // If the key to be deleted is greater than the root's key, // then it lies in right subtree else if (key > root->key) root->right = deleteNode(root->right, key); // if key is same as root's key, then This is the node // to be deleted else { // node with only one child or no child if (root->left == NULL) { struct node *temp = root->right; free(root); return temp; } else if (root->right == NULL) { struct node *temp = root->left; free(root); return temp; } // node with two children: Get the inorder successor (smallest // in the right subtree) struct node* temp = minValueNode(root->right); // Copy the inorder successor's content to this node root->key = temp->key; // Delete the inorder successor root->right = deleteNode(root->right, temp->key); } return root; } int isvalid(char command) { if(command=='i' || command == 'd' || command == 's' || command == 'p') return 1; else return 0; } int main() { char command; int n; struct node *root = NULL; do { scanf("%c",&command); if(command=='i') { scanf("%d",&n); root = insert(root,n); } else if(command=='d') { scanf("%d",&n); root = deleteNode(root,n); } else if(command=='s') { scanf("%d",&n); if(search(root,n)) printf("present\n"); else printf("absent\n"); } else if(command=='p') { inorder(root); printf("\n"); } else command = 'q'; // flushes the standard input // (clears the input buffer) while ((getchar()) != '\n'); }while(isvalid(command)); return 0; }
I have provided the code I have written. All I need you to do is to rewrite the code. I need you to rewrite the comments, rename all the variables, and shift the code. It should look different once you have edited it. I would like to see another format for this code. It is in C-Programming. I think it shouldn’t take so long. Take your time, please! I really appreciate any help you can provide!
CODE STARTS HERE:
#include<stdio.h>
#include<stdlib.h>
struct node
{
int key;
struct node *left, *right;
};
// A utility function to create a new BST node
struct node *newNode(int item)
{
struct node *temp = (struct node *)malloc(sizeof(struct node));
temp->key = item;
temp->left = temp->right = NULL;
return temp;
}
// A utility function to do inorder traversal of BST
void inorder(struct node *root)
{
if (root != NULL)
{
printf("(");
inorder(root->left);
printf("%d", root->key);
inorder(root->right);
printf(")");
}
}
struct node* search(struct node* root, int key)
{
// Base Cases: root is null or key is present at root
if (root == NULL || root->key == key)
return root;
// Key is greater than root's key
if (root->key < key)
return search(root->right, key);
// Key is smaller than root's key
return search(root->left, key);
}
/* A utility function to insert a new node with given key in BST */
struct node* insert(struct node* node, int key)
{
/* If the tree is empty, return a new node */
if (node == NULL) {
printf("inserted\n");
return newNode(key);
}
/* Otherwise, recur down the tree */
if (key < node->key)
node->left = insert(node->left, key);
else if (key > node->key)
node->right = insert(node->right, key);
else {
printf("duplicate\n");
}
/* return the (unchanged) node pointer */
return node;
}
struct node * minValueNode(struct node* node)
{
struct node* current = node;
/* loop down to find the leftmost leaf */
while (current->left != NULL)
current = current->left;
return current;
}
/* Given a binary search tree and a key, this function deletes the key
and returns the new root */
struct node* deleteNode(struct node* root, int key)
{
// base case
if (root == NULL) return root;
// If the key to be deleted is smaller than the root's key,
// then it lies in left subtree
if (key < root->key)
root->left = deleteNode(root->left, key);
// If the key to be deleted is greater than the root's key,
// then it lies in right subtree
else if (key > root->key)
root->right = deleteNode(root->right, key);
// if key is same as root's key, then This is the node
// to be deleted
else
{
// node with only one child or no child
if (root->left == NULL)
{
struct node *temp = root->right;
free(root);
return temp;
}
else if (root->right == NULL)
{
struct node *temp = root->left;
free(root);
return temp;
}
// node with two children: Get the inorder successor (smallest
// in the right subtree)
struct node* temp = minValueNode(root->right);
// Copy the inorder successor's content to this node
root->key = temp->key;
// Delete the inorder successor
root->right = deleteNode(root->right, temp->key);
}
return root;
}
int isvalid(char command)
{
if(command=='i' || command == 'd' || command == 's' || command == 'p')
return 1;
else return 0;
}
int main()
{
char command;
int n;
struct node *root = NULL;
do
{
scanf("%c",&command);
if(command=='i')
{
scanf("%d",&n);
root = insert(root,n);
}
else if(command=='d')
{
scanf("%d",&n);
root = deleteNode(root,n);
}
else if(command=='s')
{
scanf("%d",&n);
if(search(root,n))
printf("present\n");
else printf("absent\n");
}
else if(command=='p')
{
inorder(root);
printf("\n");
}
else command = 'q';
// flushes the standard input
// (clears the input buffer)
while ((getchar()) != '\n');
}while(isvalid(command));
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

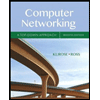
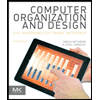
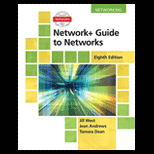
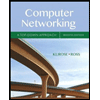
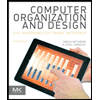
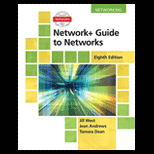
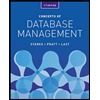
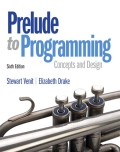
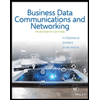