Hi! Can you help me to fix these error messages I get? Here is also some previous codes for my sudoku assignments if they help. --- def row_correct(sudoku: list, row_no: int): # Get the row to check row = sudoku[row_no] # Create a set to store the numbers that appear in the row nums_in_row = set() # Iterate through the elements in the row for num in row: # If the number is not 0 and it has already appeared in the set, return False if num != 0 and num in nums_in_row: return False # If the number is not 0, add it to the set elif num != 0: nums_in_row.add(num) # Return True if all checks pass return True --- def column_correct(sudoku, column_no): column = [] # Extract the values in the specified column for row in sudoku: column.append(row[column_no]) # Check if the column contains each number from 1 to 9 at most once for i in range(1, 10): if column.count(i) > 1: return False return True
Hi! Can you help me to fix these error messages I get? Here is also some previous codes for my sudoku assignments if they help. --- def row_correct(sudoku: list, row_no: int): # Get the row to check row = sudoku[row_no] # Create a set to store the numbers that appear in the row nums_in_row = set() # Iterate through the elements in the row for num in row: # If the number is not 0 and it has already appeared in the set, return False if num != 0 and num in nums_in_row: return False # If the number is not 0, add it to the set elif num != 0: nums_in_row.add(num) # Return True if all checks pass return True --- def column_correct(sudoku, column_no): column = [] # Extract the values in the specified column for row in sudoku: column.append(row[column_no]) # Check if the column contains each number from 1 to 9 at most once for i in range(1, 10): if column.count(i) > 1: return False return True
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter10: Pointers
Section10.4: Passing Addresses
Problem 1E
Related questions
Question
Hi! Can you help me to fix these error messages I get? Here is also some previous codes for my sudoku assignments if they help.
---
def row_correct(sudoku: list, row_no: int):
# Get the row to check
row = sudoku[row_no]
# Create a set to store the numbers that appear in the row
nums_in_row = set()
# Iterate through the elements in the row
for num in row:
# If the number is not 0 and it has already appeared in the set, return False
if num != 0 and num in nums_in_row:
return False
# If the number is not 0, add it to the set
elif num != 0:
nums_in_row.add(num)
# Return True if all checks pass
return True
---
def column_correct(sudoku, column_no):
column = []
# Extract the values in the specified column
for row in sudoku:
column.append(row[column_no])
# Check if the column contains each number from 1 to 9 at most once
for i in range(1, 10):
if column.count(i) > 1:
return False
return True
![FAIL: Sudoku Test: test_3_functinality_with_invalids
local variable 'val' referenced before assignment
FAIL: Sudoku Test: test_4_functionality_with_valids
Varmista että seuraava kutsu toimii
sudoku = [
[ 2, 6, 7, 8, 3, 9, 5, 0, 4 ],
[ 9, 0, 3, 5, 1, 0, 6, 0, 0],
[ 0, 5, 1, 6, 0, 0, 8, 3, 9 ],
2, 8 ],
[ 5, 1, 9, 0, 4, 6, 3,
[ 8, 0, 2, 1, 0, 5, 7, 0, 6 ],
[ 6, 7, 4, 3, 2, 0, 0, 0, 5 ],
[ 0, 0, 0, 4, 5,
7, 2, 6, 3 ],
[ 3, 2, 0, 0, 8, 0, 0, 5, 7 ],
[ 7, 4, 5, 0, 0, 3, 9, 0, 1 ],
]
sudoku_grid_correct (sudoku)](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fdca59d3b-f065-4790-9f9b-8546b85214d3%2F64b0eac0-65f4-4bd6-a9a7-ea052ccdf120%2Fov80is_processed.png&w=3840&q=75)
Transcribed Image Text:FAIL: Sudoku Test: test_3_functinality_with_invalids
local variable 'val' referenced before assignment
FAIL: Sudoku Test: test_4_functionality_with_valids
Varmista että seuraava kutsu toimii
sudoku = [
[ 2, 6, 7, 8, 3, 9, 5, 0, 4 ],
[ 9, 0, 3, 5, 1, 0, 6, 0, 0],
[ 0, 5, 1, 6, 0, 0, 8, 3, 9 ],
2, 8 ],
[ 5, 1, 9, 0, 4, 6, 3,
[ 8, 0, 2, 1, 0, 5, 7, 0, 6 ],
[ 6, 7, 4, 3, 2, 0, 0, 0, 5 ],
[ 0, 0, 0, 4, 5,
7, 2, 6, 3 ],
[ 3, 2, 0, 0, 8, 0, 0, 5, 7 ],
[ 7, 4, 5, 0, 0, 3, 9, 0, 1 ],
]
sudoku_grid_correct (sudoku)
![FAIL: Sudoku Test: test_0_main_program_ok
The code for testing the functions should be placed inside
if name
'___main__":
block. The following row should be moved:
N = 9
FAIL:
Sudoku Test: test_1_function_exists
Your code should contain function named as sudoku_grid_correct (sudoku: list)
FAIL: Sudoku Test: test_2_type_of_return_value
[[9, 0, 0, 0, 8, 0, 3, 0, 0], [², 0, 0, 2, 5, 0, 7, 0, 0], [0, 2, 0, 3, 0, 0, 0, 0, 4], [2,
9, 4, 0, 0, 0, 0, 0, 0], [0, 0, 0, 7, 3, 0, 5, 6, 0], [7, 0, 5, 0, 6, 0, 4, 0, 0], [0, 0, 7,
8, 0, 3, 9, 0, 0], [0, 0, 1, 0, 0, 0, 0, 0, 3], [3, 0, 0, 0, 0, 0, 0, 0, 2]]
FAIL: Sudoku Test: test_3_functinality_with_invalids
local variable 'val' referenced before assignment](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fdca59d3b-f065-4790-9f9b-8546b85214d3%2F64b0eac0-65f4-4bd6-a9a7-ea052ccdf120%2F1u6oa2x_processed.png&w=3840&q=75)
Transcribed Image Text:FAIL: Sudoku Test: test_0_main_program_ok
The code for testing the functions should be placed inside
if name
'___main__":
block. The following row should be moved:
N = 9
FAIL:
Sudoku Test: test_1_function_exists
Your code should contain function named as sudoku_grid_correct (sudoku: list)
FAIL: Sudoku Test: test_2_type_of_return_value
[[9, 0, 0, 0, 8, 0, 3, 0, 0], [², 0, 0, 2, 5, 0, 7, 0, 0], [0, 2, 0, 3, 0, 0, 0, 0, 4], [2,
9, 4, 0, 0, 0, 0, 0, 0], [0, 0, 0, 7, 3, 0, 5, 6, 0], [7, 0, 5, 0, 6, 0, 4, 0, 0], [0, 0, 7,
8, 0, 3, 9, 0, 0], [0, 0, 1, 0, 0, 0, 0, 0, 3], [3, 0, 0, 0, 0, 0, 0, 0, 2]]
FAIL: Sudoku Test: test_3_functinality_with_invalids
local variable 'val' referenced before assignment
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 6 steps with 6 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
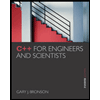
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
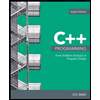
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
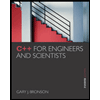
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
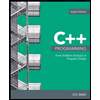
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning