7.5 20 25.4 22.8 35 21.6 utput: Output: Output: Output: aint needed: Paint needed: Paint needed: Paint needed: 2.0 .142857142857143 0.48857142857142855 1.56754285714285 gallons allons gallons gallons Cans needed: 2.0 ans needed: 3.0 Cans needed: 1.0 Cans needed: 2.6
7.5 20 25.4 22.8 35 21.6 utput: Output: Output: Output: aint needed: Paint needed: Paint needed: Paint needed: 2.0 .142857142857143 0.48857142857142855 1.56754285714285 gallons allons gallons gallons Cans needed: 2.0 ans needed: 3.0 Cans needed: 1.0 Cans needed: 2.6
Chapter6: System Integration And Performance
Section: Chapter Questions
Problem 2PE
Related questions
Question
I need help with this question in my intro to Java class. I have included what I have so far but I am not getting what I should be and dont know what I am doing wrong.

Transcribed Image Text:Input:
Input:
Input:
Input:
30
7.5
20
25.4
25
22.8
35
21.6
Output:
Output:
Output:
Output:
Paint needed:
Paint needed:
Paint needed:
Paint needed: 2.0
2.142857142857143
0.48857142857142855
1.5675428571428571
gallons
gallons
gallons
gallons
Cans needed: 2.0
Cans needed: 3.0
Cans needed: 1.0
Cans needed: 2.0
can(s)
can(s)
can(s)
can(s)
1. First, you will select a method that appropriately handles all of the included test cases by consulting the official Java documentation for the
Math class. Use the following tips to help you navigate the documentation:
• Scroll to the Method Summary section of the Math class and review the methods and their descriptions. Look for a method that will help
you round a value up to the nearest integer.
o If a method looks promising, click on its name to see a more detailed description. Pay special attention to the argument(s) and the data
type of the return value.
• Based on your review, select one or more methods from the Math class to use in your program.
2. Open the Virtual Lab by clicking on the link in the Virtual Lab Access module. Then open your IDE and upload the Paint2.zip folder. Review
the code for the Paint2.java class, looking for the comment //Complete this code block. Here, you will implement your selected method
so that your program meets the required functionality. The program should do the following:
• Calculate the number of paint cans needed to paint the wall.
• Round up to the nearest integer. Use the test cases to check your work.
Output the number of cans needed for the user.
![1 import java.util.Scanner;
2
3 public class Paint2 {
public static void main (String [] args) {
Scanner scnr = new Scanner (System.in);
double wallHeight
= 0.0;
9
double wallWidth = 0.0;
10
11
double wallArea = 0.0;
double gallonsPaintNeeded = 0.0;
12
double cansNeeded;
13
14
15
16
17
18
final
ouble squareFeetPerGallons = 350.0;
final double gallonsPerCan = l.0;
// Prompt user to input wall's height
System.out.println ("Enter wall height (feet): ");
19
20
21
22
23
24
25
26
27
wallHeight = scnr.nextDouble ();
// Prompt user to input wall's width
System.out.println ("Enter wall width (feet): ");
wallWidth - scnr.nextDouble () :
// Calculate and output wall area
wallArea = wallHeight * wallWidth;
28
29
30
31
32
System.out.println ("Wall area:
+ wallArea +
"square feet");
// Calculate and output the amount of paint in gallons needed to paint the wall
gallonsPaintNeeded = wallArea / squareFeetPerGallons;
System.out.println ("Paint needed:
" + gallonsPaintNeeded + " gallons"):
33
34
// Calculate and output the number of paint cans needed to paint the wall,
35
// rounded up to nearest integer
// Complete this code block
cansNeeded = wallArea / gallonsPaintNeeded;
38
System.out.println ("Cans needed: " + Math.ceil (cansNeeded) + "can (s) ");
39
40 }
41
333 34](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fd9fa295a-90dd-4ec6-950d-22c80fce920e%2F3b550624-b18f-4f04-8ddf-1888ac6a4a0b%2Frs697z_processed.png&w=3840&q=75)
Transcribed Image Text:1 import java.util.Scanner;
2
3 public class Paint2 {
public static void main (String [] args) {
Scanner scnr = new Scanner (System.in);
double wallHeight
= 0.0;
9
double wallWidth = 0.0;
10
11
double wallArea = 0.0;
double gallonsPaintNeeded = 0.0;
12
double cansNeeded;
13
14
15
16
17
18
final
ouble squareFeetPerGallons = 350.0;
final double gallonsPerCan = l.0;
// Prompt user to input wall's height
System.out.println ("Enter wall height (feet): ");
19
20
21
22
23
24
25
26
27
wallHeight = scnr.nextDouble ();
// Prompt user to input wall's width
System.out.println ("Enter wall width (feet): ");
wallWidth - scnr.nextDouble () :
// Calculate and output wall area
wallArea = wallHeight * wallWidth;
28
29
30
31
32
System.out.println ("Wall area:
+ wallArea +
"square feet");
// Calculate and output the amount of paint in gallons needed to paint the wall
gallonsPaintNeeded = wallArea / squareFeetPerGallons;
System.out.println ("Paint needed:
" + gallonsPaintNeeded + " gallons"):
33
34
// Calculate and output the number of paint cans needed to paint the wall,
35
// rounded up to nearest integer
// Complete this code block
cansNeeded = wallArea / gallonsPaintNeeded;
38
System.out.println ("Cans needed: " + Math.ceil (cansNeeded) + "can (s) ");
39
40 }
41
333 34
Expert Solution

Step 1: Algorithm
Step 1 : START
Step 2 : declare all variables and initialize all of them
Step 3 : enter wall height
Step 4 : enter wall width
Step 5 : calculate and output area
Step 6 : calculate and output the gallons paint needed based on the value of the area
Step 7 : calculate and output the cans needed based on the gallons paint calculated
Step 8 : END
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
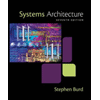
Systems Architecture
Computer Science
ISBN:
9781305080195
Author:
Stephen D. Burd
Publisher:
Cengage Learning
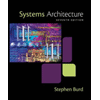
Systems Architecture
Computer Science
ISBN:
9781305080195
Author:
Stephen D. Burd
Publisher:
Cengage Learning