def integer_0_or_1(interger): #if value is true return 1 if truth_value: return 1 else: #else return 0 return 0 def truth_value(integer): """Convert an integer into a truth value.""" # Convert 0 into False and all other integers, # including 1, into True if integer == 0: truth_value = False else: truth_value = True return truth_value print(truth_value(True)) print(truth_value(False)) This question assesses Block 2 Part 4. It assumes an understanding of binary notation and truth tables (Block 1 Part 1). Write a function and_binary(), which takes two integers (each is either 0 or 1) and returns an integer in accordance with the truth table: A B and_binary(A, B) 0 0 0 0 1 0 1 0 0 1 1 1 Before you dive into writing your function, first decompose the problem. Then, write an algorithm. Only after that, implement your solution in Python. Hint: When you have decomposed the problem, you should be able to implement some of the sub-problems by using: the function truth_value() from the previous quiz question the function integer_0_or_1() from the previous quiz question Python’s and Boolean operator. Recall that the Boolean operators and, or and not can be used to carry out Boolean operations on the truth values. This is illustrated by the following calls in the Python shell: >>> not(True) False >>> True and not(False) True You can apply the operators to the literals True and False, as shown above, and also to variables that have been assigned to the value True or the value False: >>> truth_value_1 = True >>> truth_value_2 = False >>> truth_value_1 and truth_value_2 False You will find the definitions of truth_value() and integer_0_or_1() in the answer box below. All you need to add below is your definition of the function and_binary(). It will be tested automatically against relevant inputs (i.e. the test cases that are represented by the truth table for and_binary() that is provided above). So, add your function definition and nothing more. For example: Test Result print(and_binary(0, 0)) 0 the first code is mine but it won't pass the inbuilt tests of my quiz
def integer_0_or_1(interger):
#if value is true return 1
if truth_value:
return 1
else:
#else return 0
return 0
def truth_value(integer):
"""Convert an integer into a truth value."""
# Convert 0 into False and all other integers,
# including 1, into True
if integer == 0:
truth_value = False
else:
truth_value = True
return truth_value
print(truth_value(True))
print(truth_value(False))
This question assesses Block 2 Part 4. It assumes an understanding of binary notation and truth tables (Block 1 Part 1).
Write a function and_binary(), which takes two integers (each is either 0 or 1) and returns an integer in accordance with the truth table:
A |
B |
and_binary(A, B) |
0 |
0 |
0 |
0 |
1 |
0 |
1 |
0 |
0 |
1 |
1 |
1 |
Before you dive into writing your function, first decompose the problem. Then, write an
Hint: When you have decomposed the problem, you should be able to implement some of the sub-problems by using:
- the function truth_value() from the previous quiz question
- the function integer_0_or_1() from the previous quiz question
- Python’s and Boolean operator.
Recall that the Boolean operators and, or and not can be used to carry out Boolean operations on the truth values. This is illustrated by the following calls in the Python shell:
False
>>> True and not(False)
True
You can apply the operators to the literals True and False, as shown above, and also to variables that have been assigned to the value True or the value False:
>>> truth_value_2 = False
>>> truth_value_1 and truth_value_2
False
You will find the definitions of truth_value() and integer_0_or_1() in the answer box below. All you need to add below is your definition of the function and_binary(). It will be tested automatically against relevant inputs (i.e. the test cases that are represented by the truth table for and_binary() that is provided above). So, add your function definition and nothing more.
For example:
Test | Result |
---|---|
print(and_binary(0, 0))
|
0
|
the first code is mine but it won't pass the inbuilt tests of my quiz

Step by step
Solved in 3 steps with 1 images

this is the error i am getting on test
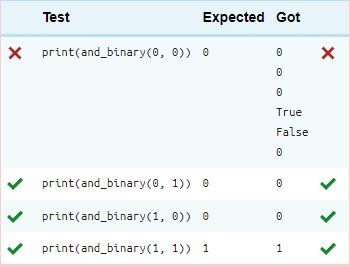
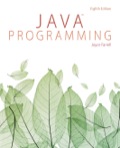
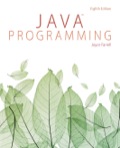