Goal 1: Update the Fractions Class Here we will overload two functions that will used by the Recipe class later: multipliedBy, dividedBy Previously, these functions took a Fraction object as a parameter. Now ADD an implementation that takes an integer as a parameter. The functionality remains the same: Instead of multiplying/dividing the current object with another Fraction, it multiplies/divides with an integer (affecting numerator/denominator of the returned object). For example; a fraction 2/3 when multiplied by 4 becomes 8/3. Similarly, a fraction 2/3 when divided by 4 becomes 1/6. Goal 2: Update the Recipe Class The Recipe constructors so far did not specify how many servings the recipe is for. We will now add a private member variable of type int to denote the serving size and initialize it to 1. This means all recipes are initially constructed for a single serving. Update the overloaded extraction operator (<<) to include the serving size (see sample output below for an example of formatting) . New Member functions to the Recipe Class: 1. Add four member functions get the value of each of the member variable of the Recipe class. These are called getRecipeName(), getIngredientNames(), getIngredientQuantities(), getServingSize() and they return the value of the respective member variables. 2. Now add and implement a member function that scales the current recipe to a new serving size. The signature of this function is as follows: void scaleRecipe(int newServingSize); 3. Test your code by uncommenting the main.cpp until the line Recipe r4("recipeDumplings.txt"); to get the output below: Following Recipe has 4 ingredients --- Peanut Sauce Recipe for 1 --- Sweet Chilli Sauce (3/4) Peanut Butter (4/3) Soy Sauce (1/2) Hoisin Sauce (1/3) Scale Recipe to 3 servings --- Peanut Sauce Recipe for 3 --- Sweet Chilli Sauce (9/4) Peanut Butter (4) Soy Sauce (3/2) Hoisin Sauce (1) Scale Recipe to 2 servings --- Peanut Sauce Recipe for 2 --- Sweet Chilli Sauce (3/2) Peanut Butter (8/3) Soy Sauce (1) Hoisin Sauce (2/3) 4. Instead of using a constructor to specify all attributes of a recipe, we will next write a constructor to load them from a file. Note, all recipes initially constructed are for a serving size of 1. The signature of this constructor would be: Recipe(string filename); //loads the name, ingredients, and quantity of each recipe from a file Each following line has the quantity followed by the name of an ingredient. Dumplings 7/2 All Purpose Flour 3 Sesame Oil 3/2 Cabbage 1 Garlic Chives 3/2 Soy Sauce 1/3 Minced Ginger Your code should be able to handle a quantity that is Fractional or whole. You can do this by checking if the line has the '/' character. In addition, your code should read the name of the ingredient without any extra whitespaces. For example " Sesame Oil" should be stored as "Sesame Oil"; "Soy Sauce" should be stored as "Soy Sauce". Best way to do this is to use an istringstream to read individual words and then join them with a single blank space. Once you have implemented this constructor, test your code by uncommenting the next two lines and getting the following output: --- Dumplings Recipe for 1 --- All Purpose Flour (7/2) Sesame Oil (3) Cabbage (3/2) Garlic Chives (1) Soy Sauce (3/2) Minced Ginger (1/3) 5. The last method we will write for the recipe class is to combine current recipe with another. Recipe combineWith(Recipe& other) const; This method will create and return a new recipe by ADDing ingredients of the other recipe AFTER the ingredients of the current recipe. There is one catch: if the other recipe has an ingredient that is also there in the current recipe, then that ingredient's quantity is appropriately adjusted (and its name is not duplicated). See below the output if the next four lines from main.cpp are uncommented. Note that the ingredient "Soy Sauce" is common in both recipes. So it is only included once but its quantity is updated to be the sum of the quantities in each recipe. Combining Peanut Sauce with Dumplings --- Peanut Sauce with Dumplings Recipe for 1 --- Sweet Chilli Sauce (3/4) Peanut Butter (4/3) Soy Sauce (2) Hoisin Sauce (1/3) All Purpose Flour (7/2) Sesame Oil (3) Cabbage (3/2) Garlic Chives (1) Minced Ginger (1/3) --- Combined recipe has 9 ingredients --- Your last task is to write some client code Combine the Macroni and Lasagna recipes. Then, combine this new recipe just created with Four Cheese. Lastly, throw a party and scale the final recipe for 10 servings! Show off your recipe by printing it. See the desired output below: --- Macroni with Lasagna with Four Cheese Recipe for 10 --- Macroni (55/2) Water (105/2) Salt (100/3) Cheddar Cheese (55) Bowtie Pasta (40) Swiss Cheese (40/3) Marinara (35) Spinach (15/2) Crushed Red Pepper (5/2) Mozzarella Cheese (20) Provolone (15/2)
Goal 1: Update the Fractions Class
Here we will overload two functions that will used by the Recipe class later:
multipliedBy, dividedBy
Previously, these functions took a Fraction object as a parameter. Now ADD an implementation that takes an integer as a parameter. The functionality remains the same: Instead of multiplying/dividing the current object with another Fraction, it multiplies/divides with an integer (affecting numerator/denominator of the returned object). For example; a fraction 2/3 when multiplied by 4 becomes 8/3. Similarly, a fraction 2/3 when divided by 4 becomes 1/6.
Goal 2: Update the Recipe Class
The Recipe constructors so far did not specify how many servings the recipe is for. We will now add a private member variable of type int to denote the serving size and initialize it to 1. This means all recipes are initially constructed for a single serving. Update the overloaded extraction operator (<<) to include the serving size (see sample output below for an example of formatting) .
New Member functions to the Recipe Class:
1. Add four member functions get the value of each of the member variable of the Recipe class. These are called
getRecipeName(), getIngredientNames(), getIngredientQuantities(), getServingSize()
and they return the value of the respective member variables.
2. Now add and implement a member function that scales the current recipe to a new serving size. The signature of this function is as follows:
void scaleRecipe(int newServingSize);
3. Test your code by uncommenting the main.cpp until the line
Recipe r4("recipeDumplings.txt");
to get the output below:
Following Recipe has 4 ingredients
--- Peanut Sauce Recipe for 1 ---
Sweet Chilli Sauce (3/4)
Peanut Butter (4/3)
Soy Sauce (1/2)
Hoisin Sauce (1/3)
Scale Recipe to 3 servings
--- Peanut Sauce Recipe for 3 ---
Sweet Chilli Sauce (9/4)
Peanut Butter (4)
Soy Sauce (3/2)
Hoisin Sauce (1)
Scale Recipe to 2 servings
--- Peanut Sauce Recipe for 2 ---
Sweet Chilli Sauce (3/2)
Peanut Butter (8/3)
Soy Sauce (1)
Hoisin Sauce (2/3)
4. Instead of using a constructor to specify all attributes of a recipe, we will next write a constructor to load them from a file. Note, all recipes initially constructed are for a serving size of 1. The signature of this constructor would be:
Recipe(string filename); //loads the name, ingredients, and quantity of each recipe from a file
Each following line has the quantity followed by the name of an ingredient.
Dumplings
7/2 All Purpose Flour
3 Sesame Oil
3/2 Cabbage
1 Garlic Chives
3/2 Soy Sauce
1/3 Minced Ginger
Your code should be able to handle a quantity that is Fractional or whole. You can do this by checking if the line has the '/' character. In addition, your code should read the name of the ingredient without any extra whitespaces. For example " Sesame Oil" should be stored as "Sesame Oil"; "Soy Sauce" should be stored as "Soy Sauce". Best way to do this is to use an istringstream to read individual words and then join them with a single blank space.
Once you have implemented this constructor, test your code by uncommenting the next two lines and getting the following output:
--- Dumplings Recipe for 1 ---
All Purpose Flour (7/2)
Sesame Oil (3)
Cabbage (3/2)
Garlic Chives (1)
Soy Sauce (3/2)
Minced Ginger (1/3)
5. The last method we will write for the recipe class is to combine current recipe with another.
Recipe combineWith(Recipe& other) const;
This method will create and return a new recipe by ADDing ingredients of the other recipe AFTER the ingredients of the current recipe. There is one catch: if the other recipe has an ingredient that is also there in the current recipe, then that ingredient's quantity is appropriately adjusted (and its name is not duplicated). See below the output if the next four lines from main.cpp are uncommented. Note that the ingredient "Soy Sauce" is common in both recipes. So it is only included once but its quantity is updated to be the sum of the quantities in each recipe.
Combining Peanut Sauce with Dumplings
--- Peanut Sauce with Dumplings Recipe for 1 ---
Sweet Chilli Sauce (3/4)
Peanut Butter (4/3)
Soy Sauce (2)
Hoisin Sauce (1/3)
All Purpose Flour (7/2)
Sesame Oil (3)
Cabbage (3/2)
Garlic Chives (1)
Minced Ginger (1/3)
--- Combined recipe has 9 ingredients ---
Your last task is to write some client code
Combine the Macroni and Lasagna recipes. Then, combine this new recipe just created with Four Cheese. Lastly, throw a party and scale the final recipe for 10 servings! Show off your recipe by printing it. See the desired output below:
--- Macroni with Lasagna with Four Cheese Recipe for 10 ---
Macroni (55/2)
Water (105/2)
Salt (100/3)
Cheddar Cheese (55)
Bowtie Pasta (40)
Swiss Cheese (40/3)
Marinara (35)
Spinach (15/2)
Crushed Red Pepper (5/2)
Mozzarella Cheese (20)
Provolone (15/2)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

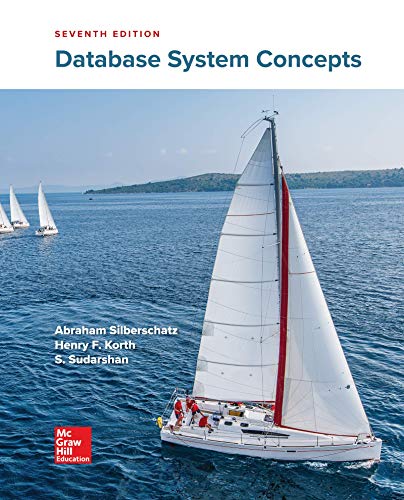
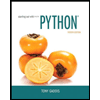
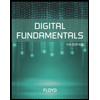
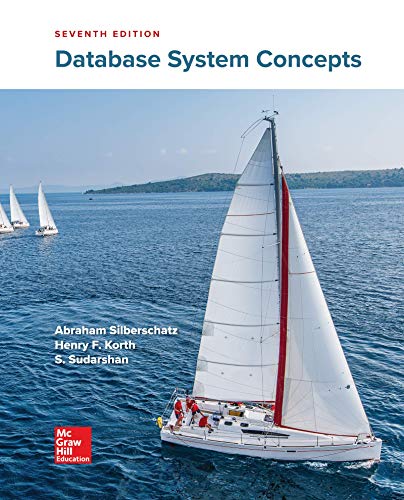
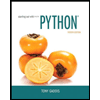
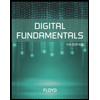
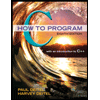
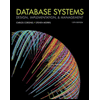
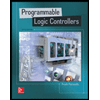