2. Case Problems 1 (three parts) - a - Carly’s Catering provides meals for parties and special events. In Chapter 2, you wrote an application that prompts the user for the number of guests attending an event, displays the company motto with a border, and then displays the price of the event and whether the event is a large one. Now modify the program so that the main() method contains only three executable statements that each call a method as follows: The first executable statement calls a public static int method that prompts the user for the number of guests and returns the value to the main() method. The second executable statement calls a public static void method that displays the company motto with the border. The last executable statement passes the number of guests to a public static void method that computes the price of the event, displays the price, and displays whether the event is a large event. Save the file as CarlysEventPriceWithMethods.java. b. Create a class to hold Event data for Carly’s Catering. The class contains: Two public final static fields that hold the price per guest ($35) and the cutoff value for a large event (50 guests) Three private fields that hold an event number, number of guests for the event, and the price. The event number is stored as a String because Carly plans to assign event numbers such as M312. Two public set methods that set the event number (setEventNumber()) and the number of guests (setGuests()). The price does not have a set method because the setGuests() method will calculate the price as the number of guests multiplied by the price per guest every time the number of guests is set. Three public get methods that return the values in the three nonstatic fields. Save the file as Event.java. Use the CarlysEventPriceWithMethods class you created in Step 1a as a starting point for a program that demonstrates the Event class you created in Step 1b, but make the following changes: c. You already have a method that gets a number of guests from a user; now add a method that gets an event number. The main() method should declare an Event object, call the two data entry methods, and use their returned values to set the fields in the Event object. Call the method from the CarlysEventPriceWithMethods class that displays the company motto with the border. The method is accessible because it is public, but you must fully qualify the name because it is in another class. Revise the method that displays the event details so that it accepts the newly created Event object. The method should display the event number, and it should still display the number of guests, the price per guest, the total price, and whether the event is a large event. Save the program as EventDemo.java.

Algorithm :
1. Create a class named CarlysEventPriceWithMethods.
2. In the main method, declare an integer variable to store the number of guests.
3. Call the getNumOfGuests() method to get the number of guests from user input.
4. Call the displayMottoWithBorder() method to display the motto of the event.
5. Call the displayEventDetails(numOfGuests) method to display the event details.
6. Create a getNumOfGuests() method to get the number of guests from user input.
7. Create a displayMottoWithBorder() method to display the motto of the event.
8. Create a display event details(int numOfGuests) method to display the event details.
9. Create a class named Event
10. Declare static variables for the price per guest and the cutoff value for large events.
11. Declare instance variables for the event number, number of guests, and price.
12. Create setEventNumber() and setGuests() methods to set the event number and the number of guests.
13. Create getEventNumber(), getNumOfGuests(), and getPrice() methods to get the event number, number of guests, and price.
14. Create a class named EventDemo.
15. In the main method, declare an Event object and assign it to a variable.
16. Call the getNumOfGuests() method to get the number of guests from user input.
17. Call the getEventNumber() method to get the event number from the user input.
18. Call the setEventNumber() and setGuests() methods to set the event number and the number of guests.
19. Call the CarlysEventPriceWithMethods.displayMottoWithBorder() method to display the motto of the event.
20. Call the display event details(Event myEvent) method to display the event details.
21. Create a getNumOfGuests() method to get the number of guests from user input.
22. Create a getEventNumber() method to get the event number from the user input.
23. Create a display event details(Event myEvent) method to display the event details.
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 5 images

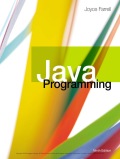
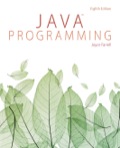
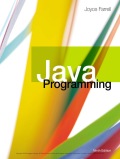
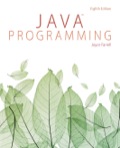
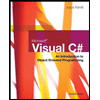