For this project, you will create a class for an elementary school that will allow a teacher to enter each student's seating assignment. Occasionally, the teacher may need to switch the seating assignment of a student. If there is a seat available, the student is moved. If all of the seats are already assigned, the teacher will have two students trade seats. Minimum Requirements: • Create a class called student that holds information about one student including the student's name. The class must have methods to set each instance variable and methods to get each instance variable. • Create a class called seatingChart and create a multidimensional array of student objects as an instance member. The array of student objects should be able to handle a class of size 20. You will not get credit for this assignment if you do not use a multidimensional array for the seating chart. • Include an addStudent function that has parameters for a student name, target seat row and column to add one student to the seating chart array in the multidimensional array position [row, column] provided by the parameters. Position [0,0] represents the seat closest to the teacher's desk. Do not store a seat number in the student class; the array index will represent each seat for the seating assignments. • Include a swapStudents function that has parameters for a student name, target seat row and column. This function should find a student's name in the multidimensional array and swap the student from his or her original seat to the target seat in the multidimensional array position [row, column] provided by the parameters. The result will be that those students trade seating assignments.
For this project, you will create a class for an elementary school that will allow a teacher to enter each student's seating assignment. Occasionally, the teacher may need to switch the seating assignment of a student. If there is a seat available, the student is moved. If all of the seats are already assigned, the teacher will have two students trade seats. Minimum Requirements: • Create a class called student that holds information about one student including the student's name. The class must have methods to set each instance variable and methods to get each instance variable. • Create a class called seatingChart and create a multidimensional array of student objects as an instance member. The array of student objects should be able to handle a class of size 20. You will not get credit for this assignment if you do not use a multidimensional array for the seating chart. • Include an addStudent function that has parameters for a student name, target seat row and column to add one student to the seating chart array in the multidimensional array position [row, column] provided by the parameters. Position [0,0] represents the seat closest to the teacher's desk. Do not store a seat number in the student class; the array index will represent each seat for the seating assignments. • Include a swapStudents function that has parameters for a student name, target seat row and column. This function should find a student's name in the multidimensional array and swap the student from his or her original seat to the target seat in the multidimensional array position [row, column] provided by the parameters. The result will be that those students trade seating assignments.
Programming with Microsoft Visual Basic 2017
8th Edition
ISBN:9781337102124
Author:Diane Zak
Publisher:Diane Zak
Chapter10: Classes And Objects
Section: Chapter Questions
Problem 2E: Open the Palace Solution.sln file contained in the VB2017\Chap10\Palace Solution folder.
Use Windows...
Related questions
Question
100%
![For this project, you will create a class for an elementary school that will allow a teacher to enter each student's seating assignment. Occasionally, the teacher may need to switch
the seating assignment of a student. If there is a seat available, the student is moved. If all of the seats are already assigned, the teacher will have two students trade seats.
Minimum Requirements:
• Create a class called student that holds information about one student including the student's name. The class must have methods to set each instance variable and methods to
get each instance variable.
• Create a class called seatingChart and create a multidimensional array of student objects as an instance member. The array of student objects should be able to handle a class
of size 20. You will not get credit for this assignment if you do not use a multidimensional array for the seating chart.
• Include an addStudent function that has parameters for a student name, target seat row and column to add one student to the seating chart array in the multidimensional array
position [row, column] provided by the parameters. Position [0,0] represents the seat closest to the teacher's desk. Do not store a seat number in the student class; the array
index will represent each seat for the seating assignments.
• Include a swapStudents function that has parameters for a student name, target seat row and column. This function should find a student's name in the multidimensional array
and swap the student from his or her original seat to the target seat in the multidimensional array position [row, column] provided by the parameters. The result will be that
those students trade seating assignments.
Note: Java does throws a null exception when you try to access a null student in the multidimensional array, so I recommend initializing the multidimensional array to fill it with a
default student. To do this, create an object of the student class with default values for the instance variables and load the entire array with that object. When you add a student,
you can overwrite the default student with the new student.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fcb2584b9-3c16-410b-8e35-749ba9c43d09%2F5ad1eb83-0577-4791-97b8-866e3b49a69d%2F41s313_processed.png&w=3840&q=75)
Transcribed Image Text:For this project, you will create a class for an elementary school that will allow a teacher to enter each student's seating assignment. Occasionally, the teacher may need to switch
the seating assignment of a student. If there is a seat available, the student is moved. If all of the seats are already assigned, the teacher will have two students trade seats.
Minimum Requirements:
• Create a class called student that holds information about one student including the student's name. The class must have methods to set each instance variable and methods to
get each instance variable.
• Create a class called seatingChart and create a multidimensional array of student objects as an instance member. The array of student objects should be able to handle a class
of size 20. You will not get credit for this assignment if you do not use a multidimensional array for the seating chart.
• Include an addStudent function that has parameters for a student name, target seat row and column to add one student to the seating chart array in the multidimensional array
position [row, column] provided by the parameters. Position [0,0] represents the seat closest to the teacher's desk. Do not store a seat number in the student class; the array
index will represent each seat for the seating assignments.
• Include a swapStudents function that has parameters for a student name, target seat row and column. This function should find a student's name in the multidimensional array
and swap the student from his or her original seat to the target seat in the multidimensional array position [row, column] provided by the parameters. The result will be that
those students trade seating assignments.
Note: Java does throws a null exception when you try to access a null student in the multidimensional array, so I recommend initializing the multidimensional array to fill it with a
default student. To do this, create an object of the student class with default values for the instance variables and load the entire array with that object. When you add a student,
you can overwrite the default student with the new student.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
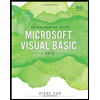
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning
COMPREHENSIVE MICROSOFT OFFICE 365 EXCE
Computer Science
ISBN:
9780357392676
Author:
FREUND, Steven
Publisher:
CENGAGE L
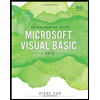
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning
COMPREHENSIVE MICROSOFT OFFICE 365 EXCE
Computer Science
ISBN:
9780357392676
Author:
FREUND, Steven
Publisher:
CENGAGE L