9.17 Lab 7: Dynamic Arrays Using Vectors Provide full code for main.cpp, dynamicarray.h and dynamic.cpp Step 1: Preparation For the moment, "comment out" the following under-construction code: dynamicarray.h: All function prototypes except the constructors and destructor. Keep the member variables for now. dynamicarray.cpp: All function implementations except the constructors and destructor. Remove (not just comment out) INITIAL_CAP, and replace it with a hard-coded 10 inside the default constructor. main.cpp: Comment out all the code inside the RunPart1Tests function between the lines. Comment out all the code starting with the "Equality comparison" comment and just before the "return 0;" line. bool pass = true; and return pass; Step 2: Replacing Member Data and the Two Constructors Replace the current member data (arr, len, and capacity) with a single vector of integers. At the top of your .h, include the library. Also, remove your current member data, and replace it with a vector of ints. Name it "arr" so you can keep as much of your current code as possible. Next, change the constructor and destructor. The implementation is now { }. You could remove the default constructor from the class but might as well be explicit For the copy constructor, copy the vector from the other object. One way to do so would be to do an element-by-element copy. The other is that we can use the copy constructor that's defined for the STL vector class through what is called a constructor initialization list. The new copy constructor: DynamicArray::DynamicArray(const DynamicArray& other) : arr(other.arr) { } The ": arr(other)" is the initialization list. It specifies how to construct each of the class's member variables and uses the copy constructor of the STL vector class. For destructor, deallocate any memory we dynamically allocated. The destructor is also now { }. The code should report the Lab 6 tests as "passed." Step 3: The One-Liners Plus Output Tackle four member functions (cap(), at(), append() and operator=) that translates well to functions that the vector class provides for you. You'll also tackle print. Uncomment the declarations and definitions of these functions from the .h and .cpp files. Replace your cap() function (defined in the .h) with a single-line function that uses a member function of the vector class. Do the same for your at() function. Do NOT return -11111 when out-of-bounds. It's OK if your code throws an exception (which will cause a crash) for out-of-bounds errors. Do the same for your append() function. Repeat for the = operator. This will be two lines: one to actually copy the vector, and then the obligatory return * this; Look at the output function: print(). It makes reference to two member variables: arr[i] and len. Replace these references with the appropriate ways to access them using your new vector. Go to the RunPart1Tests function in main.cpp. Put everything back in until the line labeled "Test sum." Two bad things are going to happen: You're going to fail two capacity tests. You can comment out the sections that test the capacity. THIS CODE SHOULD CRASH, with an e
9.17 Lab 7: Dynamic Arrays Using
Provide full code for main.cpp, dynamicarray.h and dynamic.cpp
Step 1: Preparation
For the moment, "comment out" the following under-construction code:
- dynamicarray.h: All function prototypes except the constructors and destructor. Keep the member variables for now.
- dynamicarray.cpp: All function implementations except the constructors and destructor. Remove (not just comment out) INITIAL_CAP, and replace it with a hard-coded 10 inside the default constructor.
- main.cpp: Comment out all the code inside the RunPart1Tests function between the lines. Comment out all the code starting with the "Equality comparison" comment and just before the "return 0;" line.
bool pass = true;
and
return pass;
Step 2: Replacing Member Data and the Two Constructors
Replace the current member data (arr, len, and capacity) with a single vector of integers.
- At the top of your .h, include the <vector> library. Also, remove your current member data, and replace it with a vector of ints. Name it "arr" so you can keep as much of your current code as possible.
Next, change the constructor and destructor.
- The implementation is now { }. You could remove the default constructor from the class but might as well be explicit
- For the copy constructor, copy the vector from the other object. One way to do so would be to do an element-by-element copy. The other is that we can use the copy constructor that's defined for the STL vector class through what is called a constructor initialization list. The new copy constructor:
DynamicArray::DynamicArray(const DynamicArray& other) : arr(other.arr) { }
The ": arr(other)" is the initialization list. It specifies how to construct each of the class's member variables and uses the copy constructor of the STL vector class.
- For destructor, deallocate any memory we dynamically allocated. The destructor is also now { }.
The code should report the Lab 6 tests as "passed."
Step 3: The One-Liners Plus Output
Tackle four member functions (cap(), at(), append() and operator=) that translates well to functions that the vector class provides for you. You'll also tackle print.
- Uncomment the declarations and definitions of these functions from the .h and .cpp files.
- Replace your cap() function (defined in the .h) with a single-line function that uses a member function of the vector class.
- Do the same for your at() function. Do NOT return -11111 when out-of-bounds. It's OK if your code throws an exception (which will cause a crash) for out-of-bounds errors.
- Do the same for your append() function.
- Repeat for the = operator. This will be two lines: one to actually copy the vector, and then the obligatory return * this;
Look at the output function: print(). It makes reference to two member variables: arr[i] and len. Replace these references with the appropriate ways to access them using your new vector.
Go to the RunPart1Tests function in main.cpp. Put everything back in until the line labeled "Test sum."
Two bad things are going to happen:
- You're going to fail two capacity tests. You can comment out the sections that test the capacity.
- THIS CODE SHOULD CRASH, with an error message including:
terminate called after throwing an instance of 'std::out_of_range: vector'
Step 4: The remaining functions
You should have three functions left.
sum
Steps:
- Un-comment it from dynamicarray.h
- In dynamicarray.cpp, make similar modifications to the ones you made for print()
- In main.cpp, put the "Test sum" section back into RunPart1Tests().
You should now be passing all the current tests.
operator==
The vector function doesn't have an equality operator, so your code still has to do the work of defining one.
- Uncomment it from your .h
- Modify the implementation as necessary
- In the main() function of main.cpp, put the Equality Comparison tests back in.
Recompile and rerun. Everything should pass.
remove
Last but not least; remove().
- Uncomment it from your .h
- Modify the implementation as follows
- Find valToDelete in the vector, and return false if it's not there.
- When you find it, you need to call vector's erase function and pass it an iterator for the position you want to delete.
vector.begin() + i where i is the position of the element. Then return true.
- In function RunPart1Tests() in main.cpp, put everything back except the capacity tests that's commented out, and the single (long) line of code under a.remove(100 + i). You should be passing all the Part 1 tests.
- In function main() in main.cpp, uncomment the rest of the code. All tests should pass with desired output.
Step 5: Overloading the output (<<) operator
Define and implement the output operator, which mirrors the print() method. Recall signature of this method is:
friend ostream& operator<< (ostream &out, const DynamicArray& objToPrint);
Replace the following lines at the end of main():
stringstream testoutput;
a.print(testoutput);
b.print(testoutput);
cout << testoutput.str();
with
cout << a << b;

Step by step
Solved in 4 steps with 1 images

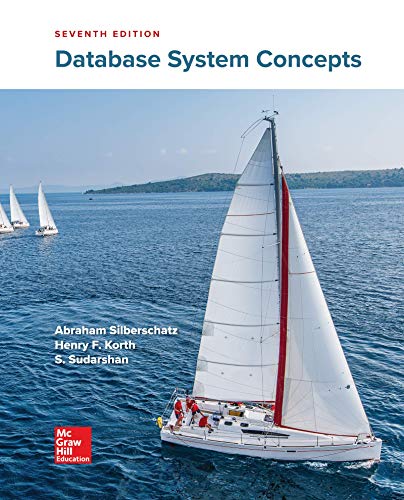
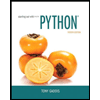
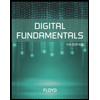
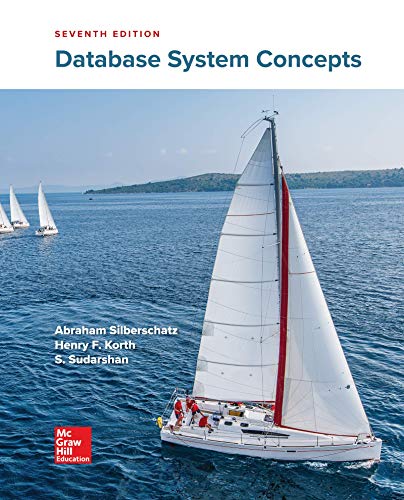
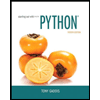
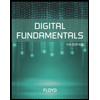
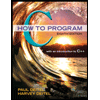
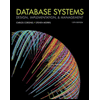
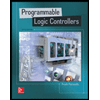