ASSIGNMENTWrite a Java program that meets these requirements.• Create a NetBeans project named LastnameAssign1. Change Lastname to your last name. For example, my project would be named NicholsonAssign1.• In the Java file, print a welcome message that includes your full name.• The program should prompt for an XML filename to write too The filename entered must end with .xml and have at least one letter before the period. If it does not, print a warning and prompt again. Keep nagging the user until a proper filename is entered.• Prompt the user for 3 integers.o The three integers represent red, blue, and green channel values for a color.o Process the numbers according to the instructions belowo Once you have handled the 3 input values, prompt the user againo Keep prompting the user for 3 integers until the user enters the single word DONEo Reading input should not stop for any reason except for when the user enters DONE.o The user will not mix DONE and the numbers. The user will either enter 3 integers OR theword DONE• Once the user is done, open the filename entered in the first step and output the data.o If no numbers were entered, then do not open the file for writing. Simply print a messagethat no data was entered.o When 1 or more colors are entered, write out the XML data for each color. See below forthe format.o Sometimes users may enter integer values that are larger than 255 or less than 0. Valueslike this will need to be “clipped”, that is, converted to good values. For example, if the userenters 300 for red, then the actual red channel value will be 255. If the user entered -10 forthe blue channel, then the actual blue channel value will be 0.You should follow the sample below and your program should exhibit the same behavior.For full credit, your generated XML files must pass validation with NetBeans Check XML. XML FORMATThe very first line of the XML file must look exactly like this:<?xml version="1.0" encoding="utf-8" ?>After that line, the root element should be<quiz>For every number entered in the program there should be a<question>element child of the assignment element. The question element should have an attribute callednum. Its value is a number that should start at 1 for the first question, then 2 for the second question, andso on. The whole start tag for a question should follow this pattern:<question num="1">except the value for the attribute will be different.There should be a question element for each color (3 integers) the user entered. For example, if the userentered values for 4 colors during the input, then the XML file will have 4 question elements inside itsassignment element.Inside each question element, there will be four child elements:1. <data> - this will contain the values entered by the user. Inside <data>, there will be threemore elements: <red>, <green>, and <blue>. For example, if the user entered 255 for red, 0for green, and 0 for blue, then this element would be:<data><red>255</red><green>0</green><blue>0</blue></data>2. < rgb-int> - this will be the rgb version with three integers in the range of 0 - 2255. Forexample:<rgb-int> rgb(255, 0, 0) </rgb-int>3. < rgb-pct> - this will be the rgb version with three percentages. For example:<rgb-pct> rgb(100.0%, 0.0%, 0.0%) </rgb-pct>4. <hex> - this will be the color in hexadecimal format. For example:<hex> #ff0000 </hex>For example, suppose the fifth color the user entered the values 102 for red, 51 for green, and 255 for blue,then entire question element for that number would be:<question assign-num="5"><data><red>102</red><green>51</green><blue>255</blue></data><rgb-int> rgb(102, 51, 255) </rgb-int><rgb-pct> rgb(40.0%, 20.0%, 100.0%) </rgb-pct><hex> #6633ff </hex></question>As mentioned previously, the user may enter values for the color channels that are greater than 255 or lessthan 0. In that case, the values entered by the user should be shown in the <data> element as entered.The incorrect values should be clipped for the <rgb-int>, <rgb-pct>, and <hex> elements. Forexample, for the 6th question, if the user entered the values 300 for the red channel, 153 for the greenchannel, and -117 for the blue channel, the question element would be:<question assign-num="6"><data><red>300</red><green>153</green><blue>-117</blue></data><rgb-int> rgb(255, 153, 0) </rgb-int><rgb-pct> rgb(100.0%, 60.0%, 0.0%) </rgb-pct><hex> #ff9900 </hex></question>A full example is provided in the file sample.xml. SAMPLE RUNThe following is a sample run. Your program should have similar prompts and work the same. Make surethe greeting has your name. The blue, bold items are the values entered by the user.--- Welcome to Harry Potter's Color Quiz Answer Generator ---Enter the filename the data be saved tosample.xmlEnter 3 integers, or the word DONE to stop:25500Enter 3 integers, or the word DONE to stop:759942Enter 3 integers, or the word DONE to stop:999-88851Enter 3 integers, or the word DONE to stop:682555Enter 3 integers, or the word DONE to stop:
ASSIGNMENT
Write a Java program that meets these requirements.
• Create a NetBeans project named LastnameAssign1. Change Lastname to your last name. For example, my project would be named NicholsonAssign1.
• In the Java file, print a welcome message that includes your full name.
• The program should prompt for an XML filename to write to
o The filename entered must end with .xml and have at least one letter before the period. If it does not, print a warning and prompt again. Keep nagging the user until a proper filename is entered.
• Prompt the user for 3 integers.
o The three integers represent red, blue, and green channel values for a color.
o Process the numbers according to the instructions below
o Once you have handled the 3 input values, prompt the user again
o Keep prompting the user for 3 integers until the user enters the single word DONE
o Reading input should not stop for any reason except for when the user enters DONE.
o The user will not mix DONE and the numbers. The user will either enter 3 integers OR the
word DONE
• Once the user is done, open the filename entered in the first step and output the data.
o If no numbers were entered, then do not open the file for writing. Simply print a message
that no data was entered.
o When 1 or more colors are entered, write out the XML data for each color. See below for
the format.
o Sometimes users may enter integer values that are larger than 255 or less than 0. Values
like this will need to be “clipped”, that is, converted to good values. For example, if the user
enters 300 for red, then the actual red channel value will be 255. If the user entered -10 for
the blue channel, then the actual blue channel value will be 0.
You should follow the sample below and your program should exhibit the same behavior.
For full credit, your generated XML files must pass validation with NetBeans Check XML.
XML FORMAT
The very first line of the XML file must look exactly like this:
<?xml version="1.0" encoding="utf-8" ?>
After that line, the root element should be
<quiz>
For every number entered in the program there should be a
<question>
element child of the assignment element. The question element should have an attribute called
num. Its value is a number that should start at 1 for the first question, then 2 for the second question, and
so on. The whole start tag for a question should follow this pattern:
<question num="1">
except the value for the attribute will be different.
There should be a question element for each color (3 integers) the user entered. For example, if the user
entered values for 4 colors during the input, then the XML file will have 4 question elements inside its
assignment element.
Inside each question element, there will be four child elements:
1. <data> - this will contain the values entered by the user. Inside <data>, there will be three
more elements: <red>, <green>, and <blue>. For example, if the user entered 255 for red, 0
for green, and 0 for blue, then this element would be:
<data>
<red>255</red>
<green>0</green>
<blue>0</blue>
</data>
2. < rgb-int> - this will be the rgb version with three integers in the range of 0 - 2255. For
example:
<rgb-int> rgb(255, 0, 0) </rgb-int>
3. < rgb-pct> - this will be the rgb version with three percentages. For example:
<rgb-pct> rgb(100.0%, 0.0%, 0.0%) </rgb-pct>
4. <hex> - this will be the color in hexadecimal format. For example:
<hex> #ff0000 </hex>
For example, suppose the fifth color the user entered the values 102 for red, 51 for green, and 255 for blue,
then entire question element for that number would be:
<question assign-num="5">
<data>
<red>102</red>
<green>51</green>
<blue>255</blue>
</data>
<rgb-int> rgb(102, 51, 255) </rgb-int>
<rgb-pct> rgb(40.0%, 20.0%, 100.0%) </rgb-pct>
<hex> #6633ff </hex>
</question>
As mentioned previously, the user may enter values for the color channels that are greater than 255 or less
than 0. In that case, the values entered by the user should be shown in the <data> element as entered.
The incorrect values should be clipped for the <rgb-int>, <rgb-pct>, and <hex> elements. For
example, for the 6th question, if the user entered the values 300 for the red channel, 153 for the green
channel, and -117 for the blue channel, the question element would be:
<question assign-num="6">
<data>
<red>300</red>
<green>153</green>
<blue>-117</blue>
</data>
<rgb-int> rgb(255, 153, 0) </rgb-int>
<rgb-pct> rgb(100.0%, 60.0%, 0.0%) </rgb-pct>
<hex> #ff9900 </hex>
</question>
A full example is provided in the file sample.xml.
SAMPLE RUN
The following is a sample run. Your program should have similar prompts and work the same. Make sure
the greeting has your name. The blue, bold items are the values entered by the user.
--- Welcome to Harry Potter's Color Quiz Answer Generator ---
Enter the filename the data be saved to
sample.xml
Enter 3 integers, or the word DONE to stop:
255
0
0
Enter 3 integers, or the word DONE to stop:
75
99
42
Enter 3 integers, or the word DONE to stop:
999
-888
51
Enter 3 integers, or the word DONE to stop:
68
255
5
Enter 3 integers, or the word DONE to stop:

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

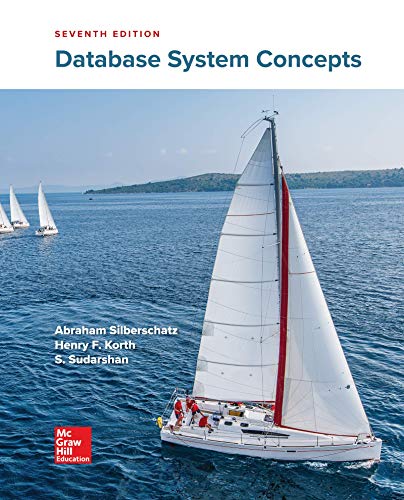
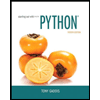
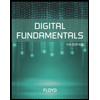
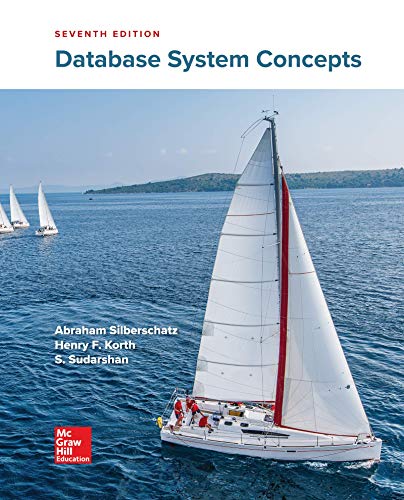
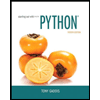
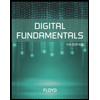
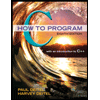
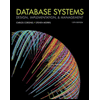
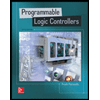