C++ Assignment Setup Part 1: Working With Process IDs Modify the getProcessID() function in the file named Processes.cpp The function must find and store the process's own process id The function must return the process id to the calling program. Note that the function currently returns a default value of -1. Hint: search for “process id” in the “System Calls” section of the Linux manual. Part 2: Working With Multiple Processes Modify the createNewProcess() function in the file named Processes.cpp as follows: The child process must print the message I am a child process! The child process must then return a string with the message I am bored of my parent, switching programs now The parent process must print the message I just became a parent! The parent process must then wait until the child process terminates, at which point it must return a string with the message My child process just terminated! and then terminate itself. Hint: search for “wait for process” in the “System Calls” section of the Linux manual. IMPORTANT: Do NOT type the messages, copy-paste them from here. Your output must be exact. Part 3: Working With External Commands Modify the replaceProcess() function in the file named Processes.cpp as follows: The parent process will use the fork() function to create a child process. This step has been done for you. The child process must then change its memory image to a different program by using the execvp system call (http://linux.die.net/man/3/execvp). The parameter args that has been passed to the replaceProcess() function is the array of parameters to be passed to execvp, telling it which program to execute and what parameters to pass to that program. For example, the test code provided to you executes the “ls” (directory list) program with the parameter “-al” by setting the args array as follows: char * args[3] = {(char * )"ls", (char * )"-al", NULL}; IMPORTANT: Although the test code executes the “ls” program, we must be able to change the command that execvp executes. So, DO NOT hardcode the command to be passed to execvp. Simply use the args array provided. Finally, in the parent process, you must make sure to invoke the necessary system call to wait for the child process to terminate. Once the child terminates, exit the program. Expected Output: If you execute the code you pull from GitLab without any modifications, it will produce the following output: If you make all required changes correctly, your program should produce output similar to the following: below is my code which isnt giving the correct output when i run it // // Processes.cpp // ITSC 3146 // // Created by Bahamon, Julio on 1/12/17. // /* @file Processes.cpp @author student name, student@uncc.edu @author student name, student@uncc.edu @author student name, student@uncc.edu @description: @course: ITSC 3146 @assignment: in-class activity [n] */ #ifndef Processes_cpp #define Processes_cpp //Import required header file #include "Processes.h" using namespace std; //Part 1: Working With Process IDs pid_t getProcessID(void) { // TODO: Add your code here //Get the pid of the process pid_t id = getpid(); //return the id return id; } //Part 2: Working With Multiple Processes //Implement method to create child process string createNewProcess(void) { //Create a child process pid_t id = fork(); // DO NOT CHANGE THIS LINE OF CODE process_id = id; //If the id is -1 if (id == -1) { return "Error creating process"; } //If the ID is 0 else if (id == 0) { cout << "I am a child process! "; return "I am bored of my parent, switching programs now"; } //Otherwise else { cout << "I just became a parent! "; int status = 0; wait(&status); return "My child process just terminated!"; } } //Part 3: Working With External Commands" //Implement the method to replace the process void replaceProcess(char * args[]) { //Create child process pid_t id = fork(); //Execute with the new process execvp(args[0], args); } #endif /* TestProg_cpp */ the output is in images
C++
Assignment Setup
Part 1: Working With Process IDs
-
Modify the getProcessID() function in the file named Processes.cpp
-
The function must find and store the process's own process id
-
The function must return the process id to the calling
program . Note that the function currently returns a default value of -1.
Hint: search for “process id” in the “System Calls” section of the Linux manual.
-
Part 2: Working With Multiple Processes
-
Modify the createNewProcess() function in the file named Processes.cpp as follows:
-
The child process must print the message I am a child process!
-
The child process must then return a string with the message I am bored of my parent, switching programs now
-
The parent process must print the message I just became a parent!
-
The parent process must then wait until the child process terminates, at which point it must return a string with the message My child process just terminated! and then terminate itself. Hint: search for “wait for process” in the “System Calls” section of the Linux manual.
IMPORTANT:
Do NOT type the messages, copy-paste them from here. Your output must be exact.
-
Part 3: Working With External Commands
-
Modify the replaceProcess() function in the file named Processes.cpp as follows:
-
The parent process will use the fork() function to create a child process. This step has been done for you.
-
The child process must then change its memory image to a different program by using the execvp system call (http://linux.die.net/man/3/execvp). The parameter args that has been passed to the replaceProcess() function is the array of parameters to be passed to execvp, telling it which program to execute and what parameters to pass to that program.
-
For example, the test code provided to you executes the “ls” (directory list) program with the parameter “-al” by setting the args array as follows:
char * args[3] = {(char * )"ls", (char * )"-al", NULL};
IMPORTANT:
Although the test code executes the “ls” program, we must be able to change the command that execvp executes. So, DO NOT hardcode the command to be passed to execvp. Simply use the args array provided.
-
Finally, in the parent process, you must make sure to invoke the necessary system call to wait for the child process to terminate. Once the child terminates, exit the program.
Expected Output:
If you execute the code you pull from GitLab without any modifications, it will produce the following output:
If you make all required changes correctly, your program should produce output similar to the following:
below is my code which isnt giving the correct output when i run it
//
// Processes.cpp
// ITSC 3146
//
// Created by Bahamon, Julio on 1/12/17.
//
/*
@file Processes.cpp
@author student name, student@uncc.edu
@author student name, student@uncc.edu
@author student name, student@uncc.edu
@description: <ADD DESCRIPTION>
@course: ITSC 3146
@assignment: in-class activity [n]
*/
#ifndef Processes_cpp
#define Processes_cpp
//Import required header file
#include "Processes.h"
using namespace std;
//Part 1: Working With Process IDs
pid_t getProcessID(void)
{
// TODO: Add your code here
//Get the pid of the process
pid_t id = getpid();
//return the id
return id;
}
//Part 2: Working With Multiple Processes
//Implement method to create child process
string createNewProcess(void)
{
//Create a child process
pid_t id = fork();
// DO NOT CHANGE THIS LINE OF CODE
process_id = id;
//If the id is -1
if (id == -1)
{
return "Error creating process";
}
//If the ID is 0
else if (id == 0)
{
cout << "I am a child process! ";
return "I am bored of my parent, switching programs now";
}
//Otherwise
else
{
cout << "I just became a parent! ";
int status = 0;
wait(&status);
return "My child process just terminated!";
}
}
//Part 3: Working With External Commands"
//Implement the method to replace the process
void replaceProcess(char * args[])
{
//Create child process
pid_t id = fork();
//Execute with the new process
execvp(args[0], args);
}
#endif /* TestProg_cpp */
the output is in images


Trending now
This is a popular solution!
Step by step
Solved in 7 steps with 3 images

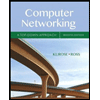
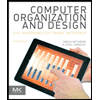
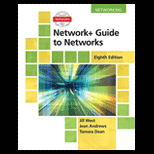
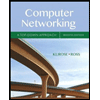
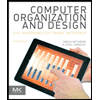
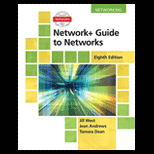
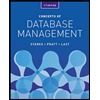
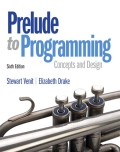
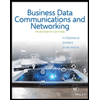