Code requirements: A robot is positioned on an integral point in a two-dimensional coordinate grid (xr, yr). There is a treasure that has been placed at a point in the same grid at (xt, yt). All x’s and y’s will be integral values. The robot can move up (North), down (South), left (West), or right (East). Commands can be given to the robot to move one position in one of the four direction. That is, “E” moves a robot one slot East (to the right) so if the robot was on position (3, 4), it would now be on (4, 4). The command N would move the robot one position north so a robot at position (4, 4) would be at (4, 5). Because the robot cannot move diagonally, the shortest distance between a robot at (xr, yr) and a treasure at (xt, yt) is | xr – xt | + | yr - yt | = ShortestPossibleDistance Write a recursive program which determines all the unique shortest possible paths from the robot to the treasure with the following stipulation: The robot may never move in the same direction more than MaxDistance times in a row. The input to the program will be the starting position of the robot (xr, yr), followed by the position of the treasure (xt, yt), followed by the MaxDistance parameter. Assume that all five are integers and do not worry about error conditions in inputs. Take these parameters as arguments to the program. For instance, an input of 1 3 -2 4 2 corresponds to the robot starting at position (1, 3) and needing to get to position (-2, 4) with the constraints that one can only move 2 steps in one direction before having to shift to a new position. The output of the program should be the listing of all the unique shortest possible paths followed by the number of unique paths. The paths must follow the stipulation whereby the robot cannot move in the same direction more than MaxDistance times in a row. A path should be output as a string of characters with each character corresponding to a direction the Robot should move. For instance, NNENE corresponds to having the robot move North, North, East, North and East. This would be one answer to the input: 3 3 5 6 2, which corresponds to (3,3) - > (5,6) with a MaxDistance of 2. / / / / This is a C++ program. I was using this solution I found as a reference and used concepts from it to build my code, but I couldn't figure out how to incorporate the Max Distance without getting a bunch of errors (this code doesn't include max distance). That's all I need solved. Read the assignment and just post the reworked solution with MaxDistance in it, thanks! #include #include using namespace std; int findPermutations(string str, int index, int n,int counts) { if (index >= n) { cout << str << endl; return 1+counts; } for (int i = index; i < n; i++) { bool check=true; for (int j = index; j < i; j++) { if (str[j] == str[i]) { check=false; } } if (check) { char temp=str[index]; str[index]=str[i]; str[i]=temp; counts=findPermutations(str, index + 1, n,counts); temp=str[index]; str[index]=str[i]; str[i]=temp; } } return counts; } int main() { int x1,x2,y1,y2,counts=0; string s=""; char str[100]; cout<<"Enter the coordinates as x1 y1 x2 y2 where x1 and y1 are initial and x2 and y2 are final: "; cin>>x1>>y1>>x2>>y2; if(x2>x1) { for(int i=0;i<(x2-x1);i++) { s=s+"E"; } } else { for(int i=0;i<(x1-x2);i++) { s=s+"W"; } } if(y2>y1) { for(int i=0;i<(y2-y1);i++) { s=s+"N"; } } else { for(int i=0;i<(y1-y2);i++) { s=s+"S"; } } int n = s.length(); cout<<"Number of paths are: "< return 0; }
Code requirements:
A robot is positioned on an integral point in a two-dimensional coordinate grid (xr, yr). There is a treasure that has been placed at a point in the same grid at (xt, yt). All x’s and y’s will be integral values. The robot can move up (North), down (South), left (West), or right (East). Commands can be given to the robot to move one position in one of the four direction. That is, “E” moves a robot one slot East (to the right) so if the robot was on position (3, 4), it would now be on (4, 4). The command N would move the robot one position north so a robot at position (4, 4) would be at (4, 5). Because the robot cannot move diagonally, the shortest distance between a robot at (xr, yr) and a treasure at (xt, yt) is | xr – xt | + | yr - yt | = ShortestPossibleDistance Write a recursive
/
/
/
/
This is a C++ program. I was using this solution I found as a reference and used concepts from it to build my code, but I couldn't figure out how to incorporate the Max Distance without getting a bunch of errors (this code doesn't include max distance). That's all I need solved. Read the assignment and just post the reworked solution with MaxDistance in it, thanks!
#include
#include
using namespace std;
int findPermutations(string str, int index, int n,int counts)
{
if (index >= n) {
cout << str << endl;
return 1+counts;
}
for (int i = index; i < n; i++) {
bool check=true;
for (int j = index; j < i; j++)
{
if (str[j] == str[i])
{
check=false;
}
}
if (check) {
char temp=str[index];
str[index]=str[i];
str[i]=temp;
counts=findPermutations(str, index + 1, n,counts);
temp=str[index];
str[index]=str[i];
str[i]=temp;
}
}
return counts;
}
int main()
{
int x1,x2,y1,y2,counts=0;
string s="";
char str[100];
cout<<"Enter the coordinates as x1 y1 x2 y2 where x1 and y1 are initial and x2 and y2 are final: ";
cin>>x1>>y1>>x2>>y2;
if(x2>x1)
{
for(int i=0;i<(x2-x1);i++)
{
s=s+"E";
}
}
else
{
for(int i=0;i<(x1-x2);i++)
{
s=s+"W";
}
}
if(y2>y1)
{
for(int i=0;i<(y2-y1);i++)
{
s=s+"N";
}
}
else
{
for(int i=0;i<(y1-y2);i++)
{
s=s+"S";
}
}
int n = s.length();
cout<<"Number of paths are: "< return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

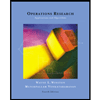
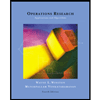