Recursion exercise
.docx
keyboard_arrow_up
School
Northeastern University *
*We aren’t endorsed by this school
Course
1285
Subject
Computer Science
Date
May 7, 2024
Type
docx
Pages
4
Uploaded by AmbassadorSnow13278 on coursehero.com
Introduction
This activity explores recursive way to solve problems. To solve a large problem, we use the solution of a smaller but similar problem. Eventually, we reach the most basic problem, for which we know the answer. Complete the worksheet to the best of your ability. You’re encourage to work with partner(s) but each of you should be filing your own worksheet. If you get stuck with some question, move on to the next question – maybe this will shed the light on the previous question too. Part 1 Factorial: Critical Thinking Questions
1. Consider two different ways to show how to calculate 4! (the product of the numbers from 1 to 4).
a) Write out all numbers
that explicitly needed to be multiplied
4! = 1 * 2 * 3 * 4
b) Write the expression using 3! 4! = 3! * 4
2. Write an expression similar to question 1 showing how each factorial can be calculated in terms of a “simpler” factorial. (By definition, 0! equals 1.)
a) 3! = 2! * 3
b) 2! = 1! * 2
c) 1! = 0! * 1
d) 100! = 99! * 100
3. Generalize your group’s answer to question 2 in terms of n
: write down a formula to compute n! using factorial of a smaller number.
n! = (n-1) * n
1
4. When faced with a large problem to solve, we might seek to use a solution to a smaller, simpler problem. If we repeatedly decompose the original problem into smaller simpler expressions, we will eventually identify the most simple or basic component that can’t be broken down any further. This is referred to as the base case
. What would your group propose
to be the base case
for factorial computation? I.e. what is the smallest number for which factorial can be easily computed (and cannot be represented by the above formula)? The base case is when we calculate the 0! Since that is equal to 1 as mentioned above
Part 2 Analyzing recursive code
When a function makes a call to itself this is referred to as recursion
. To define a recursive function, you should write an if-statement that checks for the base case. When the operation is not
the base case, you include a call to the function you are writing (known as the recursive call
).
Consider the following code for factorial computation:
’’’calculates n factorial’’’
def factorial(n):
if n == 0:
return 1
else:
print(n)
return n * factorial(n-1)
Critical Thinking Questions
1. Underline the recursive call in the above code.
2. What happens if you try to calculate the factorial of a negative number? Fix the bug in the factorial method so this does not occur. It would go on forever so you should add a condition that breaks out the loop if n < 0
If n< 0:
print(“Cannot find the factorial of negative numbers”)
break
3. How many distinct calls are made to the factorial() function to calculate the factorial of 3? Identify the value of the parameter n for each of these separate calls.
2
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
Personal project Q5.
This question is concerned with the design and analysis of recursive algorithms.
You are given a problem statement as shown below. This problem is concerned with performing calculations on a sequence A of real numbers. Whilst this could be done using a conventional loop-based approach, your answer must be developed using a recursive algorithm. No marks will be given if your answer uses loops.
FindAverageAndProduct(a1, ...., an) such that n > 1
Input: A sequence of real values A = (a1, ...., an) Output:, A 2-tuple (average, product) containing the average (average) of all the values and the product (product) of all the values of the elements in A.
Your recursive algorithm should use a single recursive structure to find the average and product values, and should not use two separate instances of a recursive design. You should not employ any global variables.
(a) Produce a pseudo code design for a recursive algorithm to solve this problem.
(b) Draw a call-stack…
arrow_forward
Investigate both the iterative and the recursive methods of problem resolution, and then compare and contrast the results of your research. When would you use iteration when recursion would be more appropriate, and when would you use recursion when iteration would be more appropriate? Your answer should be justified by giving examples that are different from those that are shown on the slides of the presentation.
arrow_forward
Question 5
When writing a recursive method,
O you do not need to know ahead of time exactly how many levels of recursion will occur.
O you must keep count of how many recursion call levels you have traversed.
O you must make sure the method does not take any input parameters.
arrow_forward
Computer Science
When you are working in an organization, there will be different ways to communicate with your colleagues.
based on this give at least 4 methods for team communication (one of the methods must be unique and creative way of communication.
Using your own words, specify what type of information can be shared using the suggested method.
arrow_forward
Personal project Q5.
This question is concerned with the design and analysis of recursive algorithms.
You are given a problem statement as shown below. This problem is concerned with performing calculations on a sequence ? of real numbers. Whilst this could be done using a conventional loop-based approach, your answer must be developed using a recursive algorithm. No marks will be given if your answer uses loops.
FindAverageAndProduct(a1, ...., an) such that n > 1
Input: A sequence of real values A = (a1, ..., an) Output:, A 2-tuple (average, product) containing the average (average) of all the values and the product (product) of all the values of the elements in A.
Your recursive algorithm should use a single recursive structure to find the average and product values, and should not use two separate instances of a recursive design. You should not employ any global variables.
(a) Produce a pseudo code design for a recursive algorithm to solve this problem.
(b) Draw a call-stack…
arrow_forward
Artificial Intelligence (Part - 1)
====================
The Towers of Hanoi is a famous problem for studying recursion in computer science and searching in artificial intelligence. We start with N discs of varying sizes on a peg (stacked in order according to size), and two empty pegs. We are allowed to move a disc from one peg to another, but we are never allowed to move a larger disc on top of a smaller disc. The goal is to move all the discs to the rightmost peg (see figure). To solve the problem by using search methods, we need first formulate the problem. Supposing there are K pegs and N disk.
(1) Propose a state representation for the problem?
arrow_forward
In order to accomplish the task of terminating recursion, you must first describe three distinct types of recursion, a high-level description of each kind, and a specific technique that fits into each category.
arrow_forward
Artificial Intelligence (Part - 2)
====================
The Towers of Hanoi is a famous problem for studying recursion incomputer science and searching in artificial intelligence. We start with N discs of varying sizes on a peg (stacked in order according to size), and two empty pegs. We are allowed to move a disc from one peg to another, but we are never allowed to move a larger disc on top of a smaller disc. The goal is to move all the discs to the rightmost peg (see figure). To solve the problem by using search methods, we need first formulate the problem. Supposing there are K pegs and N disk.
(2) What is the size of the state space?
arrow_forward
Ex. 01 : Recursion
About
So far, we have learned that we can perform repetitive tasks using loops. However, another way is by creating methods that call themselves. This programming technique is called recursion
and, a method that calls itself within it's own body is called a recursive method. One use of recursion is to perform repetitive tasks instead of using loops, since some problems seem to be
solved more naturally with recursion than with loops.
To solve a problem using recursion, it is broken down into sub-problems. Each sub-problem is similar to the original problem, but smaller in size. You can apply the same approach to each
sub-problem to solve it recursively.
All recursive methods use conditional tests to either
1. stop or
2. continue the recursion.
Each recursive method has the following characteristics:
1. end/terminating case: One or more end cases to stop the recursion.
2. recursive case: reduces the problem in to smaller sub-problems, until it reaches (becomes) the end…
arrow_forward
Programming is communication; the programmer “explains” to a computer how to carry out a task, with the explanation being the program. Can you think of any cases where communication directed to people uses direct or indirect recursion? Are there cases where such a use of recursion is indispensable?
arrow_forward
In a recursive algorithm, the goal is to solve a problem by breaking it into smaller sub-problems to solve. This is accomplished by constructing an algorithm using two important components. What are these components, what is the purpose of each?
arrow_forward
Task 1
Count the number of vowels in a phrase using recursion only. You can think of this problem as
"I can count the number of vowels in this phrase by counting the number of vowels in the first
character, then counting the number of vowels in the rest of the phrase." We define a vowel as
being A, E, I, O or U.
This is one of those problems that can just as easily be solved with traditional programming
structures such as a loop - but we're asking you to use recursion for the exercise.
Consider: what replaces the loop structure? When will we stop recursion?
Task 2
The constant e (Euler's number) is approximated by the following sequence:
1₁
n!
where the number of terms in the sequence is sufficient to generate the required precision in
decimal places.
1+
2!
+
...
We say that there is some value epsilon (e) such that, when the change in the approximation
from one term to the next is less than said epsilon, we have reached sufficient precision. That
is, when the term you're proposing to…
arrow_forward
Part C: Function, for and plotting
We did a project in the lecture on calculating the free fall speeds and plotting them on a graph. This part is similar to the project.
An engineer has derived a relationship between the force applied to a material and the extension in length that the force would cause. The relationship between force f and extension e is given by:
You are asked to plot a graph showing the relationship between force and extension. You are asked to complete the following tasks:
Task 1
Write a Python function which returns the value of e for a given input f. Do not use literals (e.g. 5.5, 10) in the expressions for e in the function. Instead you should define constants and use them.
Note that the relationship between e and f depends on whether f is bigger than 10 or not, this means you need a certain Python construction in your function. If you can't think of that, have a look at Part A of Lab03.
arrow_forward
1. The entrance room (or the starting of the maze) is considered as level
1. Now, answer these following questions: (a). Write an algorithm to
figure out how many maximum levels the maze can go up to. (b). Figure
out the complexity of your algorithm.
To create a maze some rooms of a building is connected. Starting room is called Entrance room. From
the entrance room other rooms are there connected from it. However, some rooms of that maze-
building have connected room from it, and some rooms do not have any connected room. Each of the
room can have at most or up to two rooms connected from it. The starting room is the entrance room of
the maze or building. Fore example: It can be any one like the followings:
Exemple -:
Room1
Roono
Room
Entrance
Room Raom
Room2
Room?
Roo
Roomo
Here, maxinum level =7
Example -2;
Entrace
Room D-
Room5
Room 2
Room 4
Maxximum level=3
arrow_forward
Do not use static variables to implement recursive methods.
USING JAVA
1.
Using Big Oh notation, indicate the time requirement for each of the following tasks in the worst case. Describe which operations are assumed to take constant time to.
After arriving at a party, you shake hands with each person there. n is the number of persons in the party.
Each person in a room shakes hands with everyone else in the room. n is the number of persons in the room.
You climb a flight of stairs. n is the number of stairs
After entering an elevator, you press a button to choose a floor. n is the number of floors
You ride the elevator from the ground floor up to the nth floor.
You read a book twice. n is the number of pages in the book
Using Big Oh notation, indicate the time requirement of each of the following tasks in the worst case.
Display all the integers in an array of integers.
Display all the integers in a chain of linked nodes.
Display the nth integer in an array of integers.
Compute…
arrow_forward
Recursion is the best and the fastest way to solve any problem.True or False
arrow_forward
Backtracking normally uses ______ to find out all solutions to a computational problem. (recursion, iteration).
arrow_forward
DISCRETE STRUCTURE
A game is played by moving a marker ahead either 2 or 3 steps on a linear path. Let cn be the number of different ways a path of length n can be covered. Given, Cn =Cn-2 + Cn-3, Ci=0, c2=1, c3=1 Write a recursive algorithm to compute Cn.
arrow_forward
Design and implement a recursive program that solves the Nonattacking Queens problem. That is, write a program to determine how eight queens can be positioned on an eight-by-eight chessboard so that none of them is in the same row, column, or diagonal as any other queen. There are no other chess pieces on the board.
Design and implement a recursive program that solves the Nonattacking Queens problem. That is, write a program to determine how eight queens can be positioned on an eight-by-eight chessboard so that none of them is in the same row, column, or diagonal as any other queen. There are no other chess pieces on the board.
Design and implement a recursive program that solves the Nonattacking Queens problem. That is, write a program to determine how eight queens can be positioned on an eight-by-eight chessboard so that none of them is in the same row, column, or diagonal as any other queen. There are no other chess pieces on the board.
arrow_forward
write a recursive method to schedule compatible activities that result in the maximum usage of the room.
arrow_forward
When recursion is used to solve a problem, why must the recursive method call itself to solve a smaller version of the original problem?
arrow_forward
Compare and contrast between iterative and recursive solutions. When would you preferiteration over recursion and vice-versa? Justify your answer by giving different examples than the ones which are provided in the lecture slides
java code
arrow_forward
Pascal's triangle is a useful recursive definition that tells us the coefficients in the expansion of the polynomial (x + a)^n. Each element in the triangle has a coordinate, given by the row it is on and its position in the row (which you could call a column). Every number in Pascals triangle is defined as the sum of the item above it and the item above it and to the left. If there is a position that does not have an entry, we treat it as if we had a 0 there.
*picture of the pascals triangle*
Given the following recursive function signature, write the recursive function that takes a row and a column and finds the value at that position in the triangle. Assume that the triangle starts at row 0 and column 0.
Examples: pascal(2, 1) -> 2, pascal(1, 2) -> 0
public int pascal(int row, int column) {
}
arrow_forward
What are the basic components required to create a recursive method? How can you avoid creating an infinitely recursive method? In your post, also provide an example of how recursion can be used in a specific example.
arrow_forward
Question-1
Friend's Party Circle:
There are a few friends living in the same area.
They have a party every weekend and the place
of party change each week. It is always a
lifficult task to select a place which is nearest
for everyone. They
advantage of Computer Science to solve this
problem.
all decided to take
Names of friends are Ahmed, Rehman, Careem,
Basit, Dawood, Ghani, and Farid. Ahmed lives
at 5 minutes' walk from rehman and at 10
minutes' walk from Careem. Careem lives at 3
minutes' walk from Dawood. Rehman lives at 4
minutes' walk from Basit and 2 minutes' walk
from Dawood. Dawood lives at two minutes'
walk from Farid. Ghani lives at 2 minutes' walk
from Basit.
a. If we represent a graph G = V (V, E) in
which set of vertices are home of each
Friend and an edge represents a path
between
two
homes.
Provide the
adjacency matrix of directed graph of
the graph G.
b. In above directed graph G. You are
required to devise an algorithm to find
all possible paths.
arrow_forward
When an algorithm that normally recursively calls itself will no longer do so, what are the conditions that must be met?
arrow_forward
For each of the following recursive methods, identify the base case, the general case, and the constraints on the argument values, and explain what the method does. (images below)
arrow_forward
Only correct answer will be appreciated else downvoted.
arrow_forward
Please draw the working of this semaphore. If you don't know please skip it:
arrow_forward
Logic Desgin
Take your time in answering the questions.
I want to solve questions ( f ) correctly please.
arrow_forward
Any simple task may be aided by the Spiral Model.
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
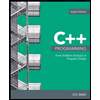
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
Related Questions
- Personal project Q5. This question is concerned with the design and analysis of recursive algorithms. You are given a problem statement as shown below. This problem is concerned with performing calculations on a sequence A of real numbers. Whilst this could be done using a conventional loop-based approach, your answer must be developed using a recursive algorithm. No marks will be given if your answer uses loops. FindAverageAndProduct(a1, ...., an) such that n > 1 Input: A sequence of real values A = (a1, ...., an) Output:, A 2-tuple (average, product) containing the average (average) of all the values and the product (product) of all the values of the elements in A. Your recursive algorithm should use a single recursive structure to find the average and product values, and should not use two separate instances of a recursive design. You should not employ any global variables. (a) Produce a pseudo code design for a recursive algorithm to solve this problem. (b) Draw a call-stack…arrow_forwardInvestigate both the iterative and the recursive methods of problem resolution, and then compare and contrast the results of your research. When would you use iteration when recursion would be more appropriate, and when would you use recursion when iteration would be more appropriate? Your answer should be justified by giving examples that are different from those that are shown on the slides of the presentation.arrow_forwardQuestion 5 When writing a recursive method, O you do not need to know ahead of time exactly how many levels of recursion will occur. O you must keep count of how many recursion call levels you have traversed. O you must make sure the method does not take any input parameters.arrow_forward
- Computer Science When you are working in an organization, there will be different ways to communicate with your colleagues. based on this give at least 4 methods for team communication (one of the methods must be unique and creative way of communication. Using your own words, specify what type of information can be shared using the suggested method.arrow_forwardPersonal project Q5. This question is concerned with the design and analysis of recursive algorithms. You are given a problem statement as shown below. This problem is concerned with performing calculations on a sequence ? of real numbers. Whilst this could be done using a conventional loop-based approach, your answer must be developed using a recursive algorithm. No marks will be given if your answer uses loops. FindAverageAndProduct(a1, ...., an) such that n > 1 Input: A sequence of real values A = (a1, ..., an) Output:, A 2-tuple (average, product) containing the average (average) of all the values and the product (product) of all the values of the elements in A. Your recursive algorithm should use a single recursive structure to find the average and product values, and should not use two separate instances of a recursive design. You should not employ any global variables. (a) Produce a pseudo code design for a recursive algorithm to solve this problem. (b) Draw a call-stack…arrow_forwardArtificial Intelligence (Part - 1) ==================== The Towers of Hanoi is a famous problem for studying recursion in computer science and searching in artificial intelligence. We start with N discs of varying sizes on a peg (stacked in order according to size), and two empty pegs. We are allowed to move a disc from one peg to another, but we are never allowed to move a larger disc on top of a smaller disc. The goal is to move all the discs to the rightmost peg (see figure). To solve the problem by using search methods, we need first formulate the problem. Supposing there are K pegs and N disk. (1) Propose a state representation for the problem?arrow_forward
- In order to accomplish the task of terminating recursion, you must first describe three distinct types of recursion, a high-level description of each kind, and a specific technique that fits into each category.arrow_forwardArtificial Intelligence (Part - 2) ==================== The Towers of Hanoi is a famous problem for studying recursion incomputer science and searching in artificial intelligence. We start with N discs of varying sizes on a peg (stacked in order according to size), and two empty pegs. We are allowed to move a disc from one peg to another, but we are never allowed to move a larger disc on top of a smaller disc. The goal is to move all the discs to the rightmost peg (see figure). To solve the problem by using search methods, we need first formulate the problem. Supposing there are K pegs and N disk. (2) What is the size of the state space?arrow_forwardEx. 01 : Recursion About So far, we have learned that we can perform repetitive tasks using loops. However, another way is by creating methods that call themselves. This programming technique is called recursion and, a method that calls itself within it's own body is called a recursive method. One use of recursion is to perform repetitive tasks instead of using loops, since some problems seem to be solved more naturally with recursion than with loops. To solve a problem using recursion, it is broken down into sub-problems. Each sub-problem is similar to the original problem, but smaller in size. You can apply the same approach to each sub-problem to solve it recursively. All recursive methods use conditional tests to either 1. stop or 2. continue the recursion. Each recursive method has the following characteristics: 1. end/terminating case: One or more end cases to stop the recursion. 2. recursive case: reduces the problem in to smaller sub-problems, until it reaches (becomes) the end…arrow_forward
- Programming is communication; the programmer “explains” to a computer how to carry out a task, with the explanation being the program. Can you think of any cases where communication directed to people uses direct or indirect recursion? Are there cases where such a use of recursion is indispensable?arrow_forwardIn a recursive algorithm, the goal is to solve a problem by breaking it into smaller sub-problems to solve. This is accomplished by constructing an algorithm using two important components. What are these components, what is the purpose of each?arrow_forwardTask 1 Count the number of vowels in a phrase using recursion only. You can think of this problem as "I can count the number of vowels in this phrase by counting the number of vowels in the first character, then counting the number of vowels in the rest of the phrase." We define a vowel as being A, E, I, O or U. This is one of those problems that can just as easily be solved with traditional programming structures such as a loop - but we're asking you to use recursion for the exercise. Consider: what replaces the loop structure? When will we stop recursion? Task 2 The constant e (Euler's number) is approximated by the following sequence: 1₁ n! where the number of terms in the sequence is sufficient to generate the required precision in decimal places. 1+ 2! + ... We say that there is some value epsilon (e) such that, when the change in the approximation from one term to the next is less than said epsilon, we have reached sufficient precision. That is, when the term you're proposing to…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
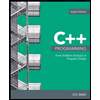
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning