1. created a p07 2. copied .c .h and makefile 3. renamed main.c to sort158a.c 4. modified the header title 5. updated the make file to: #Create binary sort158: main.o merge.o mergesort.o wrt.o gcc -o sort158 main.o merge.o mergesort.o wrt.o -lm #Create object file for main.c main.o: main.c mergesort.h gcc -c main.c #Create object file for merge.c merge.o: merge.c mergesort.h gcc -c merge.c #Create object file for mergesort.c mergesort.o: mergesort.c mergesort.h gcc -c mergesort.c wrt.o: wrt.c gcc -c wrt.c #Delete the all of the object files clean: rm *.o sort158 #Create object file for wrt.c 6. Error: The makefile wont compile 7. Error: I need to make the files to compile with eachother sort158a.c (main.c) #include "mergesort.h" int main(void) { int sz, key[] = { 4, 3, 1, 67, 55, 8, 0, 4, -5, 37, 7, 4, 2, 9, 1, -1 }; int n = sizeof(key) / sizeof(int); /* the size of key [] */ int i; /*remove first 4 elements from key [] */ for(i = 4; i < n; i++) { key[ i - 4] = key[i]; } sz = n - 4; /* Update the size of key [] */ wrt(key, sz); /* Print the contents of the orginal array */ mergesort(key, sz); /* Sort the array using mergesort */ printf("After mergesort:\n"); /*prints "After mergesort:"*/ wrt(key, sz); // Print the contents of the sorted array /* Restore first 3 elements in key[] */ key[0] = 4; key[1] = 3; key[2] = 1; key[3] = 67; sz = n; return 0; } Merge.c #include "mergesort.h" void merge(int a[], int b[], int c[], int m, int n) { int i = 0, j = 0, k = 0; /* Loop through both arrays and compare their elements to eachother */ while (i < m && j < n) if (a[i] < b[j]) c[k++] = a[i++]; else c[k++] = b[j++]; /* If there are any remaining elements in array b[]. copy them to array c[]. */ while (i < m) /* pick up any remainder */ c[k++] = a[i++]; /* If there are any remaining elements in array a[], copy them to array c[]. */ while (j < n) c[k++] = b[j++]; } mergesort.h #include "mergesort.h" void mergesort(int key[], int n) { int j, k, m, *w; // Find the largest power of 2 less than or equal to n for (m = 1; m < n; m *= 2) /* m is a power of 2 */ ; // Check if n is a power of 2, if not exit with error message if (n < m) { printf("ERROR: Array size not a power of 2 - bye!\n"); exit(1); } w = calloc(n, sizeof(int)); /* allocate workspace */ assert(w != NULL); /* check that calloc() worked */ //Sort the array using mergesort for (k = 1; k < n; k *= 2) { for (j = 0; j < n - k; j += 2 * k) /* merge two subarrays of key [] into a subarray of w[]. */ merge(key + j, key + j + k, w + j, k, k); for (j = 0; j < n; j++) key[j] = w[j]; /* write W back into key */ } free (w); /*free the workspace*/ } Mergesort.h #include #include #include // This function merges two sorted arrys a and b into a sorted array c. // paterameters: a - c sorts its array. m: is the elements in a n: is the number of elements in b void merge(int a[], int b[], int c[], int m, int n); //This function sorts an array of integers using the merge sort algorithm //key: the array of integers to be sorted //n: the number of elements in the array void mergesort(int key[], int n); //This function writes an array of integers //key: the array of integers to be written //sz: the number of elements in the array void wrt(int key [], int sz); wrt.c
1. created a p07
2. copied .c .h and makefile
3. renamed main.c to sort158a.c
4. modified the header title
5. updated the make file to:
#Create binary
sort158: main.o merge.o mergesort.o wrt.o
gcc -o sort158 main.o merge.o mergesort.o wrt.o -lm
#Create object file for main.c
main.o: main.c mergesort.h
gcc -c main.c
#Create object file for merge.c
merge.o: merge.c mergesort.h
gcc -c merge.c
#Create object file for mergesort.c
mergesort.o: mergesort.c mergesort.h
gcc -c mergesort.c
wrt.o: wrt.c
gcc -c wrt.c
#Delete the all of the object files
clean:
rm *.o sort158
#Create object file for wrt.c
6. Error: The makefile wont compile
7. Error: I need to make the files to compile with eachother
sort158a.c (main.c)
#include "mergesort.h"
int main(void)
{
int sz, key[] = { 4, 3, 1, 67, 55, 8, 0, 4,
-5, 37, 7, 4, 2, 9, 1, -1 };
int n = sizeof(key) / sizeof(int); /* the size of key [] */
int i;
/*remove first 4 elements from key [] */
for(i = 4; i < n; i++) {
key[ i - 4] = key[i];
}
sz = n - 4; /* Update the size of key [] */
wrt(key, sz); /* Print the contents of the orginal array */
mergesort(key, sz); /* Sort the array using mergesort */
printf("After mergesort:\n"); /*prints "After mergesort:"*/
wrt(key, sz); // Print the contents of the sorted array
/* Restore first 3 elements in key[] */
key[0] = 4;
key[1] = 3;
key[2] = 1;
key[3] = 67;
sz = n;
return 0;
}
Merge.c
#include "mergesort.h"
void merge(int a[], int b[], int c[], int m, int n)
{
int i = 0, j = 0, k = 0;
/* Loop through both arrays and compare their elements to eachother */
while (i < m && j < n)
if (a[i] < b[j])
c[k++] = a[i++];
else
c[k++] = b[j++];
/* If there are any remaining elements in array b[]. copy them to array c[]. */
while (i < m) /* pick up any remainder */
c[k++] = a[i++];
/* If there are any remaining elements in array a[], copy them to array c[]. */
while (j < n)
c[k++] = b[j++];
}
mergesort.h
#include "mergesort.h"
void mergesort(int key[], int n)
{
int j, k, m, *w;
// Find the largest power of 2 less than or equal to n
for (m = 1; m < n; m *= 2) /* m is a power of 2 */
;
// Check if n is a power of 2, if not exit with error message
if (n < m) {
printf("ERROR: Array size not a power of 2 - bye!\n");
exit(1);
}
w = calloc(n, sizeof(int)); /* allocate workspace */
assert(w != NULL); /* check that calloc() worked */
//Sort the array using mergesort
for (k = 1; k < n; k *= 2) {
for (j = 0; j < n - k; j += 2 * k)
/* merge two subarrays of key [] into a subarray of w[]. */
merge(key + j, key + j + k, w + j, k, k);
for (j = 0; j < n; j++)
key[j] = w[j]; /* write W back into key */
}
free (w); /*free the workspace*/
}
Mergesort.h
#include <assert.h>
#include <stdio.h>
#include <stdlib.h>
// This function merges two sorted arrys a and b into a sorted array c.
// paterameters: a - c sorts its array. m: is the elements in a n: is the number of elements in b
void merge(int a[], int b[], int c[], int m, int n);
//This function sorts an array of integers using the merge sort
//key: the array of integers to be sorted
//n: the number of elements in the array
void mergesort(int key[], int n);
//This function writes an array of integers
//key: the array of integers to be written
//sz: the number of elements in the array
void wrt(int key [], int sz);
wrt.c

![Program Name
sort158a
Description
The program will sort any number of integers for lowest to highest.
The program will use the format and words displayed in the Example of Output.
The program will use the following files:
sort158a.c
merge.c
mergesort.c
mergesort.h
Requirements
All the module are files from the class textbook "A Book on C", 6.9 An Example: of
Merge and Merge Sort, Pages 263-269. You should have already entered these modules on
CISWEB as part of assignment A07 and will be in directory a07. If you have not done
assignment A07, do it NOW! Source code file main.c will become sort158a.c.
To begin do the following:
wrt.c
1. Create the p07 directory as required and go to it.
2. Copy all the .c and .h files as well as the makefile from a07
3. Rename main.c to sort158a.c
4. Go into sort158a.c and change the title. Make sure to indicate that this is a modification
of main.c from "A Book on C"
5. Update the makefile to reflect the new filenames.
6. Compile the program using the make command and verify that no errors or warnings
occur. If they do, make the appropriate corrections.
7. Validate that sort158a works properly. If it does not make corrections as needed.
As written in the text and A07, the program will sort integers. However, the number of
integers must be a power of 2. In main.c, key[ ] has 16 integers. But as you should already
know, the program will not sort if one integers is removed or added.
What you are going to do is modify the mergesort.c module so that a non-power of 2
number of integers can be sorted. When you modify a module, indicate by way of
comment, what was changed, the date the change was made. You will also need to add or
remove an integer to/from key[] in module sort158a.c.
You are NOT allowed to change merge.c, mergesort.h or wrt.c files
The mergesort function in file mergesort.c MUST call the merge function which it currently does.
The main function in sort158a.e you can only change the number of integers in the key[] array.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F9f50000c-ff84-4cf9-9ace-590e65be4826%2F26566338-f5e9-4c2f-bd56-1ffeba4b323f%2F225rdxp_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 5 steps

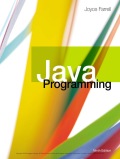
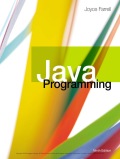