For this assignment, you will be building a class, creating objects, doing comparisons and using nested control statements. You need to remember what you have learned in class, lab, books and your assignments. Be sure to refer to them when you need to. There are 2 parts to this assignment. In the first part, you are going to be given a problem and you will then need to create a structure, write algorithms and a flow chart to solve it. In the second part, you’ll be turning this into a java program. So let’s get started! Part 1: Your haunted house adventure: The user always starts the game at the front door and must immediately decide where they want to go. Your movement constraints are as follows: -From the front door, the user must choose between going into the living room, dining room or up the stairs. -To get from one room to the next, there must be a door. That means that to get to some rooms, the user must go through other rooms. See the above diagram for more details. -If the user is in a room that is attached to another room via a door (not the one they just came in—no backtracking!), the user must be given the option between going to the other room or exploring an item in the current room. -If the user is in a room that has no other exit, then they must be given the option between exploring the items in the room. -The user will have a backpack that contains the item they’ve collected during their journey. -The backpack does NOT have to be an array. -The backpack can be a simple string, which can contain the item that a user has. -Use the contains method on the string that is acting as the user’s inventory to find out what item(s) they currently contain. e.g. inventory.contains(“string”) will return true if the word “string” is anywhere within the string inventory. Possible rooms to explore: Floor Room Item(s) Outcome 1 Living Room Chest Ghost escapes and scares you to death 1 Dining Room Candelabra Light up by themselves and see a death shadow 1 Kitchen Refrigerator Open it and find some delicious soul food 1 Kitchen Cabinet The dishes and glasses start flying at you as soon as you open the door. You get hit in the head and feel yourself start moving towards a light 1 Pantry Dusty recipe box You open it up and a recipe for chocolate devils food cake appears our of no where 1 Pantry Broom Flies up in the air as soon as you touch it 1 Bathroom Mirror See a bloody face looking back at you 1 Bathroom Shower Room suddenly steams up and you feel fingers touching the back of your neck 2 Bedroom 1 Rocking Chair Chair starts rocking by itself with no one in it 2 Bedroom 1 Window See a child outside on a swing who suddenly disappears 2 Bedroom 2 Doll House The dolls start dancing on their own 2 Bedroom 2 Dresser A ghost flies out of the dresser as soon as you open it and goes right though your body 2 Master Bedroom Jewelry Box You find the cursed Hope Diamond and feel your doom 2 Master Bathroom Intricate Oil Lamp Rub the lamp and a genie pops out who says he’ll grant you 3 wishes 2 Master Bathroom Shower Suddenly hear singing in the shower, but no one is there 2 Bathroom Mirror See a bloody face looking back at you 2 Bathroom Shower Room suddenly steams up and you feel fingers touching the back of your neck Program Flow: -Ask the user to enter their name so that you can personalize their experience. You will want to use their name as they move through the house and make decisions -Start at the front door as described above. -Each time the user moves to a new room, you must then ask the user what he or she wants to do next. The options available are derived above. Note that there are sometimes more that 2 options available. -Should the user reach a room where there is no other exit, they must select an object to explore. -Once the object is explored, it must be put into the backpack. The game is over. This should be indicated clearly to the user (and have fun!). Input/Output Requirements: -Welcome the user to the game. Be sure to include their name in your welcome message. -Using ascii art or graphics, print out an image showing where in the house the user is starting. -For each step, present the users with their options and ask them what they want to do (hint: put word options in quotes to indicate what they should type in to respond to your question) -Once they have selected an object to explore, be sure to print out their final outcome, including telling them what item they have in their backpack. -At the end of the game, using ascii art or graphics, print out an image showing where in the house the user ended the game. Program Structure Requirements: -For your class structure, you must use classes, objects and methods. -Do NOT just use a huge series of sequential if statements, nested if statements or switch statements for your program flow
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
For this assignment, you will be building a class, creating objects, doing comparisons and using nested control statements. You need to remember what you have learned in class, lab, books and your assignments. Be sure to refer to them when you need to.
There are 2 parts to this assignment. In the first part, you are going to be given a problem and you will then need to create a structure, write
So let’s get started!
Part 1: Your haunted house adventure:
The user always starts the game at the front door and must immediately decide where they want to go. Your movement constraints are as follows:
-From the front door, the user must choose between going into the living room, dining room or up the stairs.
-To get from one room to the next, there must be a door. That means that to get to some rooms, the user must go through other rooms. See the above diagram for more details.
-If the user is in a room that is attached to another room via a door (not the one they just came in—no backtracking!), the user must be given the option between going to the other room or exploring an item in the current room.
-If the user is in a room that has no other exit, then they must be given the option between exploring the items in the room.
-The user will have a backpack that contains the item they’ve collected during their journey.
-The backpack does NOT have to be an array.
-The backpack can be a simple string, which can contain the item that a user has.
-Use the contains method on the string that is acting as the user’s inventory to find out what item(s) they currently contain.
e.g. inventory.contains(“string”) will return true if the word “string” is anywhere within the string inventory.
Possible rooms to explore:
Floor |
Room |
Item(s) |
Outcome |
1 |
Living Room |
Chest |
Ghost escapes and scares you to death |
1 |
Dining Room |
Candelabra |
Light up by themselves and see a death shadow |
1 |
Kitchen |
Refrigerator |
Open it and find some delicious soul food |
1 |
Kitchen |
Cabinet |
The dishes and glasses start flying at you as soon as you open the door. You get hit in the head and feel yourself start moving towards a light |
1 |
Pantry |
Dusty recipe box |
You open it up and a recipe for chocolate devils food cake appears our of no where |
1 |
Pantry |
Broom |
Flies up in the air as soon as you touch it |
1 |
Bathroom |
Mirror |
See a bloody face looking back at you |
1 |
Bathroom |
Shower |
Room suddenly steams up and you feel fingers touching the back of your neck |
2 |
Bedroom 1 |
Rocking Chair |
Chair starts rocking by itself with no one in it |
2 |
Bedroom 1 |
Window |
See a child outside on a swing who suddenly disappears |
2 |
Bedroom 2 |
Doll House |
The dolls start dancing on their own |
2 |
Bedroom 2 |
Dresser |
A ghost flies out of the dresser as soon as you open it and goes right though your body |
2 |
Master Bedroom |
Jewelry Box |
You find the cursed Hope Diamond and feel your doom |
2 |
Master Bathroom |
Intricate Oil Lamp |
Rub the lamp and a genie pops out who says he’ll grant you 3 wishes |
2 |
Master Bathroom |
Shower |
Suddenly hear singing in the shower, but no one is there |
2 |
Bathroom |
Mirror |
See a bloody face looking back at you |
2 |
Bathroom |
Shower |
Room suddenly steams up and you feel fingers touching the back of your neck |
Program Flow:
-Ask the user to enter their name so that you can personalize their experience. You will want to use their name as they move through the house and make decisions
-Start at the front door as described above.
-Each time the user moves to a new room, you must then ask the user what he or she wants to do next. The options available are derived above. Note that there are sometimes more that 2 options available.
-Should the user reach a room where there is no other exit, they must select an object to explore.
-Once the object is explored, it must be put into the backpack. The game is over. This should be indicated clearly to the user (and have fun!).
Input/Output Requirements:
-Welcome the user to the game. Be sure to include their name in your welcome message.
-Using ascii art or graphics, print out an image showing where in the house the user is starting.
-For each step, present the users with their options and ask them what they want to do (hint: put word options in quotes to indicate what they should type in to respond to your question)
-Once they have selected an object to explore, be sure to print out their final outcome, including telling them what item they have in their backpack.
-At the end of the game, using ascii art or graphics, print out an image showing where in the house the user ended the game.
Program Structure Requirements:
-For your class structure, you must use classes, objects and methods.
-Do NOT just use a huge series of sequential if statements, nested if statements or switch statements for your program flow


Trending now
This is a popular solution!
Step by step
Solved in 10 steps with 2 images

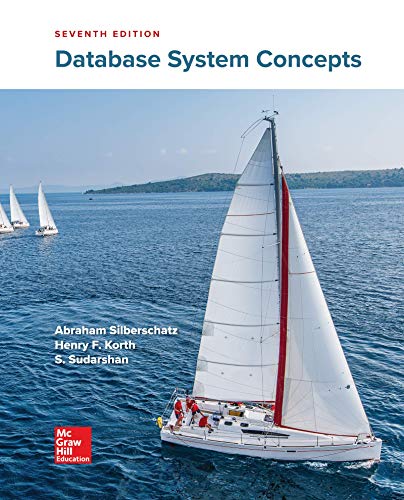
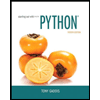
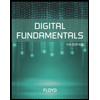
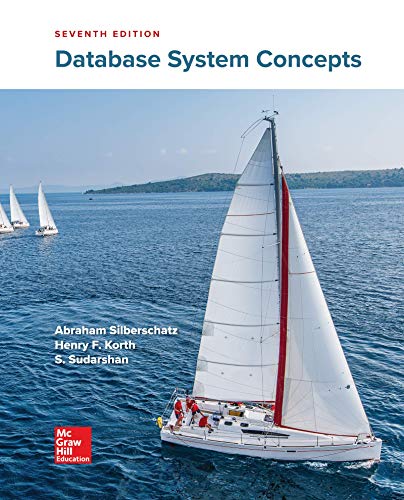
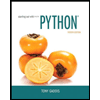
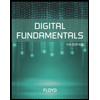
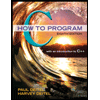
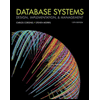
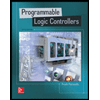